NumPy: Array creation routines
Array creation routines
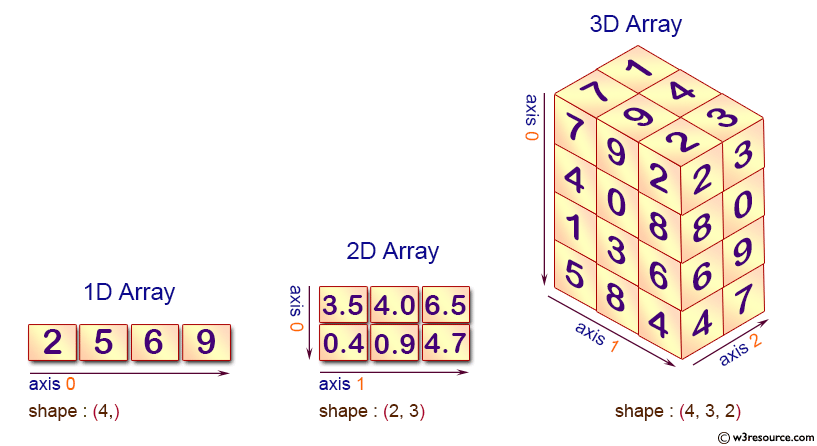
Ones and zeros | ||
Name | Description | Syntax |
---|---|---|
empty() | Return a new array of given shape and type, without initializing entries. | numpy.empty(shape[, dtype, order]) |
empty_like | Return a new array with the same shape and type as a given array. | numpy.empty_like(a[, dtype, order, subok]) |
eye() | Return a 2-D array with ones on the diagonal and zeros elsewhere. | numpy.eye(N[, M, k, dtype]) |
identity() | Return the identity array. | numpy.identity(n[, dtype]) |
ones() | Return a new array of given shape and type, filled with ones. | numpy.ones(shape[, dtype, order]) |
ones_like | Return an array of ones with the same shape and type as a given array. | numpy.ones_like(a[, dtype, order, subok]) |
zeros | Return a new array of given shape and type, filled with zeros. | numpy.zeros(shape[, dtype, order]) |
zeros_like | Return an array of zeros with the same shape and type as a given array. | numpy.zeros_like(a[, dtype, order, subok]) |
full() | Return a new array of given shape and type, filled with fill_value. | numpy.full(shape, fill_value[, dtype, order]) |
full_like() | Return a full array with the same shape and type as a given array. | numpy.full_like(a, fill_value[, dtype, order, subok]) |
Pictorial Presentation: NumPy Array creation
From existing data | ||
Name | Description | Syntax |
---|---|---|
array() | Create an array. | numpy.array(object[, dtype, copy, order, subok, ndmin]) |
asarray() | Convert the input to an array. | numpy.asarray(a[, dtype, order]) |
asanyarray() | Convert the input to an ndarray, but pass ndarray subclasses through. | numpy.asanyarray(a[, dtype, order]) |
ascontiguousarray() | Return a contiguous array in memory (C order). | numpy.ascontiguousarray(a[, dtype]) |
asmatrix() | Interpret the input as a matrix. | numpy.asmatrix(data[, dtype]) |
copy() | Return an array copy of the given object. | numpy.copy(a[, order] |
frombuffer() | Interpret a buffer as a 1-dimensional array. | numpy.frombuffer(buffer[, dtype, count, offset]) |
fromfile() | Construct an array from data in a text or binary file. | numpy.fromfile(file[, dtype, count, sep]) |
fromfunction() | Construct an array by executing a function over each coordinate. | numpy.fromfunction(function, shape, **kwargs) |
fromiter() | Create a new 1-dimensional array from an iterable object. | numpy.fromiter(iterable, dtype[, count]) |
fromstring() | A new 1-D array initialized from raw binary or text data in a string. | numpy.fromstring(string[, dtype, count, sep]) |
loadtxt() | Load data from a text file. | numpy.loadtxt(fname[, dtype, comments, delimiter, ...]) |
Creating record arrays (numpy.rec) |
||
Name | Description | Syntax |
---|---|---|
core.records.array() | Construct a record array from a wide-variety of objects. | core.defchararray.array(obj[, itemsize, ...]) |
core.records.fromarrays() | create a record array from a (flat) list of arrays | core.records.fromarrays(arrayList[, dtype, ...]) |
core.records.fromrecords() | create a recarray from a list of records in text form. | core.records.fromrecords(recList[, dtype, ...]) |
core.records.fromstring() | create a (read-only) record array from binary data contained in a string. | core.records.fromstring(datastring[, dtype, ...]) |
core.records.fromfile() | Create an array from binary file data. | core.records.fromfile(fd[, dtype, shape, ...]) |
Creating character arrays (numpy.char) |
||
Name | Description | Syntax |
---|---|---|
core.defchararray.array() | Create a chararray. | core.defchararray.array(obj[, itemsize, ...]) |
core.defchararray.array() | Convert the input to a chararray, copying the data only if necessary. | core.defchararray.asarray(obj[, itemsize, ...]) |
Numerical ranges | ||
Name | Description | Syntax |
---|---|---|
arange() | Return evenly spaced values within a given interval. | numpy.arange([start,] stop[, step,][, dtype]) |
linspace() | Return evenly spaced numbers over a specified interval. | numpy.linspace(start, stop[, num, endpoint, ...]) |
logspace() | Return numbers spaced evenly on a log scale. | numpy.logspace(start, stop[, num, endpoint, base, ...]) |
geomspace() | Return numbers spaced evenly on a log scale (a geometric progression). | numpy.geomspace(start, stop[, num, endpoint, dtype]) |
meshgrid() | Return coordinate matrices from coordinate vectors. | numpy.meshgrid(*xi, **kwargs) |
mgrid() | nd_grid instance which returns a dense multi-dimensional "meshgrid". | numpy.mgrid |
ogrid() | nd_grid instance which returns an open multi-dimensional "meshgrid". | numpy.ogrid |
Building matrices | ||
Name | Description | Syntax |
---|---|---|
diag() | Extract a diagonal or construct a diagonal array. | numpy.diag(v[, k]) |
diagflat() | Create a two-dimensional array with the flattened input as a diagonal. | numpy.diagflat(v[, k]) |
tri() | An array with ones at and below the given diagonal and zeros elsewhere. | numpy.tri(N[, M, k, dtype]) |
tril() | Lower triangle of an array. | numpy.tril(m[, k]) |
triu() | Upper triangle of an array. | numpy.triu(m[, k]) |
vander() | Generate a Vandermonde matrix. | numpy.vander(x[, N, increasing]) |
The Matrix class | ||
Name | Description | Syntax |
---|---|---|
mat() | Interpret the input as a matrix. | numpy.mat(data[, dtype]) |
bmat() | Build a matrix object from a string, nested sequence, or array. | numpy.bmat(obj[, ldict, gdict]) |
Previous: NumPy ndarray
Next: Ones and Zeros
empty()
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/numpy/array-creation/index.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics