NumPy: numpy.block() function
numpy.block() function
The numpy.block() function assembles an nd-array from nested lists of blocks.
Blocks in the innermost lists are concatenated along the last dimension (-1), then these are concatenated along the second-last dimension (-2), and so on until the outermost list is reached.
This function can be used to create more complex arrays from simpler ones. For example, it can be used to create block matrices from smaller matrices, concatenate a mix of 1-D and 2-D arrays, or create 3-D arrays by stacking 2-D arrays along a new axis.
Syntax:
numpy.block(arrays)
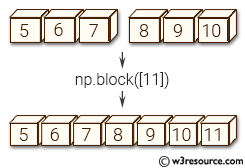
Parameter:
Name | Description | Required / Optional |
---|---|---|
arrays | If passed a single ndarray or scalar (a nested list of depth 0), this is returned unmodified (and not copied). Elements shapes must match along the appropriate axes (without broadcasting), but leading 1s will be prepended to the shape as necessary to make the dimensions match. |
Required |
Return value:
block_array : ndarray
The array assembled from the given blocks.
The dimensionality of the output is equal to the greatest of: * the dimensionality of all the inputs * the depth to which the input list is nested
Raises: ValueError -
- If list depths are mismatched - for instance, [[a, b], c] is illegal, and should be spelt [[a, b], [c]]
- If lists are empty - for instance, [[a, b], []]
Example-1: Combining arrays using numpy.block()
import numpy as np
>>> A = np.eye(2) * 2
>>> B = np.eye(3) * 3
>>> np.block([
... [A, np.zeros((2, 3))],
... [np.ones((3, 2)), B ]
... ])
array([[ 2., 0., 0., 0., 0.],
[ 0., 2., 0., 0., 0.],
[ 1., 1., 3., 0., 0.],
[ 1., 1., 0., 3., 0.],
[ 1., 1., 0., 0., 3.]])
In the above code Array A is a 2x2 identity matrix scaled by 2, while array B is a 3x3 identity matrix scaled by 3.
The np.block() function takes in a list of arrays and combines them according to a specified block structure. Here, a block structure is created using zeros and ones arrays to create a 5x5 matrix C.
Array A is placed in the top left corner of C, while a 2x3 zeros array is placed to the right of A. Array B is placed in the bottom right corner of C, while a 3x2 ones array is placed above B.
The resulting array C has the dimensions 5x5 and is a combination of A and B.
Example-2: Combining arrays using numpy.block()
import numpy as np
>>> np.block([1, 2, 3])
array([1, 2, 3])
>>> a = np.array([1, 2, 3])
>>> b = np.array([2, 3, 4])
>>> np.block([a, b, 10])
array([1, 2, 3, 2, 3, 4, 10])
In the above code the numpy.block() function is used to assemble arrays from smaller subarrays. In the first example, we have a simple array [1,2,3], which can be created using the np.block() function with the input [1,2,3]. This code simply stacks the input arrays horizontally.
In the second example, we have two arrays 'a' and 'b' with elements [1,2,3] and [2,3,4] respectively. By using the np.block() function with input [a,b,10], we can stack both arrays 'a' and 'b' horizontally, and append a scalar value '10' to the end of the array. This results in the array [1,2,3,2,3,4,10].
Pictorial Presentation:
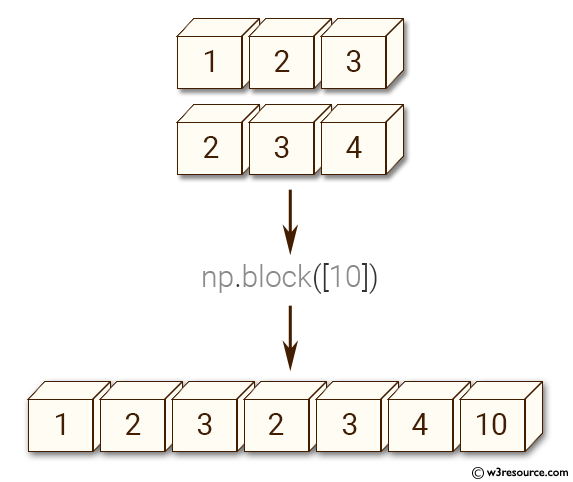
Python - NumPy Code Editor:
Previous: vstack()
Next: Splitting arrays split()