NumPy String: numpy.char.splitlines() function
numpy.char.splitlines() function
For each element in a given array numpy.char.splitlines() function returns a list of the lines in the element, breaking at line boundaries.
Syntax:
numpy.char.splitlines(a, keepends=None)
Parameters:
Name | Description | Required / Optional |
---|---|---|
a: array-like of str or unicode | Input an array-like of string or unicode. | Required |
keepends: bool | Line breaks are not included in the resulting list unless keepends is given and true. | Optional |
Return value:
out [ndarray] Array of list objects.
Example: Splitting a String into Lines using numpy.char.splitlines()
>>> import numpy as np
>>> x = np.char.splitlines('The quick \nbrown fox jumps \nover the lazy dog.')
>>> x
array(list(['The quick ', 'brown fox jumps ', 'over the lazy dog.']),
dtype=object)
In the above example numpy.char.splitlines() function is used to split a string or an array of strings into substrings at line breaks.
The returned array contains three strings: 'The quick ', 'brown fox jumps ', and 'over the lazy dog.' Each of these strings is a substring of the original string that was separated by line breaks. The dtype=object parameter specifies the type of elements in the returned array.
Pictorial Presentation:
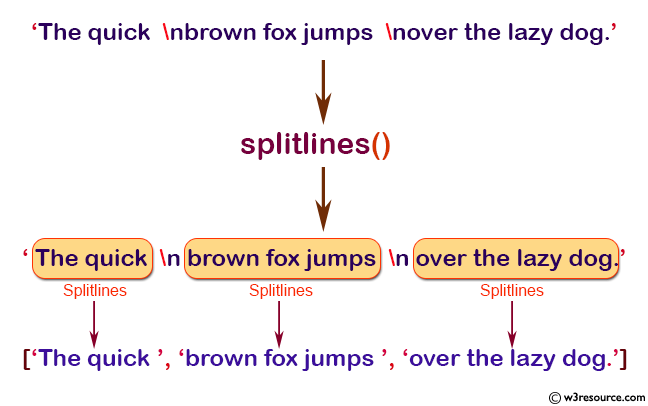
Example: Splitting lines from a string using numpy.char.splitlines()
>>> import numpy as np
>>> x = np.char.splitlines('The quick brown \rfox jumps over \rthe lazy dog.', keepends=None)
>>> x
array(list(['The quick brown ', 'fox jumps over ', 'the lazy dog.']),
dtype=object)
Note: \n or \r is used for breaking at line boundaries.
This code uses the numpy.char.splitlines() function to split a string into substrings based on newline characters. the input string contains newline characters as well as carriage return characters. The optional parameter 'keepends' is set to None, which means that the newline or carriage return characters are not included in the output substrings.
Python - NumPy Code Editor: