NumPy: numpy.asarray_chkfinite() function
numpy.asarray_chkfinite() function
The numpy.asarray_chkfinite() function is used to convert the input to an array, checking for NaN or infinity values. If the input array contains any NaN or infinity values, it raises a ValueError.
This function can be useful when working with data that should not contain any invalid values, such as in scientific or financial calculations.
Syntax:
numpy.asarray_chkfinite(a, dtype=None, order=None)
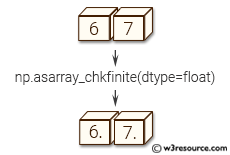
Parameters:
Name | Description | Required / Optional |
---|---|---|
a | Input data, in any form that can be converted to an array. This includes lists, lists of tuples, tuples, tuples of tuples, tuples of lists and ndarrays. Success requires no NaNs or infinity values. | Required |
dtype | By default, the data-type is inferred from the input data. | Optional |
Return value:
out : ndarray - Array interpretation of a. No copy is performed if the input is already an ndarray. If a is a subclass of ndarray, a base class ndarray is returned.
Example: Converting a list to a NumPy array with data type checking
>>> import numpy as np
>>> a = [2, 4]
>>> np.asarray_chkfinite(a, dtype=float)
array([ 2., 4.])
In the above code, the input list 'a' contains integer values. By specifying the data type as float, the numpy.asarray_chkfinite() function converts the integer values in a to floating-point numbers in the resulting NumPy array. The resulting NumPy array contains the same values as the input list a, but with the data type of the elements changed to float.
Pictorial Presentation:
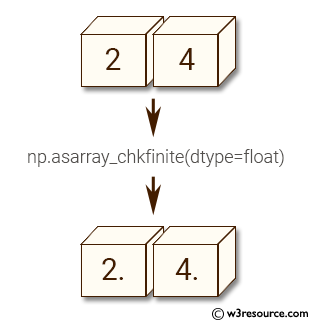
Example: Raises error using numpy.asarray_chkfinite
>>> import numpy as np
>>> a = [2, 4, np.inf]
>>> try:
... np.asarray_chkfinite(a)
... except ValueError:
... print('ValueError')
...
ValueError
In the above code, the list 'a' contains the infinite value np.inf. When np.asarray_chkfinite(a) is called, it raises a ValueError because of the presence of the infinite value. The try-except block is used to catch this error and print a message indicating that a ValueError was raised.
Python - NumPy Code Editor:
Previous: ascontiguousarray
Next: asscalar()