NumPy: numpy.tril() function
numpy.tril() function
The numpy.tril() function is used to get a lower triangle of an array. The function can be useful for working with matrices that have a lower triangular structure, such as when solving systems of linear equations.
Return a copy of an array with elements above the k-th diagonal zeroed.
Syntax:
numpy.tril(m, k=0)
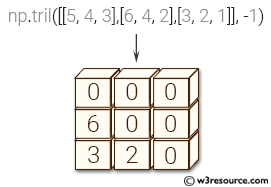
Parameters:
Name | Description | Required / Optional |
---|---|---|
m | Number of rows in the array. | |
k | Diagonal above which to zero elements. k = 0 (the default) is the main diagonal, k < 0 is below it and k > 0 is above. | optional |
Return value:
tril : ndarray, shape (M, N) - Lower triangle of m, of same shape and data-type as m.
Example: Lower triangle of an array using np.tril()
>>> import numpy as np
>>> np.tril([[1,2,3],[4,5,6],[7,8,9]], -1)
array([[0, 0, 0],
[4, 0, 0],
[7, 8, 0]])
In the above code np.tril() takes two arguments: the input array and the index of the lower diagonal (here, -1). The input array is a 3x3 matrix [[1,2,3],[4,5,6],[7,8,9]], and the function returns a 3x3 matrix where all elements above the first subdiagonal are set to zero.
Pictorial Presentation:
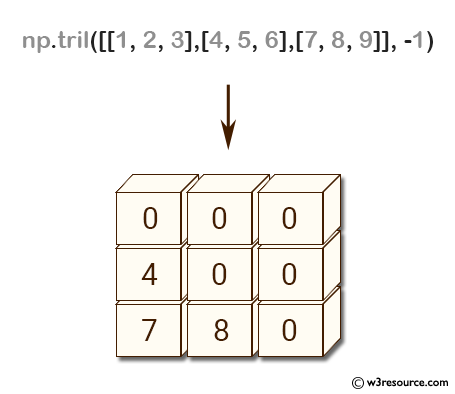
Example: Creating a lower triangular matrix with numpy.tril()
>>> import numpy as np
>>> np.tril([[1,2,3],[4,5,6],[7,8,9],[10,11,12]], -1)
array([[ 0, 0, 0],
[ 4, 0, 0],
[ 7, 8, 0],
[10, 11, 12]])
The above code demonstrates the use of the numpy.tril() function to create a lower triangular matrix with a given array. Here, the function is applied to the 4x3 array [[1,2,3],[4,5,6],[7,8,9],[10,11,12]], and the argument -1 is passed to specify that the matrix should be shifted down by one diagonal.
Pictorial Presentation:
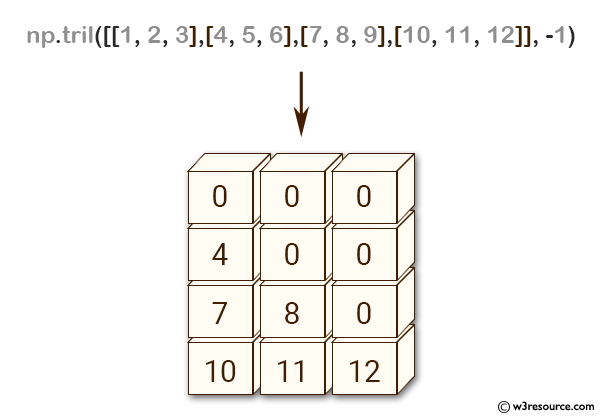
Python - NumPy Code Editor:
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/numpy/array-creation/tril.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics