NumPy String: numpy.char.replace() function
numpy.char.replace() function
The numpy.char.partition() function is used to partition the elements of a string array or a single string around a specified separator (substring).
This function is useful in -
- Text processing: In natural language processing or text analysis tasks, we may need to split string arrays into parts based on a specific separator for further analysis, manipulation, or processing.
- Data preprocessing: When working with data containing string arrays, we may need to partition the elements based on a specific separator to extract meaningful information or to restructure the data for further analysis.
- Data cleaning: In some cases, we may need to correct inconsistencies within a dataset by partitioning string elements around a specific separator and extracting relevant information.
Syntax:
numpy.char.replace(a, old, new, count=None)
Parameters:
Name | Description | Required / Optional |
---|---|---|
a | Given array-like of string or unicode | Required |
old, new | Given old or new string or unicode | Required |
count | If the optional argument count is given, only the first count occurrences are replaced. | Optional |
Return value:
Output array of str or unicode, depending on input type.
Example: Replacing substring using NumPy char.replace()
>>> import numpy as np
>>> x = np.char.replace('The quick brown fox', 'fox', 'wolf')
>>> x
array('The quick brown wolf', dtype='<U20')
In the above code example, the numpy.char.replace() function is used to replace a specified substring within a single string. Here the function replaced the occurrence of the search substring 'fox' with the substring 'wolf'.
Pictorial Presentation:
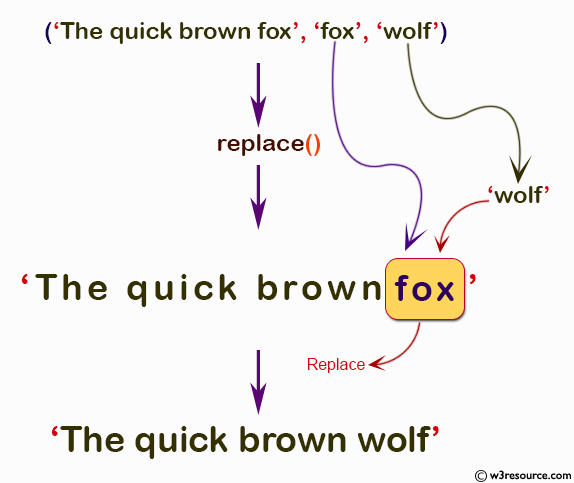
Example: No substring replacement with NumPy char.replace() using count=0
>>> import numpy as np
>>> x = np.char.replace('The quick fox brown fox', 'fox', 'wolf', count=0)
>>> x
array('The quick fox brown fox', dtype='<U23')
The numpy.char.replace() function is called with the input string 'The quick fox brown fox', the search substring 'fox', the replace substring 'wolf', and the count parameter set to 0. Since the count is 0, no replacements will be made.
The result is an array containing the original string with a dtype of '<U23'.
Pictorial Presentation:
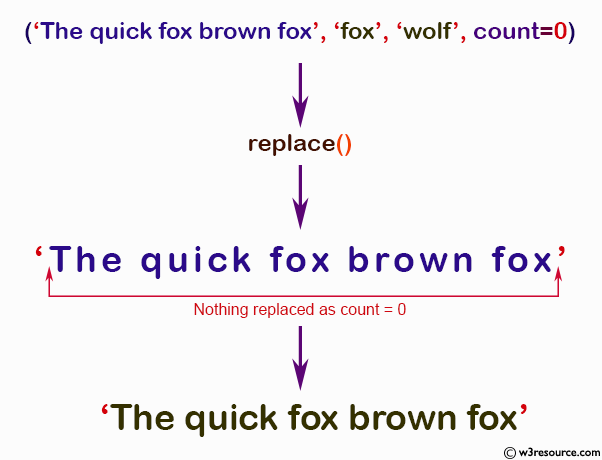
Example: Replacing substring once using NumPy char.replace() with count=1
>>> import numpy as np
>>> x = np.char.replace('The quick fox brown fox', 'fox', 'wolf', count=1)
>>> x
array('The quick wolf brown fox', dtype='<U24')
This example shows how the count parameter in numpy.char.replace() can be used to control the number of replacements made within a string. In this case, setting count to 1 results in only the first occurrence of the search substring being replaced.
Pictorial Presentation:
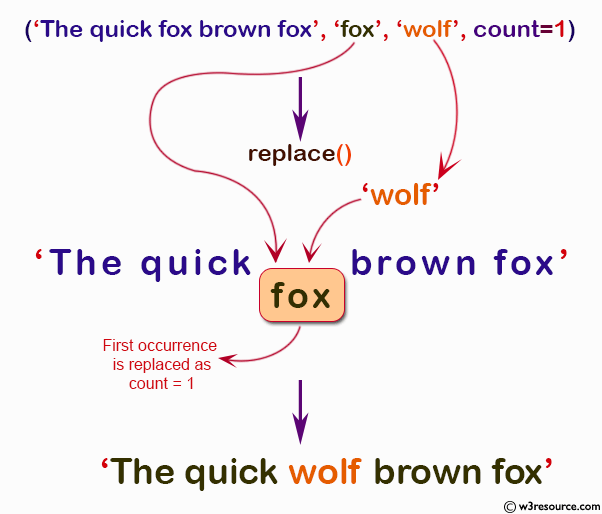
Example: Replacing substring multiple times using NumPy char.replace() with count=2
>>> import numpy as np
>>> x = np.char.replace('The quick fox brown fox', 'fox', 'wolf', count=2)
>>> x
array('The quick wolf brown wolf', dtype='<U25')
This above code shows how the count parameter in numpy.char.replace() can be used to control the number of replacements made within a string. In this case, setting count to 2 results in both occurrences of the search substring being replaced.
Pictorial Presentation:
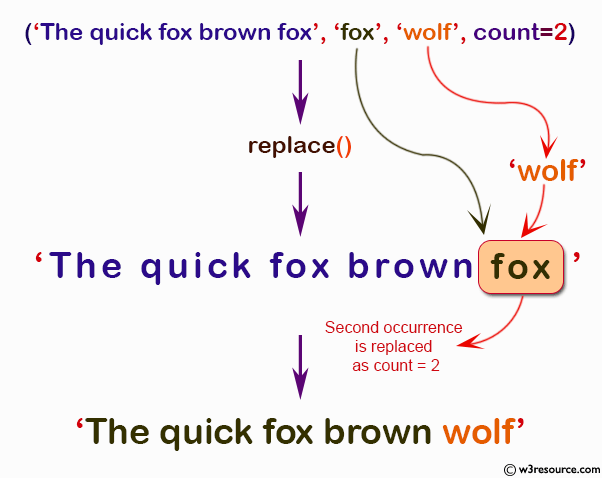
Python - NumPy Code Editor:
Previous:
partition()
Next:
rjust()