NumPy: numpy.stack() function
numpy.stack() function
The numpy.stack() function is used to join a sequence of arrays along a new axis.
The axis parameter specifies the index of the new axis in the dimensions of the result. For example, if axis=0 it will be the first dimension and if axis=-1 it will be the last dimension.
numpy.stack() is useful when working with machine learning models that require a single input array. For example, when working with image data, it is common to have multiple image files that need to be joined into a single array for processing by the machine learning model.
Syntax:
numpy.stack(arrays, axis=0, out=None)
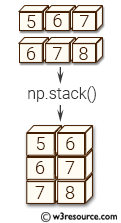
Parameters:
Name | Description | Required / Optional |
---|---|---|
arrays | A sequence of arrays that must have the same shape. | Required |
axis | The axis in the result array along which the input arrays are stacked. The default is 0. | Optional |
out | If provided, the destination to place the result. The shape must match what stack would have returned if no out argument were specified. | Optional |
Return value:
stacked: ndarray
The stacked array has one more dimension than the input arrays.
Example 1: Stacking 2-D Arrays Vertically
import numpy as np
arrays = [np.random.randn(2, 3) for _ in range(8)]
result = np.stack(arrays, axis=0)
print(result.shape)
Output:
(8, 2, 3)
In this example, a list of eight 2x3 arrays is created. The np.stack() function stacks these arrays along the first axis, resulting in an 8x2x3 array.
Example 2: Stacking 2-D Arrays Along Different Axes
import numpy as np
arrays = [np.random.randn(2, 3) for _ in range(8)]
result_axis_1 = np.stack(arrays, axis=1)
result_axis_2 = np.stack(arrays, axis=2)
print(result_axis_1.shape)
print(result_axis_2.shape)
Output:
(2, 8, 3) (2, 3, 8)
Here, the same list of arrays is stacked along different axes. When stacked along axis=1, the shape becomes (2, 8, 3). When stacked along axis=2, the shape becomes (2, 3, 8).
Example 3: Stacking 1-D Arrays
import numpy as np
x = np.array([2, 3, 4])
y = np.array([3, 4, 5])
result_default = np.stack((x, y))
result_axis_neg1 = np.stack((x, y), axis=-1)
print(result_default)
print(result_axis_neg1)
Output:
[[2 3 4] [3 4 5]] [[2 3] [3 4] [4 5]]
In this example, two 1-D arrays x and y are stacked. By default, they are stacked along axis=0, creating a 2x3 array. When stacked along axis=-1, the result is a 3x2 array.
Visual Presentation:
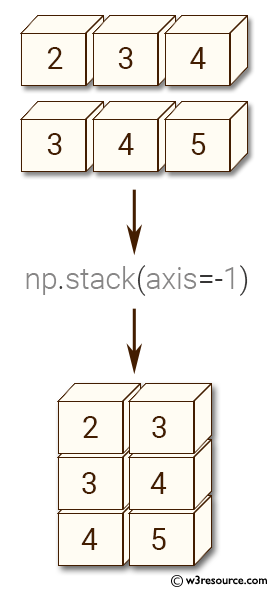
Frequently Asked Questions (FAQ): numpy.stack() Function
1. What does the numpy.stack() function do?
The numpy.stack() function joins a sequence of arrays along a new axis.
2. Why is numpy.stack() useful?
It is useful for combining multiple arrays into a single array, especially for preparing data for machine learning models that require input in a specific shape.
3. What must be true about the arrays used with numpy.stack()?
All arrays must have the same shape.
4. How does the axis parameter affect numpy.stack()?
The axis parameter specifies the index of the new axis in the resulting array. For example, axis=0 adds a new first dimension, and axis=-1 adds a new last dimension.
Python - NumPy Code Editor:
Previous: concatenate()
Next: column_stack()