NumPy String: numpy.char.strip() function
numpy.char.strip() function
The numpy.char.strip() function is used to remove the leading and trailing characters from a given array of strings. The function returns a new array with the stripped strings.
Syntax:
numpy.char.strip(a, chars=None)
Parameters:
Name | Description | Required / Optional |
---|---|---|
a: array-like of str or unicode | Input an array_like of string or unicode. | Required |
chars: str or unicode | The chars argument is a string specifying the set of characters to be removed. If omitted or None, the chars argument defaults to removing whitespace. The chars argument is not a prefix or suffix; rather, all combinations of its values are stripped. | Optional |
Return value:
Output array of str or unicode, depending on input type.
Example: Removing leading and trailing characters with numpy.char.strip()
>>> import numpy as np
>>> x = np.array(['aAaAaA', ' aA ', 'abBABba'])
>>> x
array(['aAaAaA', ' aA ', 'abBABba'], dtype='<U7')
>>> np.char.strip(x)
array(['aAaAaA', 'aA', 'abBABba'], dtype='<U7')
>>> np.char.strip(x, 'a')
array(['AaAaA', ' aA ', 'bBABb'], dtype='<U7')
>>> np.char.strip(x, 'A')
array(['aAaAa', ' aA ', 'abBABba'], dtype='<U7')
In the above example -
- The first example np.char.strip() removes any leading or trailing white spaces from each string in the array.
- The second example, np.char.strip() removes the character 'a' from the beginning and end of each string.
- The third example np.char.strip() removes the character 'A' from the beginning and end of each string.
Example: Removing specific characters from a string using numpy.char.strip()
>>> import numpy as np
>>> a = np.char.strip('apple basket', 'a')
>>> a
array('pple basket', dtype='<U12')
In the above example, numpy.char.strip() function is used to remove character 'a' from the string 'apple basket'. The function returns a new string with the specified characters removed.
Pictorial Presentation:
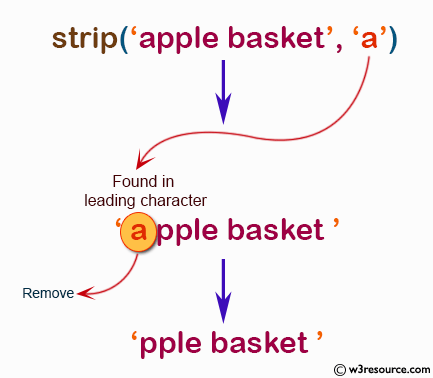
Example: Removing specified character from the beginning and end of a string using numpy.char.strip()
>>> import numpy as np
>>> a = np.char.strip('basket ball', 'l')
>>> a
array('basket ba', dtype='<U11')
In the above example numpy.char.strip() function is used to remove the characters 'l' from the end of the string 'basket ball'. The resulting array contains the modified string 'basket ba'.
Pictorial Presentation:
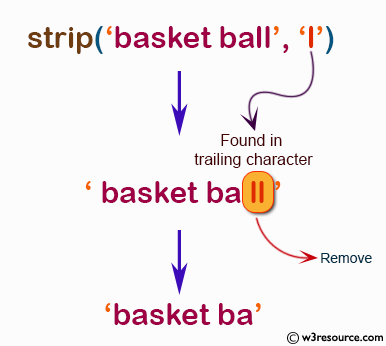
Python - NumPy Code Editor:
Previous:
splitlines()
Next:
swapcase()