NumPy: numpy.rollaxis() function
numpy.rollaxis() function
The numpy.rollaxis() function is used to roll the specified axis backwards, until it lies in a given position. This function does not change the order of the other axes.
The function is particularly useful for reshaping multi-dimensional arrays to a desired configuration. The application of numpy.rollaxis() function includes data reshaping, array broadcasting, and manipulation of multi-dimensional data. It allows for efficient and concise manipulation of multi-dimensional arrays, making it a useful tool for data analysis and scientific computing.
Syntax:
numpy.rollaxis(a, source, destination)
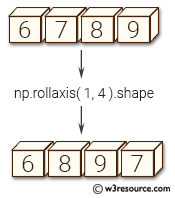
Parameters:
Name | Description | Required / Optional |
---|---|---|
a | Input array. | Required |
axis | The axis to roll backwards. The positions of the other axes do not change relative to one another. | Required |
start | The axis is rolled until it lies before this position. The default, 0, results in a "complete" roll. | Optional |
Return value:
res [ndarray] For NumPy >= 1.10.0 a view of a is always returned. For earlier NumPy versions a view of a is returned only if the order of the axes is changed, otherwise the input array is returned.
Example: Rearranging axes using numpy.rollaxis()
>>> import numpy as np
>>> a = np.ones((2,3, 4, 5))
>>> np.rollaxis(a, 2, 1).shape
(2, 4, 3, 5)
In the above code, a 4-dimensional numpy array "a" is created using the np.ones() function. The shape of "a" is (2, 3, 4, 5), which means it has 2 elements along the first axis, 3 elements along the second axis, 4 elements along the third axis, and 5 elements along the fourth axis. Each element is set to 1.
In the next line, the np.rollaxis() function is used to move the third axis of "a" to the second position. This means the shape of the resulting array will be (2, 4, 3, 5) instead of (2, 3, 4, 5).
Pictorial Presentation:
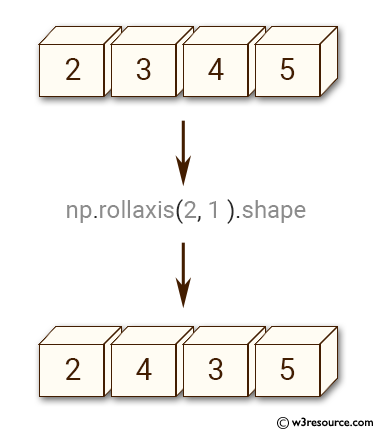
Example: Transposing an array using numpy.rollaxis()
>>> import numpy as np
>>> y = np.ones((2,3, 4, 5))
>>> np.rollaxis(y, 3).shape
(5, 2, 3, 4)
In the above code, a NumPy array 'y' is created with the shape (2, 3, 4, 5) using the np.ones() function. The np.rollaxis() function is then called with y and the parameter 3, which specifies the axis to be moved to the front. The resulting array has a shape of (5, 2, 3, 4), indicating that the axis at index 3 (i.e., the last axis) has been moved to the front, becoming the first axis.
Pictorial Presentation:
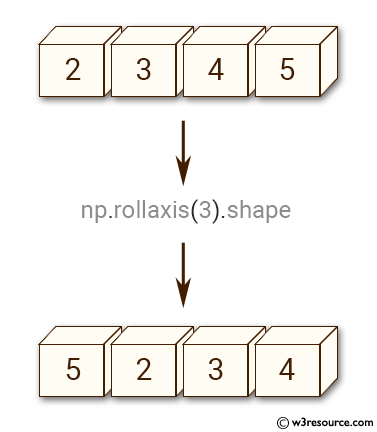
Example: Re-arranging the axes of a numpy array using numpy.rollaxis()
>>> import numpy as np
>>> y = np.ones((2,3, 4, 5))
>>> np.rollaxis(y, 1, 3).shape
(2, 4, 3, 5)
In the above code numpy.rollaxis() function is used to re-arrange the axes of the array. The first argument of the function is the array 'y' that we want to modify, and the second argument specifies the source axis that we want to move. The third argument specifies the destination axis to which we want to move the source axis.
Here, we are moving the third axis (of shape 4) to the second position (i.e., the destination axis is 1), and leaving the other axes unchanged. As a result, the shape of the modified array becomes (2, 4, 3, 5).
Finally, we check the new shape of the modified array, which is (2, 4, 3, 5).
Pictorial Presentation:
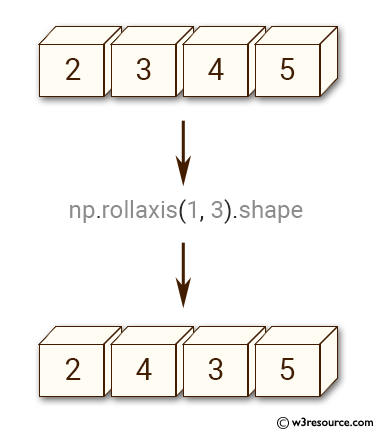
Python - NumPy Code Editor:
Previous: moveaxis()
Next: swapaxes()