Numpy: numpy.fliplr() function
numpy.fliplr() function
The numpy.fliplr() function is used to flip array in the left/right direction. Flip the entries in each row in the left/right direction. Columns are preserved, but appear in a different order than before.
This function is particularly useful for image processing tasks such as flipping an image horizontally. It is also useful in data preprocessing tasks for machine learning models where flipping the features of a dataset can improve the accuracy of the model.
Syntax:
numpy.fliplr(m)
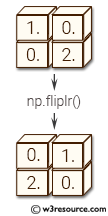
Parameter:
Name | Description | Required / Optional |
---|---|---|
m | Input array, must be at least 2-D. | Required |
Return value:
ndarray - A view of m with the columns reversed. Since a view is returned, this operation is \mathcal O(1).
Example: Flipping a 2D numpy array horizontally using numpy.fliplr()
>>> import numpy as np
>>> X = np.diag([1.,2.,3.,4.])
>>> np.fliplr(X)
array([[ 0., 0., 0., 1.],
[ 0., 0., 2., 0.],
[ 0., 3., 0., 0.],
[ 4., 0., 0., 0.]])
In the above code, a diagonal matrix is created using the numpy.diag() function, with diagonal elements [1, 2, 3, 4]. Then, the numpy.fliplr() function is applied to X to flip the array horizontally. This function flips the array in the left-right direction by reversing the order of columns in the input array.
Pictorial Presentation:
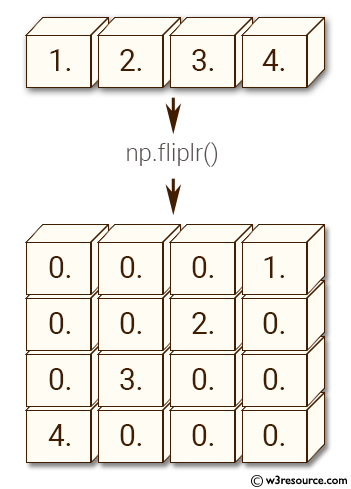
Example: Reversing the order of an array in one axis using numpy.fliplr()
>>> import numpy as np
>>> X = np.random.randn(3,5,7)
>>> np.all(np.fliplr(X) == X[:,::-1,...])
True
The code creates a 3D array X of shape (3,5,7) filled with random numbers using numpy's random.randn() function.
Then, it uses numpy's fliplr() function to reverse the order of elements in the second axis of the X array. The fliplr() function flips the array in the left/right direction, meaning it reverses the order of elements along the second axis of the array.
The code then uses numpy's all() function to check if the output of fliplr() applied to X is equal to the output of reversing the order of elements along the second axis of X using slicing (X[:,::-1,...]). The all() function returns True if all elements of the resulting arrays are equal and False otherwise.
Python - NumPy Code Editor:
Previous: Rearrangeing elements flip()
Next: flipud()