NumPy String: numpy.char.rpartition() function
numpy.char.rpartition() function
The numpy.char.rpartition() function is used to split the elements of an array of strings into three parts using a specified separator from the right end of the string.
For each element in 'a', split the element as the last occurrence of 'sep', and return 3 strings containing the part before the separator, the separator itself, and the part after the separator. If the separator is not found, return 3 strings containing the string itself, followed by two empty strings.
For example, if the separator is '.' and the string is 'www.w3resource.com', the resulting three parts will be 'www.w3resource, '.', and 'com'.
This function is useful when working with datasets containing string values that need to be split and analyzed further. It is commonly used in natural language processing (NLP) applications, such as text cleaning and data preprocessing.
Syntax:
numpy.char.rpartition(a, sep)
Parameters:
Name | Description | Required / Optional |
---|---|---|
a: array-like of str or unicode | Input array | Required |
sep: int or unicode | Right-most separator to split each element in array. | Required |
Return value:
Output array of string or unicode, depending on input type. The output array will have an extra dimension with 3 elements per input element.
Example: Splitting a string with numpy.char.rpartition()
import numpy as np
x = "Python Exercises, Practice, Solution"
print("Original string:")
print(x)
print("\nHere separator is 'Practice'")
print(np.char.rpartition(x, 'Practice'))
print("\nHere separator is 'Solution'")
print(np.char.rpartition(x, 'Solution'))
print("\nHere separator is ','")
print(np.char.rpartition(x, ','))
Output:
Original string: Python Exercises, Practice, Solution Here separator is 'Practice' ['Python Exercises, ' 'Practice' ', Solution'] Here separator is 'Solution' ['Python Exercises, Practice, ' 'Solution' ''] Here separator is ',' ['Python Exercises, Practice' ',' ' Solution']
In the above code -
- In the first example the separator is set to "Practice". It returns a NumPy array containing the left part of the string before the separator, the separator itself, and the right part of the string after the separator. In this case, the left part is "Python Exercises,", the separator is "Practice", and the right part is " Solution".
- In the second example the separator is set to "Solution". It returns a NumPy array containing the left part of the string before the separator, the separator itself, and the right part of the string after the separator. In this case, the left part is "Python Exercises, Practice,", the separator is "Solution", and the right part is an empty string.
- In the third example the separator is set to ",". It returns a NumPy array containing the left part of the string before the separator, the separator itself, and the right part of the string after the separator. In this case, the left part is "Python Exercises", the separator is ",", and the right part is " Practice, Solution"
Pictorial Presentation:
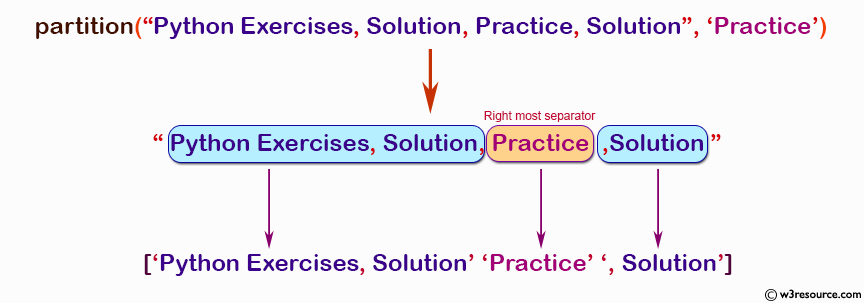
Example: Using numpy.char.rpartition() to split a string into three parts based on a separator
import numpy as np
x = "Python Exercises, Solution, Practice, Solution"
print("Original string:")
print(x)
print("\nHere separator is 'Solution'")
print(np.char.rpartition(x, 'Solution'))
Output:
Original string: Python Exercises, Solution, Practice, Solution Here separator is 'Solution' ['Python Exercises, Solution, Practice, ' 'Solution' '']
In the above example the rpartition() function searches the string from right to left for the first occurrence of the separator 'Solution'. It returns a NumPy array of three elements consisting of the part of the string before the separator, the separator itself, and the part of the string after the separator.
Pictorial Presentation:
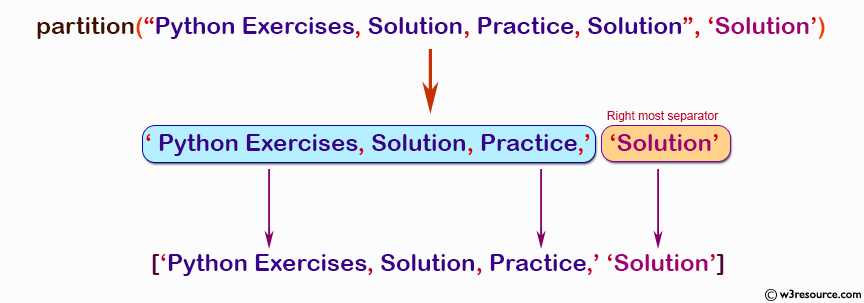
Python - NumPy Code Editor: