NumPy: numpy.asmatrix() function
numpy.asmatrix() function
The numpy.asmatrix() function is used to interpret the input as a matrix.
Unlike matrix, asmatrix does not make a copy if the input is already a matrix or an ndarray. Equivalent to matrix(data, copy=False).
Syntax:
numpy.asmatrix(data, dtype=None)
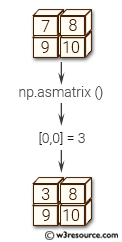
Parameters:
Name | Description | Required / Optional |
---|---|---|
data | Input data. | Required |
dtype | Data-type of the output matrix. | optional |
Return value:
mat : matrix
data interpreted as a matrix.
Example: Converting ndarray to matrix with asmatrix()
>>> import numpy as np
>>> x = np.array([[1,2], [3,4]])
>>> n = np.asmatrix(x)
>>> x[0,0] = 5
>>> n
matrix([[5, 2],
[3, 4]])
The above code demonstrates the use of asmatrix() function to convert an ndarray to a matrix. Initially, an ndarray x with values [[1,2],[3,4]] is created. Using asmatrix() function, x is converted to a matrix n.
The two-dimensional matrix n is a view of the array x. Then the value of x[0,0] is changed to 5. Since both x and n share the same data buffer, the change in value of x is reflected in n. Therefore, n now becomes [[5,2],[3,4]].
Visual Presentation:
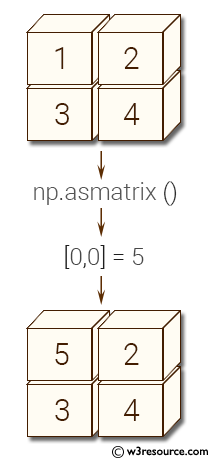
Example-2: NumPy.asmatrix() function
>>> import numpy as np
>>> a = np.array([[2,3], [4,5]])
>>> x = np.asmatrix(a)
>>> a[0,0] = 5
>>> x
matrix([[5, 3],
[4, 5]])
Visual Presentation:
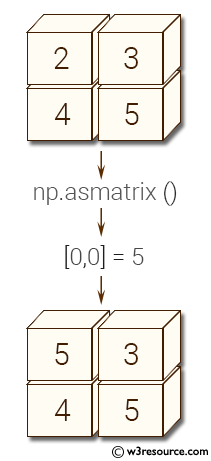
Frequently asked questions (FAQ) - numpy.asmatrix ()
1. What is numpy.asmatrix() used for?
numpy.asmatrix() is used to interpret input as a matrix. It converts input into a matrix.
2. What is the difference between numpy.asmatrix() and numpy.array()?
While both functions can be used to create matrices, numpy.asmatrix() specifically ensures that the input is treated as a matrix, whereas numpy.array() can create arrays of any dimension.
3. How does numpy.asmatrix() handle input types?
It interprets the input as a matrix, regardless of its original type, ensuring it behaves as a matrix object.
4. What are the advantages of using numpy.asmatrix()?
- It provides a convenient way to convert arrays or other sequence-like objects into matrices.
- It maintains matrix properties and operations for the converted input.
5. When should numpy.asmatrix() be used?
Use numpy.asmatrix() when you need to ensure that the input is treated as a matrix, especially when dealing with matrix-specific operations and functions in NumPy.
6. Does numpy.asmatrix() create a copy of the input?
Yes, numpy.asmatrix() creates a new matrix object, potentially with a different memory layout, from the input data.
7. What happens if the input is already a matrix?
If the input is already a matrix, numpy.asmatrix() returns a new matrix object representing the same data.
8. Can numpy.asmatrix() be used to create sparse matrices?
No, numpy.asmatrix() does not support sparse matrices. Use scipy.sparse.asmatrix() for converting sparse matrices to matrix format.
9. Is there any performance overhead associated with numpy.asmatrix()?
Converting input to a matrix using numpy.asmatrix() incurs a slight performance overhead due to the creation of a new matrix object. However, this overhead is typically negligible for small to medium-sized data.
Python - NumPy Code Editor:
Previous: ascontiguousarray()
Next: copy()
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/numpy/array-creation/asmatrix.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics