NumPy: numpy.dsplit() function
numpy.dsplit() function
The numpy.dsplit() function is used to split an array into multiple sub-arrays.
The only difference between these functions is that dsplit allows indices_or_sections to be an integer that does not equally divide the axis. For an array of length l that should be split into n sections, it returns l % n sub-arrays of size l//n + 1 and the rest of size l//n.
The numpy.dsplit() function is useful when working with three-dimensional arrays, such as image data.
Syntax:
numpy.dsplit(ary, indices_or_sections, axis=0)
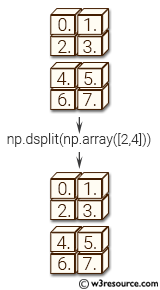
Example: Creating a 3D array using NumPy arange and reshape functions
>>> import numpy as np
>>> a = np.arange(12.0).reshape (2,2,3)
>>> a
array([[[ 0., 1., 2.],
[ 3., 4., 5.]],
[[ 6., 7., 8.],
[ 9., 10., 11.]]])
In the above code we use the NumPy 'arange' function to create an array of numbers from 0 to 11 (12 elements), and we reshape this array into a 3-dimensional array with shape (2, 2, 3) using the 'reshape' function.
The resulting array 'a' has 2 layers, each with 2 rows and 3 columns. The first layer has the elements 0 to 5, and the second layer has the elements 6 to 11.
Pictorial Presentation:
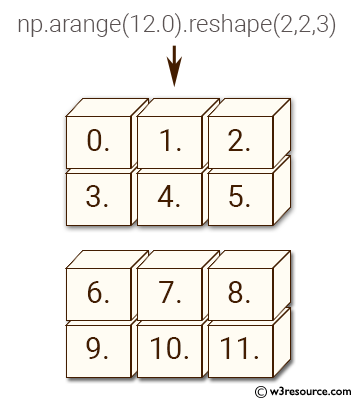
Example: Splitting a 3D array along the third dimension using numpy.dsplit()
>>> import numpy as np
>>> a = np.arange(12.0).reshape (2,2,3)
>>> np.dsplit(a,3)
[array([[[ 0.],
[ 3.]],
[[ 6.],
[ 9.]]]), array([[[ 1.],
[ 4.]],
[[ 7.],
[ 10.]]]), array([[[ 2.],
[ 5.]],
[[ 8.],
[ 11.]]])]
>>> np.dsplit(a, np.array([2,6]))
[array([[[ 0., 1.],
[ 3., 4.]],
[[ 6., 7.],
[ 9., 10.]]]), array([[[ 2.],
[ 5.]],
[[ 8.],
[ 11.]]]), array([], shape=(2, 2, 0), dtype=float64)]
In the above code first, a 3D numpy array 'a' of shape (2, 2, 3) is created using np.arange() and np.reshape() methods. It contains two 2x3 matrices stacked along the first dimension.
Then, np.dsplit() function is used to split the array 'a' into three arrays of shape (2, 2, 1), each containing the corresponding elements from the third dimension. The output is a list of three arrays.
Next, np.dsplit() function is used again with an array of splitting points [2, 6]. The output is a list of three arrays. The first array contains the elements of the third dimension up to index 2, the second array contains the elements from index 2 to 6, and the third array contains an empty array since there are no elements after index 6.
Python - NumPy Code Editor:
Previous: array_split()
Next: hsplit()