NumPy String: numpy.char.add() function
numpy.char.add() function
The numpy.char.add() function is used to create element-wise string concatenation for two given arrays of str or unicode.
The two given arrays must have the same shape.
This function can be particularly useful when you need to -
1.
Merging columns of text data in structured arrays or datasets.
2. Prepending or appending text to elements in an array, like adding units to numerical values or creating filenames.
3. Creating combined labels or descriptions from separate arrays of words or phrase
Syntax:
numpy.char.add(x1, x2)
Parameters:
Name | Description | Required / Optional |
---|---|---|
x1: array_like of str or unicode | Input array. | Required |
x2: array_like of str or unicode | Input array | Required |
Return value:
add [ndarray] Output array of string_ or unicode_, depending on input types of the same shape as x1 and x2.
Example: Concatenating elements of two string arrays using NumPy
>>> import numpy as np
>>> x1 = np.array(['p', 't'])
>>> x2 = np.array(['Y', 'H'])
>>> np.char.add(x1, x2)
array(['pY', 'tH'], dtype='<U2')
In the above code the numpy.char.add() function concatenates the corresponding elements of the two input arrays element-wise, resulting in a new array with the same shape.
The output array has the elements 'pY' and 'tH', which are the concatenations of the corresponding elements in x1 and x2. The data type of the output array is '<U2', which represents a Unicode string with a maximum length of 2 characters.
Pictorial Presentation:
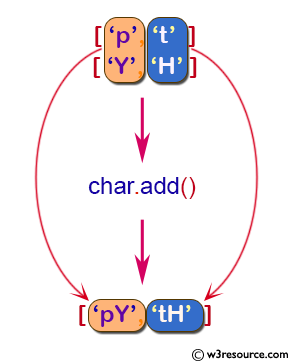
Example: Concatenating Two Strings using NumPy
>> import numpy as np
>>> s1 = np.array(("Java"))
>>> s2 = np.array(("Script"))
>>> np.char.add(s1, s2)
array('JavaScript', dtype='<U10')
In the above code the numpy.char.add() function concatenates the input strings, resulting in a new array containing the concatenated string. The output array contains the single element 'JavaScript', which is the concatenation of the input strings. The data type of the output array is '<U10', which represents a Unicode string with a maximum length of 10 characters.
Pictorial Presentation:
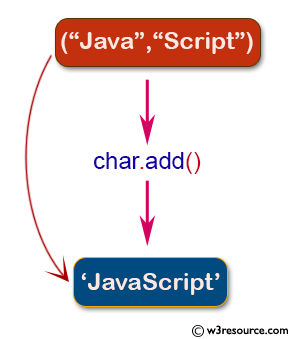
Python - NumPy Code Editor:
Previous:
NumPy String operation Home
Next:
multiply()
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/numpy/string-operations/add.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics