NumPy: numpy.insert() function
numpy.insert() function
The numpy.insert() function is used to insert values along the given axis before the given indices.
The function can be used to insert a single value or multiple values into an existing array. The function also provides the option to insert values at specific locations within the array.
Syntax:
numpy.insert(arr, obj, values, axis=None)
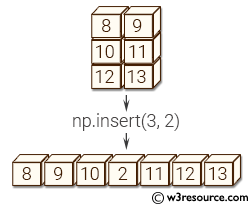
Parameters:
Name | Description | Required / Optional |
---|---|---|
arr | Input array. | Required |
obj | Object that defines the index or indices before which values is inserted.
Support for multiple insertions when obj is a single scalar or a sequence with one element (similar to calling insert multiple times). |
Required |
values | [array_like] Values to insert into arr. If the type of values is different from that of arr, values is converted to the type of arr. values should be shaped so that arr[...,obj,.. .] = values is legal | Optional |
axis | Axis along which to insert values. If axis is None then arr is flattened first. | Optional |
Return value:
out [ndarray] A copy of arr with values inserted. Note that insert does not occur in-place: a new array is returned. If axis is None, out is a flattened array.
Example: Inserting elements in a NumPy array
>>> import numpy as np
>>> x = np.array([[0,0], [1,1], [2,2]])
>>> x
array([[0, 0],
[1, 1],
[2, 2]])
>>> np.insert(x, 2, 4)
array([0, 0, 4, 1, 1, 2, 2])
>>> np.insert(x, 2, 4, axis=1)
array([[0, 0, 4],
[1, 1, 4],
[2, 2, 4]])
In the above code an array 'x' is created. The 'x' array contains 3 rows and 2 columns.
In the next line, numpy.insert() function is used to insert the value 4 at index 2 of the flattened 'x' array. This results in a new flattened array with the value 4 inserted at the 2nd index.
In the third line, np.insert() function is used to insert the value 4 in the second column of 'x' array at index 2. This results in a new 2D array where the value 4 is inserted in the 2nd column of each row at index 2.
Pictorial Presentation:
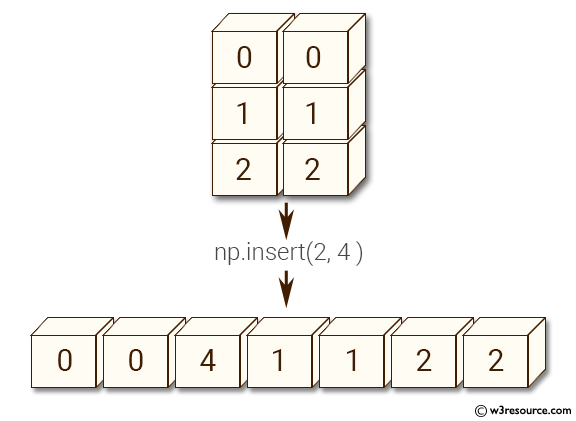
Example: Inserting values in a NumPy array along an axis using numpy.insert()
>>> import numpy as np
>>> x = np.array([[0,0], [1,1], [2,2]])
>>> np.insert(x, [1], [[3], [4], [5]], axis=1)
array([[0, 3, 0],
[1, 4, 1],
[2, 5, 2]])
>>> np.array_equal(np.insert(x, 1, [3, 4, 5], axis=1), np.insert(x, [1], [[3], [4], [5]], axis=1))
True
In the above code-
- The first line of code creates a NumPy array x of shape (3, 2) and stores it in the variable x.
- The second line of code inserts the scalar value 4 at index 2 of the flattened array (which is equivalent to the index (0, 2) in the original array) using the np.insert() function. The resulting array is flattened and returned as a one-dimensional array of shape (7,).
- The third line of code inserts the one-dimensional arrays [3], [4], and [5] at index 1 of each row of the two-dimensional array x using the np.insert() function. The resulting array has the shape (3, 3) and the values [3, 4, 5] are inserted as the second column of each row.
- The fourth line of code demonstrates that the two different ways of inserting values, using a single array or multiple arrays, are equivalent and produce the same result. The np.array_equal() function returns True if the two arrays are equal element-wise.
Pictorial Presentation:
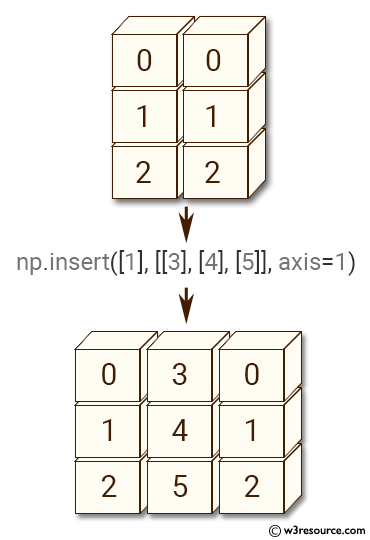
Example: Inserting values into flattened arrays using NumPy
>>> import numpy as np
>>> x = np.array([[0,0], [1,1], [2,2]])
>>> y = x.flatten()
>>> y
array([0, 0, 1, 1, 2, 2])
>>> np.insert(y, [3,3], [6,7])
array([0, 0, 1, 6, 7, 1, 2, 2])
>>> np.insert(y, slice(3,5),[7,8])
array([0, 0, 1, 7, 1, 8, 2, 2])
>>> np.insert(y, [3,3], [8.12, False])
array([0, 0, 1, 8, 0, 1, 2, 2])
In the above code, first, a NumPy array x is created with shape (3,2) containing some values. Then, the flatten() method is applied on x which returns a flattened array of shape (6,).
Next, the insert() function is used to insert values into the flattened array.
In the first example, insert() is used to insert values 6 and 7 at index 3 twice. As a result, the original array is modified and the output array contains the values [0, 0, 1, 6, 7, 1, 2, 2].
In the second example, insert() is used with slice(3,5) to insert values 7 and 8 at index 3 and 4. As a result, the output array is [0, 0, 1, 7, 8, 1, 2, 2].
In the third example, insert() is used to insert the values 8.12 and False at index 3 twice. Since the original array contains integer values, the inserted values are also coerced to integer type. As a result, the output array becomes [0, 0, 1, 8, 0, 1, 2, 2].
Example: Inserting columns with specific indices in a NumPy array using numpy.insert()
>>> import numpy as np
>>> z = np.arange(12).reshape(3,4)
>>> idz = (1, 3)
>>> np.insert(z, idz, 777, axis=1)
array([[ 0, 777, 1, 2, 777, 3],
[ 4, 777, 5, 6, 777, 7],
[ 8, 777, 9, 10, 777, 11]])
The above code defines a 3x4 NumPy array z using np.arange() and reshape() methods. Then, a tuple idz is defined as (1, 3).
Using np.insert() method, the code inserts a new column with value 777 at indices defined by idz along the second dimension (i.e., axis=1) of the z array. As a result, two new columns are inserted at index 1 and 3 in z, shifting the existing columns to the right. The resulting array has shape (3, 6), with the inserted columns filled with the value 777.
Pictorial Presentation:
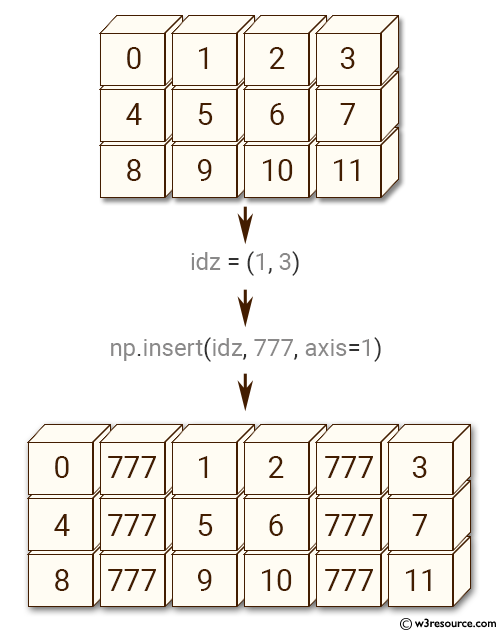
Python - NumPy Code Editor: