NumPy: numpy.moveaxis() function
numpy.moveaxis() function
The numpy.moveaxis() function is used to move axes of an array to new positions.
Other axes remain in their original order. The function takes three arguments: the input array, the source axis (or axes) to be moved, and the destination position(s) to which the source axis (or axes) should be moved.
This function is particularly useful when dealing with arrays of high dimensionality, as it provides a convenient way to reorder the axes of the array to match the expected dimensions of a particular computation or operation.
Syntax:
numpy.moveaxis(a, source, destination)
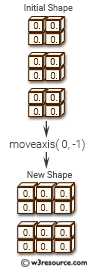
Parameters:
Name | Description | Required / Optional |
---|---|---|
a | The array whose axes should be reordered. | Required |
source | Original positions of the axes to move. These must be unique. | Required |
destination | Destination positions for each of the original axes. These must also be unique. | Required |
Return value:
result [np.ndarray] Array with moved axes. This array is a view of the input array
Example: Reshaping and moving axes in Numpy arrays
>> import numpy as np
>>> y = np.zeros((2,3,4))
>>> print("Initial Shape:",y.shape)
Initial Shape: (2, 3, 4)
>>> print(y)
[[[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]]
[[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]]]
>>> z = np.moveaxis(y, 0, -2)
>>> print("\nNew Shape:",z.shape)
New Shape: (3, 2, 4)
>>> print(z)
[[[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]]
[[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]]
[[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]]]
In the above code, a 3-dimensional array 'y' of shape (2,3,4) is created using the np.zeros() function. The shape and contents of y are printed to the console.
Next, the np.moveaxis() function is used to move the 0th axis (the first dimension) to the -2 position (second to last dimension), resulting in a new array z with shape (3,2,4). The shape and contents of z are printed to the console.
Pictorial Presentation:
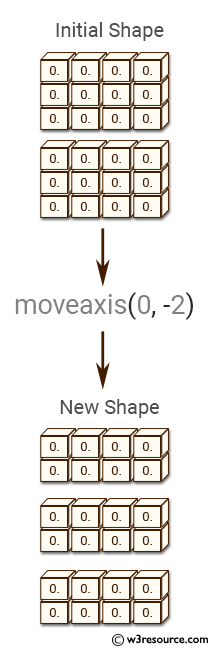
Example: Reordering axes in NumPy array using numpy.moveaxis()
>> import numpy as np
>>> y = np.zeros((2,3,4))
>>> print("Initial Shape:",y.shape)
Initial Shape: (2, 3, 4)
>>> print(y)
[[[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]]
[[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]]]
>>> z = np.moveaxis(y, -1, 0)
>>> print("\nNew Shape:",z.shape)
New Shape: (4, 2, 3)
>>> print(z)
[[[ 0. 0. 0.]
[ 0. 0. 0.]]
[[ 0. 0. 0.]
[ 0. 0. 0.]]
[[ 0. 0. 0.]
[ 0. 0. 0.]]
[[ 0. 0. 0.]
[ 0. 0. 0.]]]
In the above code, a 3-dimensional array of size 2x3x4 filled with zeros is created using the np.zeros() function. Its shape is printed to the console, which is (2, 3, 4). The array is also printed to show the initial values.
Then, the np.moveaxis() function is used to move the last axis to the first position. The y array is passed as the first argument, and the source and destination axes are specified as -1 and 0 respectively. The resulting array is assigned to the variable z.
The shape of the new array is printed, which is (4, 2, 3) as expected. The z array is also printed to show that the last axis has become the first axis in the new array.
Example: Moving axes in a Numpy array using numpy.moveaxis()
>> import numpy as np
>>> y = np.zeros((4,5,6))
>>> print("Initial Shape:",y.shape)
Initial Shape: (4, 5, 6)
>>> print(y)
[[[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]]
[[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]]
[[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]]
[[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]]]
>>> z = np.moveaxis(y, 0,-1)
>>> print("\nNew Shape:",z.shape)
New Shape: (5, 6, 4)
>>> print(z)
[[[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]]
[[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]]
[[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]]
[[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]]
[[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]]]
In the above code, initially, a 3-dimensional Numpy array y with shape (4,5,6) is created and filled with zeros. Then, the np.moveaxis() function is used to move the first axis (axis 0) to the last position (axis -1) in the array, resulting in a new array z with shape (5,6,4). Finally, both the initial and new shapes and arrays are printed to the console.
Example: Transpose of a 3D Numpy Array
>> import numpy as np
>>> y = np.zeros((4,5,6))
>>> print("Initial Shape:",y.shape)
Initial Shape: (4, 5, 6)
>>> print(y)
[[[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]]
[[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]]
[[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]]
[[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0. 0.]]]
>>> z = np.moveaxis(y, -1, 0)
>>> print("\nNew Shape:",z.shape)
New Shape: (6, 4, 5)
>>> print(z)
[[[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
[[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
[[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
[[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
[[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
[[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]]
In the above code, a 3D array y is created using the np.zeros() function with shape (4, 5, 6). The shape and values of y are printed using the shape and print functions.
Next, the np.moveaxis() function is used to transpose y by moving the last axis to the first position. The resulting array is assigned to a new variable 'z'. The shape and values of 'z' are printed using the shape and print functions.
The resulting transposed array 'z' has a shape of (6, 4, 5), meaning that the original last dimension of y (length 6) is now the first dimension of 'z'. The values in 'z' are the same as those in 'y', but with the dimensions rearranged.
Python - NumPy Code Editor:
Previous: ndarray.flatten()
Next: rollaxis()
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics