NumPy: numpy.ndarray.T function
numpy.ndarray.T function
The numpy.ndarray.T() function returns the transpose of a given array. The transpose of an array is obtained by interchanging its rows and columns.
This can be useful in machine learning applications where the input data needs to be in a certain format for further processing.
Syntax:
numpy.ndarray.T
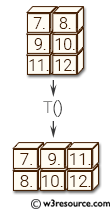
Example: Transposing a NumPy array using numpy.ndarray.T
>>> import numpy as np
>>> a = np.array([[2.,3.],[4.,5.]])
>>> a.T
array([[ 2., 4.],
[ 3., 5.]])
In the above code, a 2x2 NumPy array a is defined using the np.array() function. Then, the .T attribute is used to transpose the array. The transpose of the array a is a 2x2 array where the rows are swapped with columns. This means that the element at position (i, j) in the original array a is located at position (j, i) in the transposed array.
The .T attribute returns the transpose of a NumPy array as a new NumPy array without modifying the original array.
Pictorial Presentation:
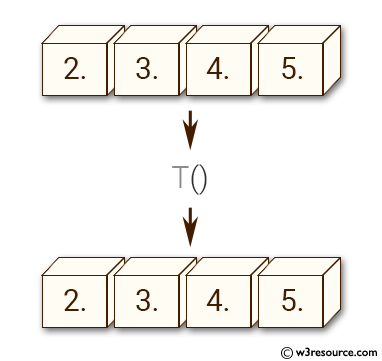
Example: Transposing a 1-D array using numpy.ndarray.T
>>> import numpy as np
>>> a = np.array([2.,3.,4.,5.])
>>> a
array([ 2., 3., 4., 5.])
>>> a.T
array([ 2., 3., 4., 5.])
The above code creates a one-dimensional numpy array a with the values [2., 3., 4., 5.]. Since a is a one-dimensional array, the .T attribute doesn't actually transpose anything, it just returns the original array. This is because transposing only has an effect on arrays with more than one dimension, and a one-dimensional array doesn't have multiple dimensions to swap. Therefore, the output of a.T is the same as the original array a.
Python - NumPy Code Editor:
Previous: swapaxes()
Next: transpose()
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/numpy/manipulation/ndarray-t.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics