NumPy String: numpy.char.capitalize() function
numpy.char.capitalize() function
The numpy.char.capitalize() function is used to create a copy of a given array with only the first character of each element capitalized.
This function is useful in
- Text preprocessing: In natural language processing or text analysis tasks, it may be necessary to standardize the capitalization of words or phrases in a dataset.
- Formatting output: When presenting text data, such as in reports or visualizations, you may want to capitalize the first character of each element in a string array to improve readability or follow a specific style guide.
Syntax:
numpy.char.capitalize(a)
Parameter:
Name | Description | Required / Optional |
---|---|---|
a: array_like of str or unicode | Input array of strings to capitalize. | Required |
Return value:
out : ndarray - Output array of str or unicode, depending on input types.
Example: Capitalizing first characters of string elements in a NumPy array
>>> import numpy as np
>>> x = np.array(['a1b2','1b2a','b2a1','2a1b']);
>>> np.char.capitalize(x)
array(['A1b2', '1b2a', 'B2a1', '2a1b'],
dtype='<U4')
In the above code the numpy.char.capitalize() function is called with the input array x. This function capitalizes the first character of each element in the array and returns a new array with the same shape. The output array contains the elements 'A1b2', '1b2a', 'B2a1', and '2a1b', with the first characters capitalized and the remaining characters unchanged.
Pictorial Presentation:
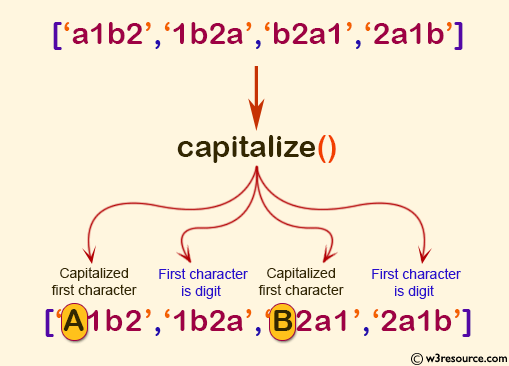
Example: Capitalizing first characters of strings using NumPy's char.capitalize()
import numpy as np
a1 = 'python tutorial'
a2 = 'python exercises'
print ("a1: ", a1)
print ("a2: ", a2)
print("\nArray data after using capitalize():")
print ("a1: ", np.char.capitalize(a1))
print ("a2: ", np.char.capitalize(a2))
Output:
a1: python tutorial a2: python exercises Array data after using capitalize(): a1: Python tutorial a2: Python exercises
In the above code the numpy.char.capitalize() function is called separately for each of the input strings 'a1' and 'a2'. This function capitalizes the first character of each string while converting the remaining characters to lowercase, and returns new 0-dimensional arrays (scalars) containing the capitalized strings.
Pictorial Presentation:
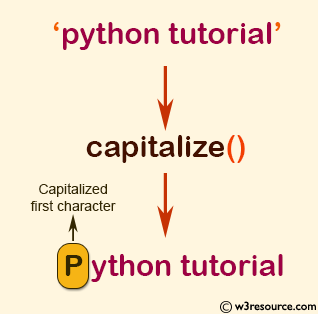
Python - NumPy Code Editor: