NumPy: numpy.bmat() function
numpy.bmat() function
The numpy.bmat() function is used to create a matrix from a string, nested sequence, or array representation of the matrix. The string representation is given as a nested list of matrices, where each element of the list represents a row of matrices and each matrix in a row is horizontally concatenated. The resulting matrix is the vertical concatenation of these rows of matrices.
Syntax:
numpy.bmat(obj, ldict=None, gdict=None)
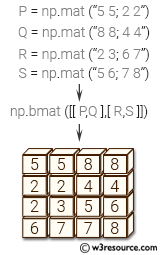
Parameters:
Name | Discription | Required / Optional |
---|---|---|
obj | Input data. If a string, variables in the current scope may be referenced by name. | Required |
ldict | A dictionary that replaces local operands in current frame. Ignored if obj is not a string or gdict is None. | Optional |
gdict | A dictionary that replaces global operands in current frame. Ignored if obj is not a string. | Optional |
Return value:
matrix - Returns a matrix object, which is a specialized 2-D array.
All the following expressions construct the same block matrix:
Example: Concatenating Matrices Horizontally and Vertically using bmat() in NumPy
>>> import numpy as np
>>> P = np.mat('3 3; 4 4')
>>> Q = np.mat('5 5; 5 5')
>>> R = np.mat('3 4; 5 8')
>>> S = np.mat('6 7; 8 9')
>>> np.bmat([[P,Q], [R, S]])
matrix([[3, 3, 5, 5],
[4, 4, 5, 5],
[3, 4, 6, 7],
[5, 8, 8, 9]])
In the above code the matrices P, Q, R, and S are defined using the np.mat() function, which creates a matrix from a string representation. Then, the np.bmat() function is used to concatenate these four matrices into a larger block matrix. The np.bmat() function takes a 2D list of matrices as its argument. In this case, the list is constructed using the square brackets notation and contains two rows and two columns of matrices.
Pictorial Presentation:
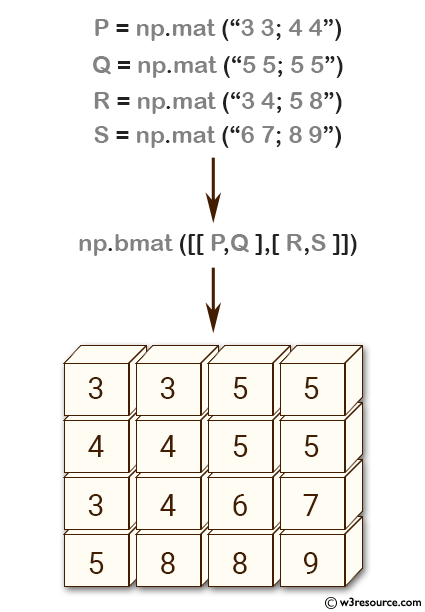
Example: Combining matrices using np.bmat()
>>> import numpy as np
>>> P = np.mat('3 3; 4 4')
>>> Q = np.mat('5 5; 5 5')
>>> R = np.mat('3 4; 5 8')
>>> S = np.mat('6 7; 8 9')
>>> np.bmat(np.r_[np.c_[P, Q], np.c_[R, S]])
matrix([[3, 3, 5, 5],
[4, 4, 5, 5],
[3, 4, 6, 7],
[5, 8, 8, 9]])
>>> np.bmat('P, Q; R, S')
matrix([[3, 3, 5, 5],
[4, 4, 5, 5],
[3, 4, 6, 7],
[5, 8, 8, 9]])
The above code demonstrates how to combine multiple matrices into a single matrix using the np.bmat() function in NumPy. First, four matrices P, Q, R, and S are defined using the np.mat() function. Then, the np.bmat() function is used in two different ways to create a single matrix from these four matrices.
Pictorial Presentation:
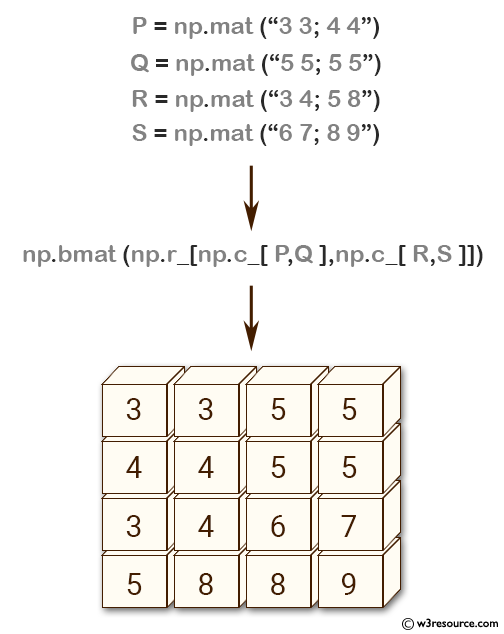
Python - NumPy Code Editor:
Previous: mat()
Next: NumPy Array manipulation Home