MySQL TIMESTAMPDIFF() function
TIMESTAMPDIFF() function
The MySQL TIMESTAMPDIFF() function is a powerful tool that calculates the difference between two date or datetime values, returning the result in the specified unit of time. This function is crucial for various time-based analyses, enabling users to compute the duration between events or timestamps accurately. The TIMESTAMPDIFF() function can handle inputs where one value is a date and the other is a datetime, treating date values as datetime values at '00:00:00'.
This function is useful in -
- TIMESTAMPDIFF() is essential for time-based analysis by enabling you to measure the duration between events or actions.
- It's useful for calculating ages or durations, such as the age of a person based on their birthdate.
- TIMESTAMPDIFF() is used in time series analysis to calculate the time intervals between data points.
- The function is valuable for filtering records or data based on time criteria, such as selecting records within a specific time range.
- TIMESTAMPDIFF() supports the analysis of historical data by providing precise measurements of time intervals.
- It provides a precise measurement of the time difference between two points in time, allowing for accurate calculations.
- The function allows you to calculate time differences in units other than seconds, such as years, months, or days.
Syntax:
TIMESTAMPDIFF(unit,datetime_expr1,datetime_expr2);
Arguments:
Name | Description |
---|---|
datetime_expr1 | The first datetime expression from which the second datetime expression is subtracted. |
datetime_expr1 | The second datetime expression to be subtracted from the first. |
unit | Specifies the unit of the result. Accepted values include:
|
Syntax Diagram:

MySQL Version: 8.0
Pictorial Presentation:
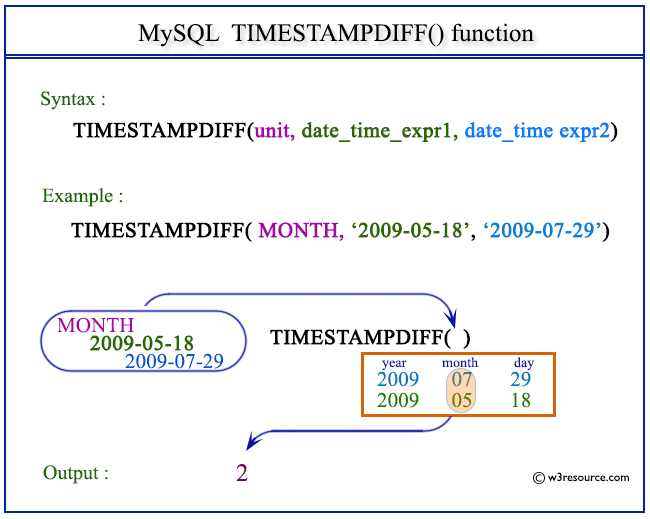
Example: Calculate Months Difference:
The following query returns the number of months between May 18, 2009, and July 29, 2009, which is 2.
Code:
SELECT TIMESTAMPDIFF(MONTH,'2009-05-18','2009-07-29');
-- Selects the difference in months between two dates.
SELECT TIMESTAMPDIFF(MONTH, -- Specifies that the result should be in months.
'2009-05-18', -- The first date (start date).
'2009-07-29' -- The second date (end date).
) AS MonthsDifference -- Aliases the result column as "MonthsDifference" for clarity.
FROM DUAL; -- DUAL is a special dummy table used in MySQL to perform calculations or retrieve constants.
Explanation:
- This SQL query calculates the number of months between the two specified dates: May 18, 2009, and July 29, 2009.
- It uses the TIMESTAMPDIFF() function with MONTH as the unit of difference.
- The function returns the integer difference in months between these two dates.
- The result, which is 2 months, is labeled as "MonthsDifference" in the output.
Output:
mysql> SELECT TIMESTAMPDIFF(MONTH,'2009-05-18','2009-07-29'); +------------------------------------------------+ | TIMESTAMPDIFF(MONTH,'2009-05-18','2009-07-29') | +------------------------------------------------+ | 2 | +------------------------------------------------+ 1 row in set (0.00 sec)
Example: TIMESTAMPDIFF() function in MINUTE
The following query computes the number of minutes between two datetime values, after subtracting 2009-05-18 11:45:42 from 2009-05-20 15:16:39, resulting in 3090 minutes.
Code:
-- Selects the difference in minutes between two datetime values.
SELECT TIMESTAMPDIFF(MINUTE, -- Specifies that the result should be in minutes.
'2009-05-18 11:45:42', -- The first datetime (start datetime).
'2009-05-20 15:16:39' -- The second datetime (end datetime).
) AS MinutesDifference -- Aliases the result column as "MinutesDifference" for clarity.
FROM DUAL; -- DUAL is a special dummy table used in MySQL to perform calculations or retrieve constants.
Explanation:
- This SQL query calculates the difference in minutes between two specified datetime values: May 18, 2009, at 11:45:42 and May 20, 2009, at 15:16:39.
- Using the TIMESTAMPDIFF() function with MINUTE as the unit, it determines the total number of minutes between these two timestamps.
- The result, which is 3090 minutes, is labeled as "MinutesDifference" in the output.
Output:
mysql> SELECT TIMESTAMPDIFF(MINUTE,'2009-05-18 11:45:42','2009-05-20 15:16:39'); +-------------------------------------------------------------------+ | TIMESTAMPDIFF(MINUTE,'2009-05-18 11:45:42','2009-05-20 15:16:39') | +-------------------------------------------------------------------+ | 3090 | +-------------------------------------------------------------------+ 1 row in set (0.00 sec)
Usage Scenarios of TIMESTAMPDIFF:
Age Calculation:
Code:
-- Calculates the difference in years between two dates.
SELECT TIMESTAMPDIFF(YEAR, -- Specifies that the result should be in years.
'1990-06-15', -- The start date (earlier date).
CURDATE() -- The current date provided by the MySQL function CURDATE().
) AS Age -- Aliases the result column as "Age" for clarity.
FROM DUAL; -- DUAL is a special dummy table used in MySQL to perform calculations or retrieve constants.
Explanation:
- This SQL query calculates the age in years from a given birthdate ('1990-06-15') to the current date.
- The TIMESTAMPDIFF() function is used with YEAR as the unit to measure the difference between the specified birthdate and the current date, which is fetched dynamically using the CURDATE() function.
- The result is displayed under the column "Age", representing the number of full years between the two dates.
Session Duration Analysis:
Code:
-- Calculates the duration of a user's session in minutes.
SELECT TIMESTAMPDIFF(MINUTE, -- Specifies that the result should be in minutes.
login_time, -- The starting timestamp of the session.
logout_time -- The ending timestamp of the session.
) AS SessionDuration -- Aliases the result column as "SessionDuration" for clarity.
FROM user_sessions -- The table containing user session records.
WHERE user_id = 12345; -- Filters the records to only include the session(s) of the user with ID 12345.
Explanation:
- This SQL query calculates the duration of a user's session in minutes by subtracting the login_time from the logout_time for a specified user.
- The TIMESTAMPDIFF() function is used with MINUTE as the unit to compute the time difference in minutes.
- The query filters the data to only include sessions for the user with the user_id 12345.
- The resulting duration is displayed under the column "SessionDuration".
Time Series Analysis:
Code:
-- Retrieves the event ID and calculates the number of days between two event dates.
SELECT event_id, -- Selects the event's unique identifier.
TIMESTAMPDIFF(DAY, -- Specifies that the result should be in days.
previous_event_date, -- The earlier event date.
current_event_date -- The later event date.
) AS DaysBetween -- Aliases the result column as "DaysBetween" for clarity.
FROM events -- The table containing event records.
WHERE event_type = 'purchase'; -- Filters the records to only include events of type 'purchase'.
Explanation:
- This SQL query calculates the number of days between two event dates (previous_event_date and current_event_date) for events categorized as 'purchase'.
- TIMESTAMPDIFF(DAY, previous_event_date, current_event_date): This function calculates the difference in days between the two specified dates.
- event_id: This is the unique identifier for each event.
- FROM events: Indicates that the data is being pulled from the events table.
- WHERE event_type = 'purchase': Filters the results to only include rows where the event type is 'purchase'.
Data Filtering:
Code:
-- Selects all columns from the 'transactions' table.
SELECT *
FROM transactions -- Specifies the 'transactions' table to query.
WHERE TIMESTAMPDIFF(HOUR, -- Calculates the difference in hours between:
transaction_time, -- The timestamp of each transaction.
NOW() -- The current date and time.
) <= 24; -- Filters for transactions that occurred within the last 24 hours.
Explanation:
- This SQL query retrieves all rows from the transactions table where the transactions happened within the last 24 hours.
- SELECT *: Selects all columns from the transactions table.
- FROM transactions: Indicates that the data is being pulled from the transactions table.
- TIMESTAMPDIFF(HOUR, transaction_time, NOW()): This function calculates the difference in hours between the transaction_time and the current time (NOW()).
- WHERE ... <= 24: The query filters the results to include only those transactions that occurred in the last 24 hours (i.e., the difference between the transaction_time and the current time is 24 hours or less).
Historical Data Analysis:
Code:
-- Calculates the number of months between two dates.
SELECT TIMESTAMPDIFF(MONTH, -- Uses the TIMESTAMPDIFF function to calculate the difference in months.
'2021-01-01', -- The starting date for the calculation (January 1, 2021).
'2024-01-01' -- The ending date for the calculation (January 1, 2024).
) AS MonthsPassed; -- Aliases the result as "MonthsPassed" for clarity in the output.
Explanation:
- This SQL query computes the number of months that have passed between January 1, 2021, and January 1, 2024.
- TIMESTAMPDIFF(MONTH, '2021-01-01', '2024-01-01'): The TIMESTAMPDIFF function is used to determine the difference between two dates in terms of months.
- MONTH: Specifies that the difference should be calculated in months.
- '2021-01-01': The starting date of the interval.
- '2024-01-01': The ending date of the interval.
- AS MonthsPassed: The result of the TIMESTAMPDIFF function is labeled as "MonthsPassed".
Best Practices and Optimization Tips
- Index Usage: Ensure your datetime columns are indexed to optimize query performance when using TIMESTAMPDIFF() in filters or joins.
- Consistent Data Types: When possible, use the same data type for both datetime expressions to avoid implicit conversions.
- Time Zones: Be mindful of time zones when comparing datetime values across different zones to ensure accuracy in your results.
- Unit Selection: Choose the appropriate unit for your calculation to avoid unnecessary conversions and improve clarity in results.
Related Functions:
-- Calculates the number of days between two dates.
SELECT DATEDIFF('2024-01-01', -- The ending date for the calculation (January 1, 2024).
'2023-01-01') -- The starting date for the calculation (January 1, 2023).
AS DaysDifference; -- Aliases the result as "DaysDifference" for clarity in the output.
Explanation:
This SQL query calculates the number of days between January 1, 2023, and January 1, 2024
- DATEDIFF('2024-01-01', '2023-01-01'): The DATEDIFF function computes the difference in days between two dates.
- '2024-01-01': The ending date for the calculation.
- '2023-01-01': The starting date for the calculation.
- AS DaysDifference: The result of the DATEDIFF function is labeled as "DaysDifference".
-- Adds a specified number of days to a given date and returns the resulting new date.
SELECT TIMESTAMPADD(DAY, -- The unit of time to add; in this case, it's days.
10, -- The number of days to add to the starting date.
'2023-01-01') -- The starting date to which the days will be added (January 1, 2023).
AS NewDate; -- Aliases the result as "NewDate" for clarity in the output.
Explanation:
This SQL query uses the TIMESTAMPADD function to add 10 days to the date January 1, 2023.
- TIMESTAMPADD(DAY, 10, '2023-01-01'):
- DAY: The unit of time for the addition (days).
- 10: The number of days to add.
- '2023-01-01': The initial date to which the days will be added.
- AS NewDate: The result of the TIMESTAMPADD function is labeled as "NewDate".
-- Subtracts one year from the specified date '2024-01-01' and returns the resulting date.
SELECT DATE_SUB('2024-01-01', -- The starting date from which the interval will be subtracted.
INTERVAL 1 YEAR) -- Specifies the interval to subtract; in this case, it's 1 year.
AS SubtractedDate; -- Aliases the result as "SubtractedDate" for clarity in the output.
Explanation:
This SQL query uses the DATE_SUB function to subtract one year from the date January 1, 2024.
- DATE_SUB('2024-01-01', INTERVAL 1 YEAR):
- '2024-01-01': The initial date from which the interval will be subtracted.
- INTERVAL 1 YEAR: Specifies that one year should be subtracted from the starting date.
- AS SubtractedDate: The result of the DATE_SUB function is labeled as "SubtractedDate".
-- Adds 2 months to the specified date '2023-01-01' and returns the resulting date.
SELECT DATE_ADD('2023-01-01', -- The starting date to which the interval will be added.
INTERVAL 2 MONTH) -- Specifies the interval to add; in this case, it's 2 months.
AS AddedDate; -- Aliases the result as "AddedDate" for clarity in the output.
Explanation:
This SQL query uses the DATE_ADD function to add 2 months to the date January 1, 2023.
- DATE_ADD('2023-01-01', INTERVAL 2 MONTH):
- '2023-01-01': The initial date to which the interval will be added.
- INTERVAL 2 MONTH: Specifies that two months should be added to the starting date.
- AS AddedDate: The result of the DATE_ADD function is labeled as "AddedDate".
Common Errors:
- Incorrect Unit Selection: Using the wrong unit can lead to misleading results. Always verify the unit aligns with the context of your calculation.
- Mixed Time Zones: Differences between datetime values in different time zones may not be accurate without proper time zone handling.
- Handling NULL Values: Ensure that neither datetime expression is NULL to avoid unexpected results or errors.
Frequently Asked Questions (FAQ) - MySQL TIMESTAMPDIFF() Function
1. What is the MySQL TIMESTAMPDIFF() function used for?
The TIMESTAMPDIFF() function in MySQL calculates the difference between two datetime or date values, returning the result in specified units such as seconds, minutes, hours, days, weeks, months, quarters, or years.
2. Where is TIMESTAMPDIFF() function commonly used?
The function is essential for time-based analysis, allowing users to measure durations between events, calculate ages or durations, analyze time series data, filter records based on time criteria, and perform historical data analysis with precise time measurements.
3. Can TIMESTAMPDIFF() handle different datetime types?
Yes, TIMESTAMPDIFF() can process inputs where one value is a date and the other is a datetime. Date values are treated as datetime values with the time part set to '00:00:00'.
4. What are the supported units in TIMESTAMPDIFF()?
Supported units include FRAC_SECOND (microseconds), SECOND, MINUTE, HOUR, DAY, WEEK, MONTH, QUARTER, and YEAR. These units define the granularity of the time difference calculation.
5. Is TIMESTAMPDIFF() compatible with all MySQL versions?
Yes, TIMESTAMPDIFF() is supported across all versions of MySQL, including MySQL 8.0.
6. What are some best practices for using TIMESTAMPDIFF()?
Best practices include ensuring datetime columns are indexed for performance optimization, maintaining consistent data types to avoid implicit conversions, considering time zones for accurate comparisons, and selecting appropriate units to enhance result clarity.
Video Presentation:
All Date and Time Functions:
Click here to see the MySQL Date and time functions.
Previous: TIMESTAMPADD()
Next: TO_DAYS()