NumPy: numpy.trim_zeros() function
numpy.trim_zeros() function
The numpy.trim_zeros() function is used to trim the leading and/or trailing zeros from a 1-D array or sequence.
The function can also be used to remove extra zeros from the beginning or end of an array, which may have been added to match the shape of other arrays.
Syntax:
numpy.trim_zeros(filt, trim='fb')
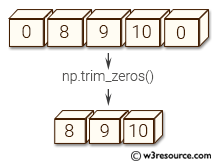
Parameter:
Name | Description | Required / Optional |
---|---|---|
filt | Input array. | Required |
trim | A string with 'f' representing trim from front and 'b' to trim from back. Default is 'fb', trim zeros from both front and back of the array. | Optional |
Return value:
trimmed : 1-D array or sequence The result of trimming the input. The input data type is preserved.
Example: Removing leading and trailing zeros from a NumPy array using np.trim_zeros()
>>> import numpy as np
>>> a = np.array((0,0,0,1,1,2,2,3,0,3))
>>> np.trim_zeros(a)
array([1, 1, 2, 2, 3, 0, 3])
In the above code, the original array 'a' contains some leading zeros, some trailing zeros, and some non-zero elements in between. The np.trim_zeros() function removes the leading and trailing zeros and returns a new array with the remaining elements.
Pictorial Presentation:
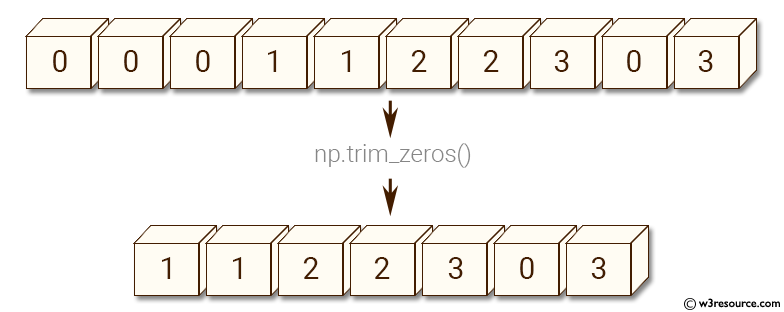
Example: Removing Trailing Zeros from a NumPy Array using numpy.trim_zeros()
>>> import numpy as np
>>> a = np.array((0,0,0,1,1,2,2,3,0,3))
>>> np.trim_zeros(a, 'b')
array([0, 0, 0, 1, 1, 2, 2, 3, 0, 3])
A NumPy array 'a' is defined with the values (0, 0, 0, 1, 1, 2, 2, 3, 0, 3). The numpy.trim_zeros() function is then applied on this array with the argument 'b', which indicates that trailing zeros should be removed.
The resulting array after applying the function is [0, 0, 0, 1, 1, 2, 2, 3, 0, 3]. Since the 'b' argument was passed, only the trailing zeros are removed from the array.
Pictorial Presentation:
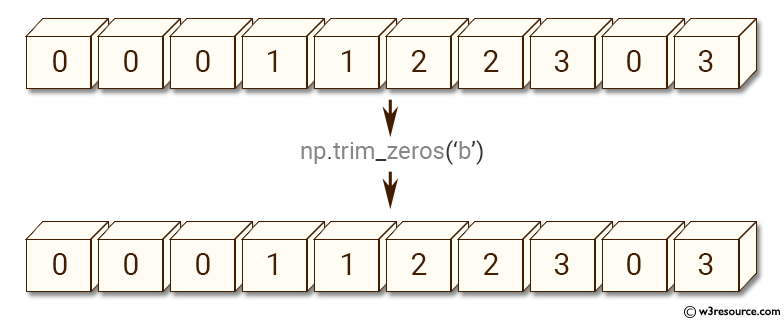
Example: Removing leading and trailing zeros from a 1-D array using numpy.trim_zeros()
>>> import numpy as np
>>> np.trim_zeros([0, 0, 1, 2, 3, 0, 0])
[1, 2, 3]
In the code, we first define a 1-D array [0, 0, 1, 2, 3, 0, 0] and pass it as an argument to numpy.trim_zeros() function. The function returns a new array [1, 2, 3] which contains only the non-zero elements of the input array.
Pictorial Presentation:
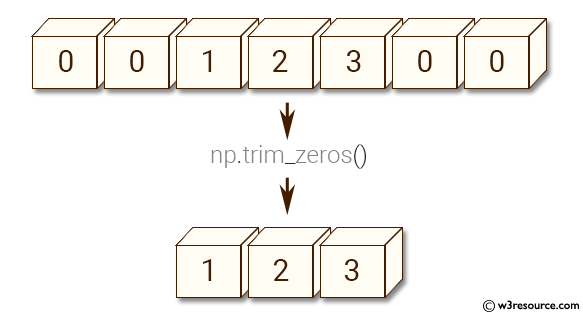
Python - NumPy Code Editor: