NumPy: numpy.resize() function
numpy.resize() function
The numpy.resize() function is used to create a new array with the specified shape. If the new size is larger than the original size, the elements in the original array will be repeated to fill the new array.
The function can be useful in cases where you want to change the size of an array without creating a new array. For example, you may want to increase the size of an array to add new elements or decrease the size of an array to remove elements.
Syntax:
numpy.resize(a, new_shape)
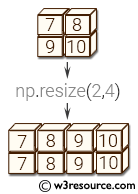
Parameters:
Name | Description | Required / Optional |
---|---|---|
a | Array to be resized. | Required |
new_shape | Shape of resized array. | Required |
Return value:
reshaped_array : ndarray - The new array is formed from the data in the old array, repeated if necessary to fill out the required number of elements. The data are repeated in the order that they are stored in memory.
Example: Resizing a NumPy array using numpy.resize()
>>> import numpy as np
>>> a = np.array([[1,2], [3,4]])
>>> np.resize(a, (3,2))
array([[1, 2],
[3, 4],
[1, 2]])
In the above code a NumPy array 'a' is created using the np.array() function with the values [[1,2], [3,4]]. Then, the np.resize() function is called with two arguments: the array a, and the new shape (3,2). This means that we want to resize the array a to have 3 rows and 2 columns. The resulting array has the values [[1, 2], [3, 4], [1, 2]].
Pictorial Presentation:
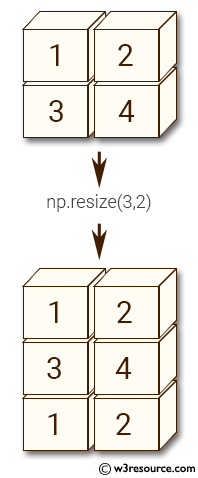
Example: Reshaping Array using numpy.resize() function
>>> import numpy as np
>>> a = np.array([[1,2], [3,4]])
>>> np.resize(a, (2,3))
array([[1, 2, 3],
[4, 1, 2]])
In the above code a 2D array 'a' has created with two rows and two columns. The np.resize() function returns a new array with the specified size. In this case, a new 2x3 array is returned, reshaped from the original array 'a'. As the new size is larger than the original array, the original array has repeated to fill the new array.
Pictorial Presentation:
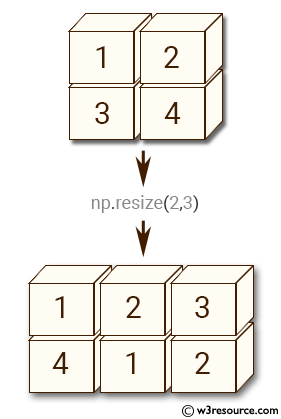
Example: Resizing a NumPy Array
>>> import numpy as np
>>> a = np.array([[1,2], [3,4]])
>>> np.resize(a, (2,4))
array([[1, 2, 3, 4],
[1, 2, 3, 4]])
In the above code-
First, a 2-dimensional NumPy array is created with the np.array() function and assigned to the variable a. This array has dimensions of 2 rows and 2 columns, and contains the values [1, 2] in the first row and [3, 4] in
the second row.
The np.resize() function is then used to resize this array to a new shape of (2,4). This means that the resulting array will have 2 rows and 4 columns.
Pictorial Presentation:
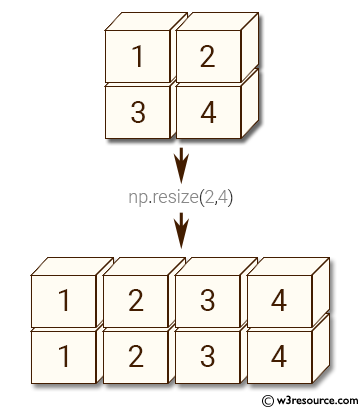
Python - NumPy Code Editor:
Previous: append()
Next: trim_zeros()