NumPy: numpy.atleast_2d() function
numpy.atleast_2d() function
The numpy.atleast_2d() is used to ensure given inputs as arrays with at least two dimensions. If the input is already a 2D or higher-dimensional array, it is returned unchanged. However, if the input is a 1D array, a new 2D array is created by adding an additional axis.
This function is useful when an input array is required to have at least two dimensions, such as when performing matrix operations.
Syntax:
numpy.atleast_2d(*arys)
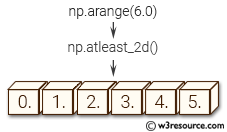
Parameters:
Name | Description | Required / Optional |
---|---|---|
arys1, arys2, . . . | One or more array-like sequences. Non-array inputs are converted to arrays. Arrays that already have two or more dimensions are preserved. | Required |
Return value:
res, res2, . . . [ndarray]
An array, or list of arrays, each with a.ndim >= 2. Copies are
avoided where possible, and views with two or more dimensions are returned.
Example: Creating a 2D array from a scalar value using numpy.atleast_2d()
>>> import numpy as np
>>> np.atleast_2d(5.0)
array([[ 5.]])
In the above code, the np.atleast_2d() function is used to create a 2D array from a scalar value of 5.0. The resulting array is a 1x1 matrix with the value 5.0.
Pictorial Presentation:
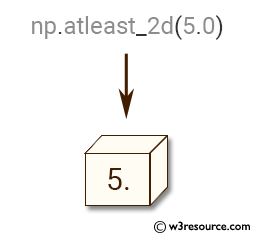
Example: Creating at least 2-dimensional array using numpy.atleast_2d()
>>> import numpy as np
>>> a = np.arange(4.0)
>>> np.atleast_2d(a)
array([[ 0., 1., 2., 3.]])
In the above code the second line, a 1-dimensional array with four elements, ranging from 0 to 3, is created using the arange() function. In the third line, the np.atleast_2d() function is used to convert the 1-dimensional array 'a' into a 2-dimensional array with a single row and multiple columns.
Pictorial Presentation:
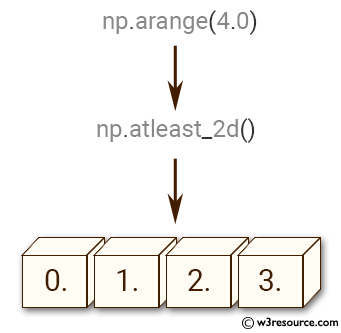
Example: Using numpy.atleast_2d() function to ensure at least two dimensions in an array
>>> import numpy as np
>>> a = np.arange(4.0)
>>> np.atleast_2d(a).base is a
True
>>> np.atleast_2d(1, [1,2], [[2,3]])
[array([[1]]), array([[1, 2]]), array([[2, 3]])]
In the first example, we create a one-dimensional array a using np.arange(). The np.atleast_2d(a) function is then used to convert a to a 2D array with a single row. The .base attribute of the resulting array is used to check if it shares the same memory as 'a'.
In the second example, the np.atleast_2d() function is used to convert a scalar, a 1D array, and a 2D array into a 2D array, a 2D array, and a 2D array, respectively. The result is a list of arrays with at least two dimensions.
Python - NumPy Code Editor:
Previous: atleast_1d()
Next: atleast_3d()
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/numpy/manipulation/atleast-2d.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics