NumPy: numpy.atleast_3d() function
numpy.atleast_3d() function
The numpy.atleast_3d() function is used to ensure given inputs as arrays with at least three dimensions. If the input is a 1D or 2D array, it will add an additional axis at the end of the array. If the input is already a 3D or higher-dimensional array, then it returns the input array without any change.
This function is useful when you want to ensure that the input array has three dimensions, for example, when you want to pass a 3D array to a function that only accepts 3D arrays, or when you want to stack 2D arrays along a new axis.
Syntax:
numpy.atleast_3d(*arys)
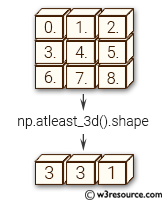
Parameter:
Name | Description | Required / Optional |
---|---|---|
arys1, arys2, . . . | One or more array-like sequences. Non-array inputs are converted to arrays. Arrays that already have three or more dimensions are preserved. |
Required |
Return value:
res1, res2, . . . [ndarray]
Example: Using numpy.atleast_3d() to ensure minimum 3 dimensions
>>> import numpy as np
>>> np.atleast_3d(6.0)
array([[[ 6.]]])
In the above code the numpy.atleast_3d() function ensures that a scalar value 6.0 is converted into an array of at least 3 dimensions. The resulting array is a 1x1x1 numpy array containing the value 6.0.
Pictorial Presentation:
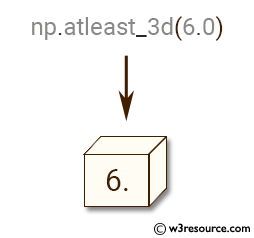
Example: Converting a 1D array to a 3D array using numpy.atleast_3d()
>>> import numpy as np
>>> y = np.arange(5.0)
>>> np.atleast_3d(y).shape
(1, 5, 1)
In the above code the input array 'y' is a 1D array, and np.atleast_3d(y) returns a 3D array with a shape of (1, 5, 1). The new dimensions are added to the array as singleton dimensions, i.e., dimensions with a size of 1. The original data is now present in the middle dimension of the resulting 3D array.
Pictorial Presentation:
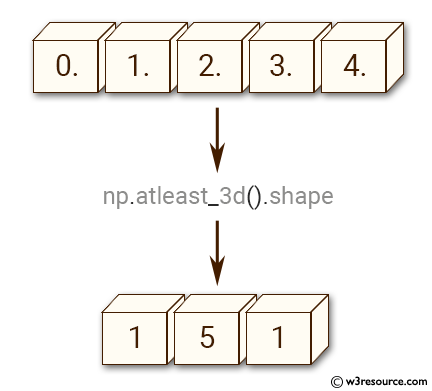
Example: Examples of numpy.atleast_3d() function
>>> import numpy as np
>>> y = np.arange(9.0).reshape(3,3)
>>> np.atleast_3d(y).shape
(3, 3, 1)
>>> np.atleast_3d(y).base is y.base
True
>>> for arr in np.atleast_3d([1,2],[[1,2]],[[[1,2]]]):
... print(arr, arr.shape)
...
[[[1]
[2]]] (1, 2, 1)
[[[1]
[2]]] (1, 2, 1)
[[[1 2]]] (1, 1, 2)
As shown in the above code first example, an array of shape (3,3) is passed as input to np.atleast_3d() function, and the resulting shape is (3,3,1).
In the second example, it is shown that the base of the output of np.atleast_3d() is the same as the base of the input.
In the third example, three input arrays of different shapes are passed to np.atleast_3d() function, and for each input array, a corresponding 3-dimensional array is returned with dimensions (1,2,1), (1,2,1), and (1,1,2) respectively.
Python - NumPy Code Editor:
Previous: arleast_2d()
Next: broadcast