NumPy: numpy.full_like() function
numpy.full_like() function
The numpy.full_like() function is used to create an array with the same shape as an existing array and fill it with a specific value.
Syntax:
numpy.full_like(a, fill_value, dtype=None, order='K', subok=True)
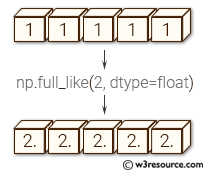
Parameters:
Name | Description | Required / Optional |
---|---|---|
a | The shape and data-type of a define these same attributes of the returned array. | Required |
fill_value | Fill value. | Required |
dtype | Overrides the data type of the result. | optional |
order | Overrides the memory layout of the result. 'C' means C-order, 'F' means F-order, 'A' means 'F' if a is Fortran contiguous, 'C' otherwise. 'K' means match the layout of a as closely as possible. | optional |
subok | If True, then the newly created array will use the sub-class type of 'a', otherwise it will be a base-class array. Defaults to True. | optional |
Return value:
Array of fill_value with the same shape and type as a.
Example: Create arrays of the same shape as another array with np.full_like
>>> import numpy as np
>>> a = np.arange(5, dtype=int)
>>> np.full_like(a, 1)
array([1, 1, 1, 1, 1])
>>> np.full_like(a, 0.1)
array([0, 0, 0, 0, 0])
>>> np.full_like(a, 0.1, dtype=float)
array([ 0.1, 0.1, 0.1, 0.1, 0.1])
>>> np.full_like(a, np.nan, dtype=np.double)
array([ nan, nan, nan, nan, nan])
In the said code the first example fills the array with integer value 1, while the second example fills it with a float value 0.1.
The second example produces an array filled with 0, not 0.1, because the data type of a is integer and the default data type of np.full_like is the same as the input array.
In the third example, dtype is explicitly set to float to get an array with float values. Here, dtype is set to np.double and the value of np.nan is used to create an array with NaN (not a number) values of double precision.
Visual Presentation:
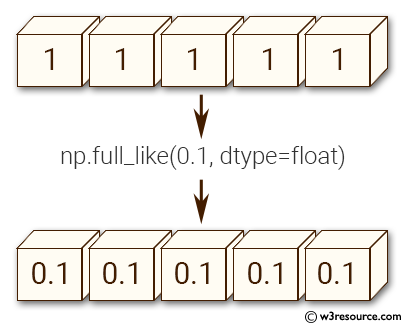
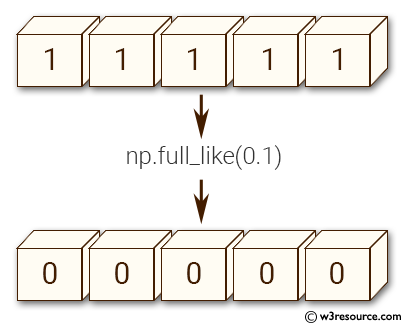
Example: Create a numpy array filled with a constant value using np.full_like()
>>> import numpy as np
>>> b = np.arange(5, dtype=np.double)
>>> np.full_like(b, 0.5)
array([ 0.5, 0.5, 0.5, 0.5, 0.5])
In the above code, an array 'b' is created using the np.arange() function with a data type of 'double'. Then, the np.full_like() function is called with 'b' as the first argument, and a constant value of 0.5 as the second argument. This creates a new numpy array of the same shape and data type as 'b', filled with the constant value 0.5.
Frequently asked questions (FAQ) - numpy. full_like ()
1. What does numpy.full_like() do?
numpy.full_like() creates a new array with the same shape and type as a given array, but with all elements initialized to a specified value.
2. When should I use numpy.full_like()?
Use numpy.full_like() when you need an array of the same shape and type as an existing array, but with all elements set to a specific value.
3. How does numpy.full_like() handle data type?
numpy.full_like() will use the data type of the input array by default, but this can be overridden by specifying a different data type.
4. Can I specify the memory layout for the array created by numpy.full_like()?
Yes, you can specify the desired memory layout (C-order, F-order, etc.) when creating the array.
5. Is numpy.full_like() efficient in terms of memory and performance?
numpy.full_like() is efficient for creating arrays with a specified value, but initializing all elements may take more time compared to numpy.empty_like().
6. What are typical use cases for numpy.full_like()?
Typical use cases include initializing arrays for computations where a specific starting value is required, such as setting initial conditions in simulations.
7. Are there any similar functions to numpy.full_like()?
Similar functions include numpy.zeros_like(), which creates an array of the same shape and type but initializes all elements to zero, and numpy.ones_like(), which initializes all elements to one.
Python - NumPy Code Editor:
Previous: full()
Next: From existing data
array()