NumPy: numpy.fromfunction() function
numpy.fromfunction() function
The numpy.fromfunction() function is used to construct an array by executing a function over each coordinate. The resulting array therefore has a value fn(x, y, z) at coordinate (x, y, z).
The fromfunction() function can be used to create arrays with complex values, as well as to create arrays with values that depend on the index. It is often used to create arrays with a specific mathematical pattern or formula.
Syntax:
numpy.fromfunction(function, shape, **kwargs)
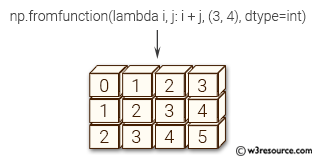
Parameters:
Name | Description | Required / Optional |
---|---|---|
function | The function is called with N parameters, where N is the rank of shape. Each parameter represents the coordinates of the array varying along a specific axis. For example, if shape were (2, 2), then the parameters would be array([[0, 0], [1, 1]]) and array([[0, 1], [0, 1]]) | Required |
shape | Shape of the output array, which also determines the shape of the coordinate arrays passed to function. | Required |
dtype | Data-type of the coordinate arrays passed to function. By default, dtype is float. | Optional |
Return value:
fromfunction : any
The result of the call to function is passed back directly. Therefore the shape of fromfunction is completely determined by function. If function returns a scalar value, the shape of fromfunction would match the shape parameter.
Example: Using numpy.fromfunction() to create a boolean array
>>> import numpy as np
>>> np.fromfunction(lambda i, j: i == j, (2, 3), dtype=int)
array([[ True, False, False],
[False, True, False]], dtype=bool)
The numpy.fromfunction() method is used to construct an array by executing a function over each coordinate of the array.
In the above code, the function is defined using a lambda function that takes in two arguments i and j, which represent the row and column indices of the array, respectively.
The function returns True if the row index i is equal to the column index j, and False otherwise.
The shape of the resulting array is specified as (2, 3), meaning it has 2 rows and 3 columns. The dtype parameter is set to int to create an array of integers.
Finally the resulting array is a boolean array where the element at position (i,j) is True if i=j, and False otherwise.
Visual Presentation:
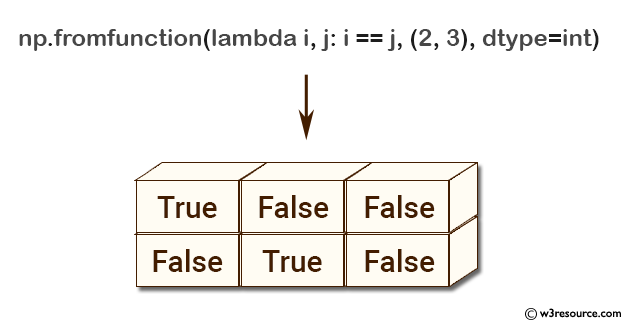
Example: Generating an array using a function with numpy.fromfunction()
>>> import numpy as np
>>> np.fromfunction(lambda i, j: i + j, (2, 3), dtype=int)
array([[0, 1, 2],
[1, 2, 3]])
In the above code, we are using a lambda function that returns the sum of indices i and j as the value for each element of the resulting array. The resulting array has a shape of (2, 3), with two rows and three columns. The elements in the array are computed using the lambda function by passing the row and column indices to it.
The resulting array contains the sum of row and column indices at each position, with values 0, 1, 2, 1, 2, and 3 for each element in the array, respectively. The resulting array has a dtype of int, which is specified using the dtype parameter of the method.
Visual Presentation:
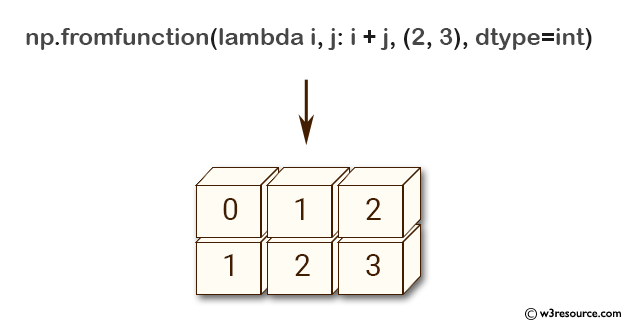
Frequently asked questions (FAQ) - numpy. fromfunction ()
1. What is numpy.fromfunction()?
numpy.fromfunction() is a function in the NumPy library used to construct an array by executing a function over each coordinate of the array.
2. How does numpy.fromfunction() work?
It works by applying a given function to each coordinate of an array, generating an array where each element's value is computed based on its indices.
3. When should we use numpy.fromfunction()?
Use numpy.fromfunction() when you need to generate an array with values that depend on the indices of the array, such as when creating grids or patterns based on coordinate values.
4. Can numpy.fromfunction() create multi-dimensional arrays?
Yes, numpy.fromfunction() can create multi-dimensional arrays by specifying the shape of the desired array and using a function that handles multiple indices.
5. Is numpy.fromfunction() efficient?
numpy.fromfunction() can be efficient for generating arrays based on simple, element-wise calculations. However, for complex operations or very large arrays, performance may vary.
6. How does numpy.fromfunction() handle the array shape?
The shape of the array is defined by the shape parameter provided to numpy.fromfunction(), which determines the dimensions of the generated array.
7. Is there a way to specify the data type of the array created by numpy.fromfunction()?
Yes, the data type of the array can be specified using the dtype parameter.
8. What is the default data type for arrays created with numpy.fromfunction()?
The default data type is float unless otherwise specified.
9. What are some common use cases for numpy.fromfunction()?
Common use cases include generating coordinate grids, creating patterns or mathematical functions over arrays, and initializing arrays with values based on index positions.
Python - Numpy Code Editor:
Previous: copy()
Next: fromiter()