NumPy: numpy.empty() function
numpy.empty() function
The numpy.empty() function is used to create a new array of given shape and type, without initializing entries. It is typically used for large arrays when performance is critical, and the values will be filled in later.
Syntax:
numpy.empty(shape, dtype=float, order='C')
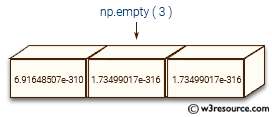
Parameters:
Name | Description | Required / Optional |
---|---|---|
shape | Shape of the empty array, such as (2, 3) or just 2. | Required |
dtype | Desired data type for the array, defaulting to numpy.float64. Examples include numpy.int8, numpy.float32, etc. | Optional |
order | Memory layout order of the array, with 'C' for row-major (C-style) and 'F' for column-major (Fortran-style). | Optional |
Return value:
ndarray: An array of uninitialized (arbitrary) data of the given shape, dtype, and order. For object arrays, entries will be initialized to None.
Example 1: Creating Empty Arrays
Code:
>>> import numpy as np
>>> np.empty(2)
array([ 6.95033087e-310, 1.69970835e-316])
>>> np.empty(32)
array([ 6.95033087e-310, 1.65350412e-316, 6.95032869e-310,
6.95032869e-310, 6.95033051e-310, 6.95033014e-310,
6.95033165e-310, 6.95033167e-310, 6.95033163e-310,
6.95032955e-310, 6.95033162e-310, 6.95033166e-310,
6.95033160e-310, 6.95033163e-310, 6.95033162e-310,
6.95033167e-310, 6.95033167e-310, 6.95033167e-310,
6.95033167e-310, 6.95033158e-310, 6.95033160e-310,
6.95033164e-310, 6.95033162e-310, 6.95033051e-310,
6.95033161e-310, 6.95033051e-310, 6.95033013e-310,
6.95033166e-310, 6.95033161e-310, 2.97403466e+289,
7.55774284e+091, 1.31611495e+294])
>>> np.empty([2, 2])
array([[7.74860419e-304, 8.32155212e-317],
[0.00000000e+000, 0.00000000e+000]])
>>> np.empty([2, 3])
array([[ 6.95033087e-310, 1.68240973e-316, 6.95032825e-310],
[ 6.95032825e-310, 6.95032825e-310, 6.95032825e-310]])
The above code demonstrates the use of np.empty() function in NumPy to create empty arrays of different sizes and data types. The np.empty() function creates an array without initializing its values, which means that the values of the array are undefined and may vary each time the function is called.
In the first example, an empty 1D array of size 2 is created, resulting in an array with two undefined values. The values are shown in the output, which may be different each time the function is called.
In the second example, an empty 1D array of size 32 is created, resulting in an array with 32 undefined values. The values are shown in the output, which may also vary each time the function is called.
In the third example, an empty 2D array of size (2, 2) is created, resulting in an array with four undefined values. The values are shown in the output, which may again vary each time the function is called.
In the fourth example, an empty 2D array of size (2, 3) is created, resulting in an array with six undefined values. The values are shown in the output, which may differ each time the function is called.
Visual Presentation:
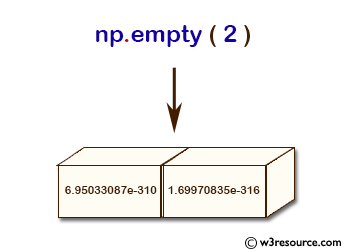
Example 2: Creating Empty Arrays with Specific Data Types
Code:
>>>import numpy as np
>>> np.empty([2, 2], dtype=int)
array([[140144669465496, 16250304],
[140144685653488, 140144685468840]])
>>> np.empty([2, 2], dtype=float)
array([[2.37015306e-316, 7.37071328e-315],
[9.06655519e-317, 2.00753892e-317]])
Explanation: This code demonstrates how to use np.empty() function in NumPy to create empty arrays with specified data types. The dtype parameter in the np.empty() function can be used to specify the data type of the empty array.
In the first example, an empty 2D array of size (2, 2) is created with the specified data type int. The resulting array contains four undefined integer values. The values shown in the output are machine-dependent and may vary each time the function is called.
In the second example, an empty 2D array of size (2, 2) is created with the specified data type float. The resulting array contains four undefined floating-point values. The values shown in the output are also machine-dependent and may vary each time the function is called.
Visual Presentation:
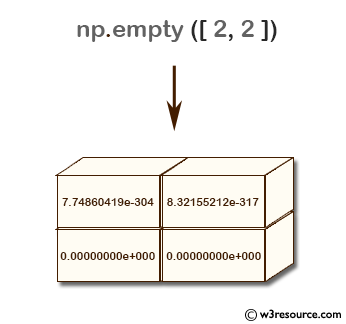
Example 3: Creating an Empty Array with Custom Data Types
Code:
import numpy as np
# Define a custom data type
dt = np.dtype([('Employee Name:', np.str_, 16), ('Age:', np.int32), ('Salary:', np.float64)])
# Create an empty array with the custom data type
employee = np.empty((2, 3), dtype=dt)
# Print the array
print(employee)
Output:
[[('', 0, 0.) ('', 0, 0.) ('', 0, 0.)] [('', 0, 0.) ('', 0, 0.) ('', 0, 0.)]]
In the above example, we first define a custom data type that consists of three fields - Employee Name
(string with length 16), Age (32-bit integer), and Salary (64-bit floating-point number). We then create an empty array with dimensions (2, 3) and data type dt. When we print the array, we see that it contains random values of the custom data type.
Frequently Asked Questions (FAQ): numpy. empty() Function
1. What is the numpy.empty() function used for?
The numpy.empty() function is used to create a new array of a specified shape and data type without initializing the entries.
2. How does numpy.empty() differ from numpy.zeros() or numpy.ones()?
Unlike numpy.zeros() or numpy.ones(), which initialize the array elements to 0 and 1 respectively, numpy.empty() creates an array with uninitialized values, meaning the elements will contain whatever values were previously stored at that memory location.
3. When should we use numpy.empty()?
We should use numpy.empty() when we need to create an array of a specific shape and type but do not require the elements to be initialized to any particular value, which can save time when dealing with large arrays.
4. Is the array created by numpy.empty() safe to use directly?
No, since numpy.empty() does not initialize the array elements, they contain random values. You should only use the array after assigning values to its elements.
5. Does numpy.empty() allocate memory for the array?
Yes, numpy.empty() allocates memory for the array, but it does not initialize the memory with any specific values.
6. Is numpy.empty() faster than functions that initialize array values?
Generally, numpy.empty() is faster than functions like numpy.zeros() or numpy.ones() because it skips the initialization step, making it more efficient for creating large arrays when initialization is not needed.
7. Can numpy.empty() be used with custom data types?
Yes, numpy.empty() can be used to create arrays with custom data types by specifying the desired data type.
8. Does numpy.empty() support multidimensional arrays?
Yes, numpy.empty() can create arrays of any shape, including multidimensional arrays, by specifying the desired dimensions.
Python - NumPy Code Editor:
Previous: NumPy array Home
Next: empty_like()
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/numpy/array-creation/empty.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics