NumPy: numpy.flip() function
numpy.flip() function
The numpy.flip() function is used to reverse the order of elements in an array along the given axis. The shape of the array is preserved, but the elements are reordered.
The function reverse the order of elements in an array to help with processing data in reverse order, or to transform data in a way that is required by a particular application or algorithm. Additionally, it can be used in image processing applications to flip images horizontally or vertically.
Syntax:
numpy.flip(m, axis=None)
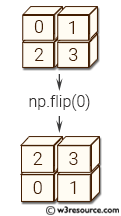
Parameters:
Name | Description | Required / Optional |
---|---|---|
m | Input array. | Required |
axis | Axis or axes along which to flip over. The default, axis=None, will flip over all of the axes of the input array. If axis is negative it counts from the last to the first axis. If axis is a tuple of ints, flipping is performed on all of the axes specified in the tuple. |
Optional |
Return value:
out : array_like - A view of m with the entries of axis reversed. Since a view is returned, this operation is done in constant time.
Example: Flipping and Reversing NumPy Arrays with numpy.flip()
>>> import numpy as np
>>> X = np.arange(8).reshape((2,2,2))
>>> np.flip(X, 0)
array([[[4, 5],
[6, 7]],
[[0, 1],
[2, 3]]])
>>> np.flip(X, 1)
array([[[2, 3],
[0, 1]],
[[6, 7],
[4, 5]]])
>>> np.flip(X)
array([[[7, 6],
[5, 4]],
[[3, 2],
[1, 0]]])
>>> np.flip(X, (0, 2))
array([[[5, 4],
[7, 6]],
[[1, 0],
[3, 2]]])
>>> X = np.random.randn(3,4,5)
>>> np.all(flip(X,2) == X[:,:,::-1,...])
True
The above code demonstrates the usage of the NumPy np.flip() function-
- In the first line of code, an array X of shape (2,2,2) is created using the np.arange() function, which generates a sequence of numbers. The reshape() method is then used to reshape the array into a three-dimensional array.
- The second line of code demonstrates flipping the array along the first axis (axis=0), resulting in a new array with the same values, but with the rows of the original array flipped in reverse order.
- The third line of code demonstrates flipping the array along the second axis (axis=1), resulting in a new array with the same values, but with the columns of the original array flipped in reverse order.
- The fourth line of code demonstrates flipping the array along all axes (default), resulting in a new array with the same values, but with all dimensions of the original array flipped in reverse order.
- The fifth line of code demonstrates flipping the array along multiple axes simultaneously, which can be achieved by passing a tuple of axis numbers.
- Finally, the sixth line of code creates a random array X of shape (3,4,5) and uses np.flip() to flip it along the third axis, and then compares it with the equivalent operation of reversing the same axis using slicing (X[:,:,::-1,...]). The np.all() function is used to check if all elements of the two arrays are equal, and the output is True.
Pictorial Presentation:
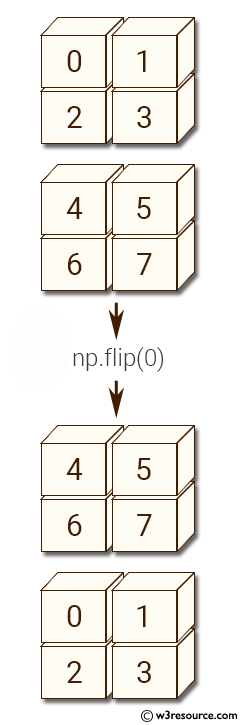
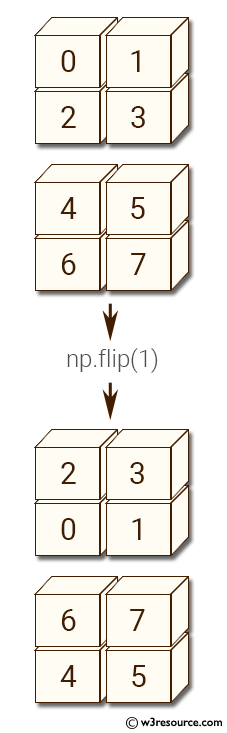
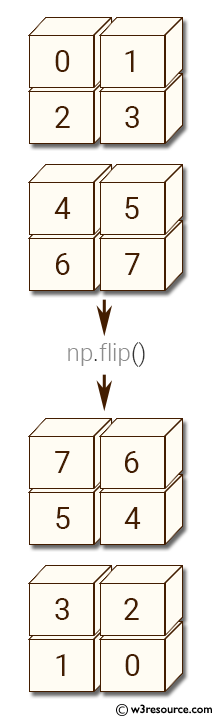
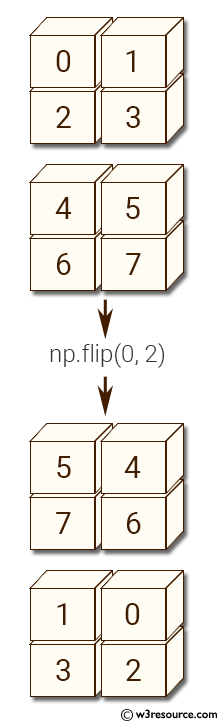
Python - NumPy Code Editor: