NumPy: numpy.squeeze() function
numpy.squeeze() function
The numpy.squeeze() function is used to remove single-dimensional entries from the shape of an array. It returns an array with the same data but reshaped dimensions.
This function can be used to reduce the dimensions of an array, which is useful for data preprocessing in machine learning applications. For example, if we have an image dataset where each image is represented as a 3D array with dimensions (height, width, channels), we can use squeeze() to remove any single-dimensional entries, such as when there is only one channel for a grayscale image.
Syntax:
numpy.squeeze(a, axis=None)
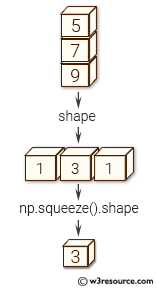
Parameters:
Name | Description | Required / Optional |
---|---|---|
a | Input data. | Required |
axis | Selects a subset of the single-dimensional entries in the shape. If an axis is selected with shape entry greater than one, an error is raised. | Optional |
Return value:
squeezed [ndarray] The input array, but with all or a subset of the dimensions of length 1 removed. This is always a itself or a view into a.
Raises: ValueError - If axis is not None, and an axis being squeezed is not of length 1
Example: Removing single-dimensional entries using numpy.squeeze()
>>> import numpy as np
>>> a = np.array([[[0], [2], [4]]])
>>> a.shape
(1, 3, 1)
>>> np.squeeze(a).shape
(3,)
In the above code, a numpy array 'a' is defined with shape (1, 3, 1), which has one element in the first dimension, three elements in the second dimension and one element in the third dimension. Since there is only one element in the first and third dimensions, np.squeeze() removes them, and the shape of the resulting array is (3,).
Pictorial Presentation:
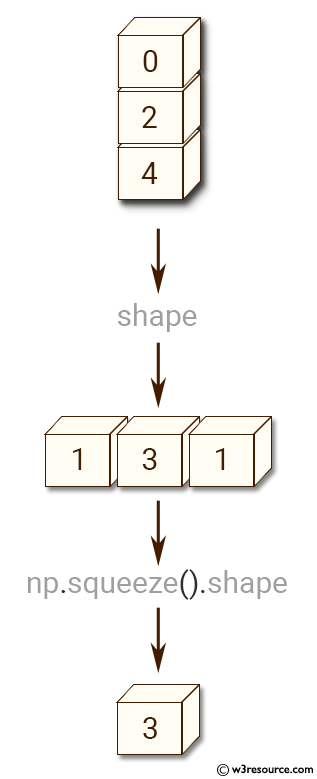
Example: Using numpy.squeeze() function with axis parameter
>>> import numpy as np
>>> a = np.array([[[0], [2], [4]]])
>>> np.squeeze(a, axis=0).shape
(3, 1)
>>> np.squeeze(a, axis=1).shape
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "/usr/local/lib/python3.6/dist-packages/numpy/core/fromnumeric.py", line 1201, in squeeze
return squeeze(axis=axis)
ValueError: cannot select an axis to squeeze out which has size not equal to one
>>> np.squeeze(a, axis=2).shape
(1, 3)
In the above code, the numpy.squeeze() function is used to remove dimensions of size 1 from the input array 'a'. The original array 'a' has a shape of (1, 3, 1).
- First, the np.squeeze(a, axis=0) removes the dimension of size 1 at index 0 and returns a new array with shape (3, 1).
- When np.squeeze(a, axis=1) is called, it raises a ValueError as the axis=1 has size not equal to one and cannot be removed.
- Finally, np.squeeze(a, axis=2) removes the dimension of size 1 at index 2 and returns a new array with shape (1, 3).
Python - NumPy Code Editor:
Previous: expand_dims()
Next: Changing kind of array asarray()