NumPy: numpy.ones_like() function
numpy.ones_like() function
The numpy.ones_like() function is used to get an array of ones with the same shape and type as an existing array.
Syntax:
numpy.ones_like(a, dtype=None, order='K', subok=True)
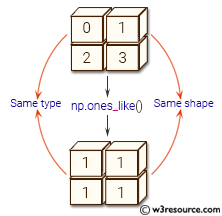
Parameters:
Name | Description | Required / Optional |
---|---|---|
a | The shape and data-type of a define these same attributes of the returned array | Required |
dtype | Overrides the data type of the result. New in version 1.6.0. | optional |
order | Overrides the memory layout of the result. 'C' means C-order, 'F' means F-order, 'A' means 'F' if a is Fortran contiguous, 'C' otherwise. 'K' means match the layout of a as closely as possible. | optional |
subok | If True, then the newly created array will use the sub-class type of ‘a’, otherwise it will be a base-class array. Defaults to True. | optional |
Return value:
[ndarray] Array of ones with the same shape and type as a.
Example: Reshaping and Creating Arrays with Numpy
>>> import numpy as np
>>> x = np.arange(4)
>>> x = x.reshape((2,2))
>>> x
array([[0, 1],
[2, 3]])
>>> x = np.arange(6)
>>> x = x.reshape((2,3))
>>> x
array([[0, 1, 2],
[3, 4, 5]])
>>> np.ones_like(x)
array([[1, 1, 1],
[1, 1, 1]])
In the above code second and third lines create a one-dimensional NumPy array x of length 4, using arange function and then reshapes it to a 2x2 array using reshape.
7th and 8th lines create a one-dimensional NumPy array x of length 6 using arange function and then reshapes it to a 2x3 array using reshape.
The 12th line uses the ones_like function to create an array of ones with the same shape as the input array x.
Pictorial Presentation:
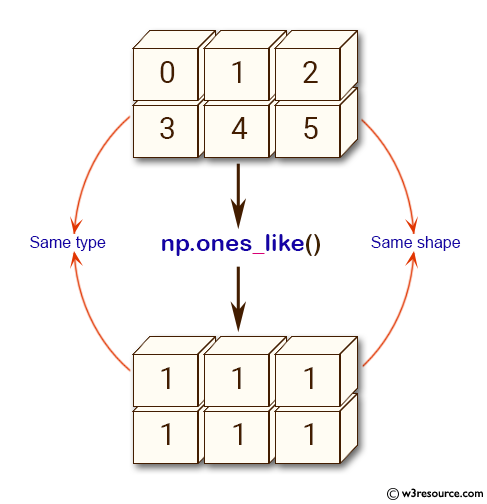
Example: numpy.ones_like where data type is int
>>> import numpy as np
>>> y = np.arange(3, dtype=float)
>>> y
array([ 0., 1., 2.])
>>> np.ones_like(y)
array([ 1., 1., 1.])
>>> a = np.arange(5, dtype=int)
>>> a
array([0, 1, 2, 3, 4])
>>> np.ones_like(a)
array([1, 1, 1, 1, 1])
Pictorial Presentation:
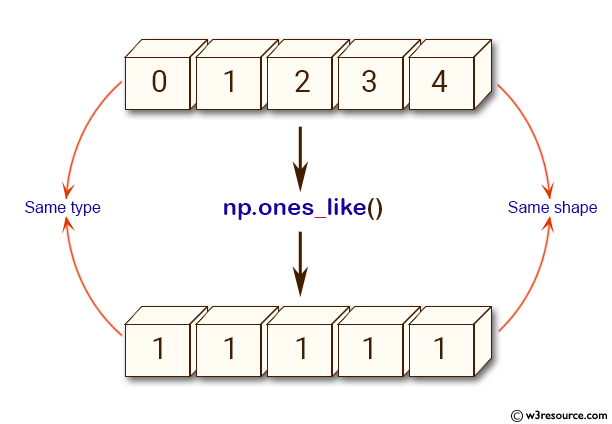
Example: Create an array of ones with the same shape as another array, but with a different data type
Code:
import numpy as np
# Create an array with random values and same shape as arr
print("Array with random values:")
arr = np.random.rand(2, 3, 4)
print(arr)
arr_ones = np.ones_like(arr, dtype=int)
print("\nSame shape as above array, but with a different data type:")
print(arr_ones)
Output:
Array with random values: [[[0.50889494 0.21100556 0.96568893 0.30631918] [0.81124072 0.11792419 0.32569323 0.57254974] [0.48291669 0.05026645 0.07577729 0.64142049]] [[0.82505059 0.9440906 0.88148473 0.04242502] [0.3830825 0.40186863 0.35258408 0.31377575] [0.85416651 0.85453533 0.50357045 0.81113633]]] Same shape as above array, but with a different data type: [[[1 1 1 1] [1 1 1 1] [1 1 1 1]] [[1 1 1 1] [1 1 1 1] [1 1 1 1]]]
In the above example, we create an array with random values and dimensions (2, 3, 4) using np.random.rand() function. We then create an array of ones with the same shape as arr using np.ones_like() function, but with integer data type. The resulting array has the same shape as arr but with ones instead of random values.
Frequently asked questions (FAQ) - numpy. ones_like ()
1. What is numpy.ones_like()?
numpy.ones_like() is a NumPy function that creates a new array of ones with the same shape and type as a given array.
2. When should we use numpy.ones_like()?
Use numpy.ones_like() when you need an array of ones that matches the shape and data type of an existing array. It is particularly useful for initializing arrays for computations that require a matching structure.
3. How does numpy.ones_like() differ from numpy.ones()?
numpy.ones_like() creates an array with the same shape and type as an existing array, whereas numpy.ones() creates an array of a specified shape and data type from scratch.
4. Can numpy.ones_like() handle different data types?
Yes, numpy.ones_like() can handle different data types and will match the data type of the input array unless specified otherwise.
5. Is numpy.ones_like() compatible with multi-dimensional arrays?
Yes, numpy.ones_like() works with arrays of any number of dimensions, creating a new array of ones that mirrors the dimensions of the input array.
6. What happens if the input array is empty?
If the input array is empty, numpy.ones_like() will return an empty array of ones with the same shape and type as the input.
7. Are there any performance considerations?
numpy.ones_like() is optimized for performance, but creating large arrays can still be memory-intensive. Ensure your system has sufficient memory for large array operations.
Python - NumPy Code Editor: