NumPy: numpy.broadcast() function
numpy.broadcast() function
The numpy.broadcast() function produces an object that mimics broadcasting. Broadcasting is the concept of making arrays with different shapes compatible for arithmetic operations.
The numpy.broadcast() function is used to apply binary operations to arrays of different shapes. It creates an iterator that can be used to perform operations on corresponding elements of the input arrays.
Syntax:
class numpy.broadcast(in1, in2, . . .)
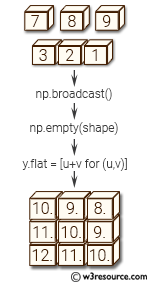
Parameter:
Name | Description | Required / Optional |
---|---|---|
in1, in2, . . . | Input parameters. | Required |
Return value:
Broadcast the input parameters against one another, and return an object that encapsulates the result. Amongst others, it has shape and nd properties, and may be used as an iterator.
Example: Broadcasting Arrays in Numpy using numpy.broadcast()
>>> import numpy as np
>>> a = np.array([[2], [3], [4]])
>>> b = np.array([5, 6, 7])
>>> z = np.broadcast(a, b)
>>> y = np.empty(z.shape)
>>> y.flat = [u+v for (u,v) in z]
>>> y
array([[ 7., 8., 9.],
[ 8., 9., 10.],
[ 9., 10., 11.]])
In the above code, two arrays a and b are defined with shapes (3,1) and (3,), respectively. The numpy.broadcast() function creates an iterator object z that represents the shape of the output array resulting from the element-wise operation between a and b. In this case, the output array has shape (3,3).
An empty array 'y' is created with shape (3,3) to store the result of the element-wise operation between 'a' and 'b'. The flat attribute of y is used to assign the values resulting from the element-wise addition between 'a' and 'b' to 'y'.
The resulting array 'y' is the element-wise addition of 'a' and 'b', where each element of 'a' is added to every element of 'b'.
Example: Broadcasting in NumPy
>>> import numpy as np
>>> a = np.array([[2], [3], [4]])
>>> b = np.array([5, 6, 7])
>>> a+b
array([[ 7, 8, 9],
[ 8, 9, 10],
[ 9, 10, 11]])
In this specific example, we have two arrays 'a' and 'b'. Array a has shape (3,1) and array b has shape (3,). When we add them together using the "+" operator, NumPy applies broadcasting rules to align the shapes of the two arrays so that they can be added element-wise.
The resulting array has shape (3,3), where each element in the output is the sum of the corresponding elements in arrays 'a' and 'b'.
Pictorial Presentation:
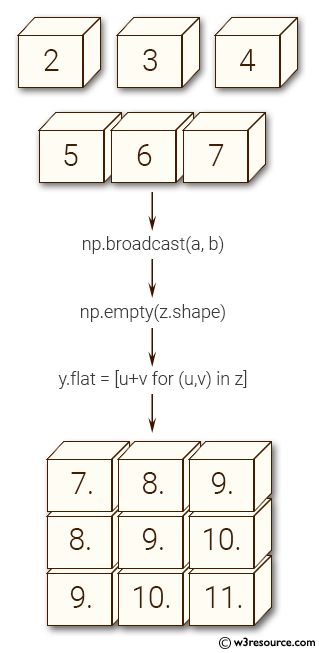
Python - NumPy Code Editor:
Previous: atleast_3d()
Next: broadcast_to()