NumPy String: numpy.char.find() function
numpy.char.find() function
The numpy.char.find() function returns the lowest index of the substring in the given string. It returns -1 if the substring is not found.
This function is useful for searching for a substring in a larger string and determining its position.
Syntax:
numpy.char.find(a, sub, start=0, end=None)
Parameters:
Name | Description | Required / Optional |
---|---|---|
a: array_like of str or unicode | Input an array_like of string or unicode. | Required |
sub: str or unicode | Input string or unicode | Required |
start, end: int | Optional arguments start and end are interpreted as in slice notation. | Optional |
Return value:
Returns -1 if sub is not found.
Example: Finding substring using numpy.char.find()
>>> import numpy as np
>>> a = np.char.find('Hello', 'World', start=0, end=None)
>>> a
array(-1)
In the above code, numpy.char.find() function is used to find the starting index of the second string ('World') in the first string ('Hello').
Since the second string is not present in the first string, the output is -1, which means the substring is not found.
Pictorial Presentation:
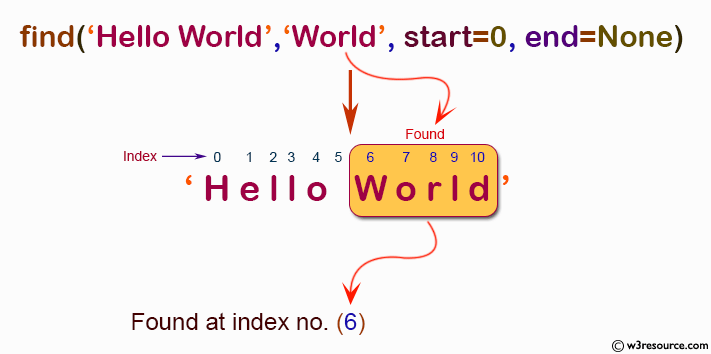
Example: Finding a substring in a string using np.char.find()
>>> import numpy as np
>>> np.char.find('Hello World', 'World', start=0, end=None)
array(6)
>>> np.char.find('Hello World', 'world', start=0, end=None)
array(-1)
In the first example, the function is applied to the string "Hello World" to find the index of the substring "World" within the string. The start parameter is set to 0 and the end parameter is set to None, which means the search is performed on the entire string. Since "World" is present in the string, the function returns an array with value 6, which is the index of the first character of the substring "World" within the string "Hello World".
In the second example, the function is applied to the same string "Hello World" to find the index of the substring "world" within the string. Since "world" is not present in the string, the function returns an array with value -1, indicating that the substring was not found in the string.
Python - NumPy Code Editor: