NumPy: numpy.split() function
numpy.split() function
The numpy.split() function is used to split an array into multiple sub-arrays. It takes three arguments: the first argument is the array to be split, the second argument is the number of splits to be performed, and the third argument is the axis along which the array is to be split.
This function is useful in cases where we want to split large datasets into smaller, more manageable subsets for processing or analysis. It can also be used to split an array into smaller, more easily manipulated pieces for more complex calculations or to extract specific elements of an array.
Syntax:
numpy.split(ary, indices_or_sections, axis=0)
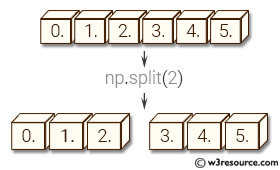
Parameters:
Name | Description | Required / Optional |
---|---|---|
ary | Array to be divided into sub-arrays. | Required |
indices_or_sections | If indices_or_sections is an integer, N, the array will be divided into N equal arrays along axis. If such a split is not possible, an error is raised. If indices_or_sections is a 1-D array of sorted integers, the entries indicate where along axis the array is split. For example, [2, 3] would, for axis=0, result in
|
Required |
axis | The axis along which to split, default is 0. | Optional |
Return value:
sub-arrays : list of ndarrays A list of sub-arrays.
Raises: ValueError - If indices_or_sections is given as an integer, but a split does not result in equal division.
Example: Splitting an array into multiple sub-arrays using numpy.split()
>>> import numpy as np
>>> a = np.arange(8.0)
>>> np.split(a, 2)
[array([ 0., 1., 2., 3.]), array([ 4., 5., 6., 7.])]
In the above code first, an array a of length 8 with values from 0 to 7 is created using np.arange(). Then, the np.split() function is called with two arguments - the array to be split (a), and the number of sub-arrays to split it into (2).
Pictorial Presentation:
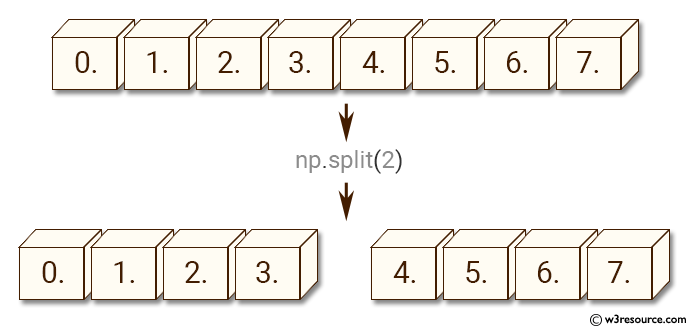
Example: Splitting an array at specified positions using numpy.split()
>>> import numpy as np
>>> a = np.arange(6.0)
>>> np.split(a, [4, 5, 6, 7])
[array([ 0., 1., 2., 3.]), array([ 4.]), array([ 5.]), array([], dtype=float64), array([], dtype=float64)]
In the above code, the numpy.split() function is used to split an input array 'a' at specified positions given as a list argument [4, 5, 6, 7]. This will split the array 'a' into subarrays at the positions 4, 5, 6, and 7.
The input array 'a' is a one-dimensional array created using the numpy.arange() function that generates a sequence of floats from 0 to 5.
Python - NumPy Code Editor:
Previous: block()
Next: array_split()