NumPy: numpy.transpose() function
numpy.transpose() function
The numpy.transpose() function is used to reverse or permute the dimensions of an array. It returns a view of the original array with the axes transposed.
The numpy.transpose() function can be useful in various applications such as image processing, signal processing, and numerical analysis.
Syntax:
numpy.transpose(a, axes=None)
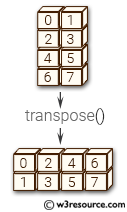
Parameters:
Name | Description | Required / Optional |
---|---|---|
a | Input array. | Required |
axis | By default, reverse the dimensions, otherwise permute the axes according to the values given. | Optional |
Return value:
[ndarray]: a with its axes permuted. A view is returned whenever possible.
Example: Transpose of a 2D array using numpy.transpose() function
>>> import numpy as np
>>> a = np.arange(6).reshape((3,2))
>>> np.transpose(a)
array([[0, 2, 4],
[1, 3, 5]])
In the above code we create a 2D array 'a' with 3 rows and 2 columns using the arange() and reshape() functions. Then, we apply the transpose() function to the array 'a'. The transpose() function takes the array as its argument and returns a new array with its rows and columns swapped.
Pictorial Presentation:
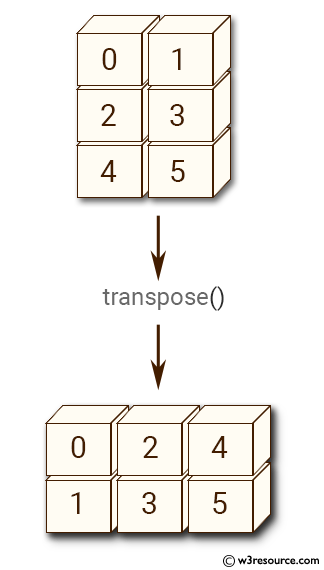
Example: Transposing multi-dimensional arrays in NumPy
>>> import numpy as np
>>> a = np.ones((2,3,4))
>>> np.transpose(a,(1,0,2)).shape
(3, 2, 4)
>>> np.transpose(a,(2,1,0)).shape
(4, 3, 2)
In the above code, a 3-dimensional array a is created using the ones() function, which creates an array of specified shape and fills it with ones. The shape of the array is (2,3,4).
The second line of code calls transpose() function with the argument (1,0,2), which indicates the desired order of axes in the output. The resulting array has a shape of (3,2,4), where the first axis of the input array is swapped with the second axis.
In the third line, transpose() is called with the argument (2,1,0), which swaps all the axes of the input array. The resulting array has a shape of (4,3,2).
Python - NumPy Code Editor:
Previous: ndarray.T()
Next: Changing number of dimensions atleast_1d()
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics