NumPy: numpy.rot90() function
numpy.rot90() function
The numpy.rot90() function is used to rotate an array by 90 degrees in the plane specified by axes. Rotation direction is from the first towards the second axis.
The function can be used to rotate an image or matrix in various applications such as computer vision, image processing, and data analysis.
Syntax:
numpy.rot90(m, k=1, axes=(0, 1))
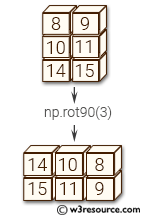
Parameters:
Name | Description | Required / Optional |
---|---|---|
m | Array of two or more dimensions. | Required |
k | Number of times the array is rotated by 90 degrees. | Required |
axes | The array is rotated in the plane defined by the axes. Axes must be different. | Required |
Return value:
y : ndarray - A rotated view of m.
Example: Rotating a matrix using numpy.rot90()
>>> import numpy as np
>>> m = np.array([[1,2], [3,4], [5,6]], int)
>>> m
array([[1, 2],
[3, 4],
[5, 6]])
>>> np.rot90(m)
array([[2, 4, 6],
[1, 3, 5]])
In the above code first, a 2D matrix 'm' of size 3x2 is created using the numpy.array() function. Then, this matrix is printed using the print() function.
Next, the numpy.rot90() function is applied to this matrix, which rotates the matrix by 90 degrees counterclockwise. The resulting rotated matrix is then printed using the print() function.
Pictorial Presentation:
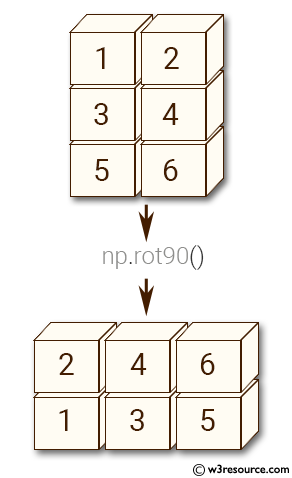
Example: Rotating a matrix by 270 degrees using numpy.rot90()
>>> import numpy as np
>>> m = np.array([[1,2], [3,4], [5,6]], int)
>>> np.rot90(m, 3)
array([[5, 3, 1],
[6, 4, 2]])
In the above code, original matrix "m" is created using the np.array() function and consists of 3 rows and 2 columns of integer values.
The np.rot90() function is then called with the matrix "m" and the parameter "3" which specifies the number of 90-degree rotations to apply. The resulting rotated matrix has 2 rows and 3 columns and is printed to the console.
The resulting matrix is obtained by rotating the original matrix 270 degrees counterclockwise.
Pictorial Presentation:
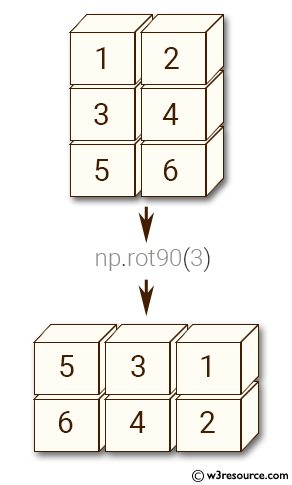
Python - NumPy Code Editor:
Previous: roll()
Next: NumPy Binary operation Home