NumPy: numpy.broadcast_arrays() function
numpy.broadcast_arrays() function
The numpy.broadcast_arrays() function broadcasts any number of arrays against each other. For example, if we have two arrays with different shapes and we want to perform an operation on them, we can use broadcast_arrays() to create new arrays with the same shape, so that the operation can be performed.
Syntax:
numpy.broadcast_arrays(*args, **kwargs)
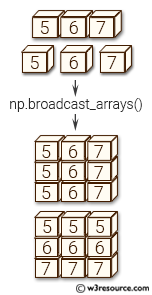
Parameters:
Name | Description | Required / Optional |
---|---|---|
*args | The arrays to broadcast. | Required |
subok | If True, then sub-classes will be passed-through, otherwise the returned arrays will be forced to be a base-class array (default). | Optional |
Return value:
broadcasted [list of arrays] These arrays are views on the original arrays. They are typically not contiguous. Furthermore, more than one element of a broadcasted array may refer to a single memory location. If you need to write to the arrays, make copies first.
Example: Broadcasting Arrays with numpy.broadcast_arrays()
>>> import numpy as np
>>> a = np.array([[2,3,4]])
>>> b = np.array([[2],[3],[4]])
>>> np.broadcast_arrays(a, b)
[array([[2, 3, 4],
[2, 3, 4],
[2, 3, 4]]), array([[2, 2, 2],
[3, 3, 3],
[4, 4, 4]])]
In the above code, the input arrays are "a" and "b", where "a" is a 2D array with shape (1, 3) and "b" is a 2D array with shape (3, 1). The numpy.broadcast_arrays() function returns a list of two arrays. The first array has a shape of (3, 3) and contains repeated copies of "a" along the rows. The second array has a shape of (3, 3) and contains repeated copies of "b" along the columns.
This operation is useful when we want to perform element-wise operations between arrays that have different shapes. Broadcasting allows us to align the shapes of the arrays so that they are compatible for element-wise operations, which can be more efficient than looping over the arrays.
Pictorial Presentation:
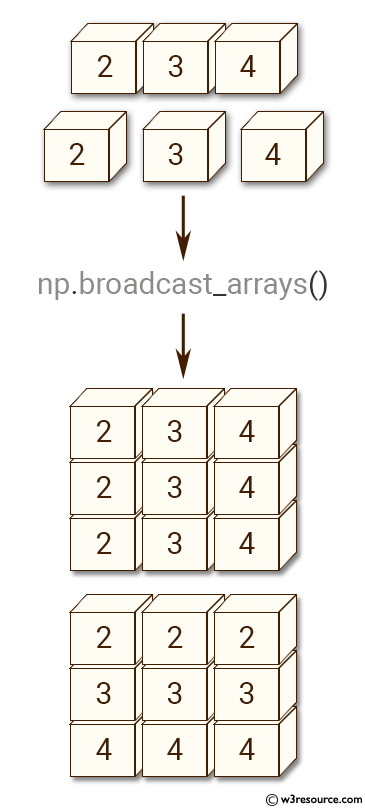
Python - NumPy Code Editor:
Previous: broadcast_to()
Next: expand_dims()