NumPy: numpy.flipud() function
numpy.flipud() function
The numpy.flipud() function is used to flip a given array in the up/down direction.
Flip the entries in each column in the up/down direction. Rows are preserved, but appear in a different order than before.
The function is particularly useful in image processing tasks where flipping an image vertically is required, or in tasks where the order of rows in an array needs to be reversed.
Syntax:
numpy.flipud(m)
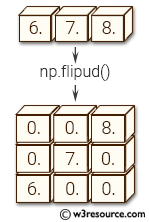
Parameter:
Name | Description | Required / Optional |
---|---|---|
m | Input array. | Required |
Return value:
out : array_like - A view of m with the rows reversed. Since a view is returned, this operation is \mathcal O(1).
Example: Flipping an array upside down using numpy.flipud()
>>> import numpy as np
>>> X = np.diag([1.0, 3, 5])
>>> np.flipud(X)
array([[ 0., 0., 5.],
[ 0., 3., 0.],
[ 1., 0., 0.]])
In the above code, we have first created a 3x3 square diagonal matrix X using the np.diag() function in NumPy. The diagonal elements of this matrix are 1.0, 3, and 5.
Next, we have used the np.flipud() function to flip the X array vertically. The resulting array after flipping X upside down is a 3x3 square diagonal matrix where the diagonal elements are in reverse order i.e., 5, 3, and 1.
Pictorial Presentation:
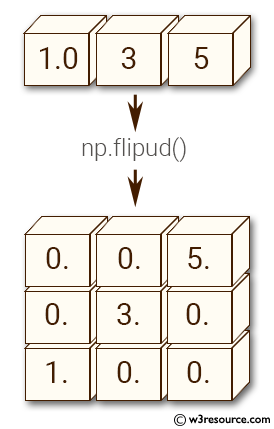
Example: Flipping arrays vertically with numpy.flipud()
>>> import numpy as np
>>> X = np.random.randn(3,5,7)
>>> np.all(np.flipud(X) == X[::-1,...])
True
In the above code first, a 3D NumPy array X with dimensions 3x5x7 is created with random values using the numpy.random.randn() function.
Then, numpy.flipud(X) is used to create a new array with the same data as X, but flipped vertically. This is achieved by reversing the order of the rows in X.
Finally, the code uses the numpy.all() function to verify that the elements in the flipped array created by numpy.flipud() are the same as those obtained by using the slicing syntax X[::-1,...].
Python - NumPy Code Editor:
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics