NumPy: numpy.vsplit() function
numpy.vsplit() function
The numpy.vsplit() function is used to split an array into multiple sub-arrays vertically (row-wise). vsplit() is equivalent to split with axis=0 (default), the array is always split along the first axis regardless of the array dimension.
The numpy.vsplit() function is useful when dealing with large datasets that need to be processed in parallel. By dividing the dataset into smaller chunks, multiple processors can work on different parts of the dataset at the same time, thus reducing processing time.
Syntax:
numpy.vsplit(ary, indices_or_sections)
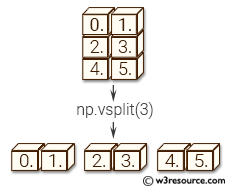
Parameters:
Name | Description | Required / Optional |
---|---|---|
ary | Input array. | Required |
indices_or_sections | Indices or sections. | Required |
Return value:
Example: Splitting numpy array vertically using numpy.vsplit()
>>> import numpy as np
>>> a = np.arange(20.0).reshape(4,5)
>>> a
array([[ 0., 1., 2., 3., 4.],
[ 5., 6., 7., 8., 9.],
[ 10., 11., 12., 13., 14.],
[ 15., 16., 17., 18., 19.]])
>>> np.vsplit(a, 2)
[array([[ 0., 1., 2., 3., 4.],
[ 5., 6., 7., 8., 9.]]), array([[ 10., 11., 12., 13., 14.],
[ 15., 16., 17., 18., 19.]])]
In the above code, we first create a 4x5 numpy array 'a' using the arange() and reshape() functions. We then use the vsplit() function to split the array 'a' into two subarrays along the vertical axis. The result is a list containing two subarrays, each of which contains half of the rows from the original array 'a'.
Pictorial Presentation:
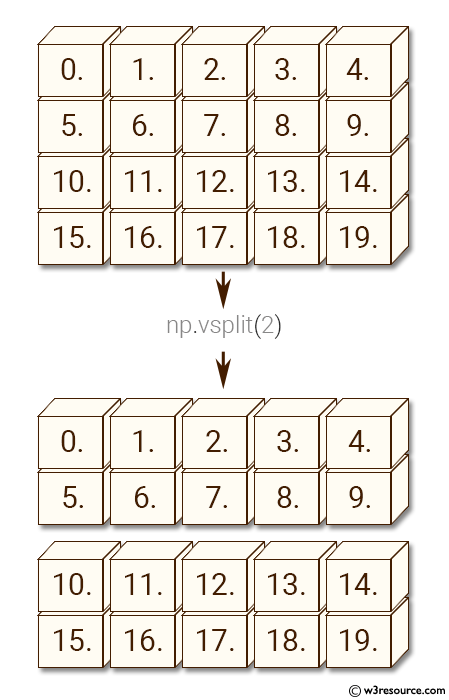
Example: Splitting a NumPy array vertically using a specified array of indices
>>> import numpy as np
>>> np.vsplit(a, np.array([2, 5]))
[array([[ 0., 1., 2., 3., 4.],
[ 5., 6., 7., 8., 9.]]), array([[ 10., 11., 12., 13., 14.],
[ 15., 16., 17., 18., 19.]]), array([], shape=(0, 5), dtype=float64)]
In the above code, we have a 4x5 NumPy array a containing numbers from 0 to 19. We then pass an array of indices [2, 5] to the np.vsplit() function.
The np.vsplit() function then splits the array a into three arrays vertically based on the specified indices:
- The first array includes rows 0 and 1 of the original array.
- The second array includes rows 2 and 3 of the original array.
- The third array is an empty array because there are no rows between indices 5 and the end of the array.
Example: Splitting a 3D numpy array vertically using numpy.vsplit()
>>> import numpy as np
>>> a = np.arange(12.0).reshape(2,3,2)
>>> a
array([[[ 0., 1.],
[ 2., 3.],
[ 4., 5.]],
[[ 6., 7.],
[ 8., 9.],
[ 10., 11.]]])
>>> np.vsplit(a, 2)
[array([[[ 0., 1.],
[ 2., 3.],
[ 4., 5.]]]), array([[[ 6., 7.],
[ 8., 9.],
[ 10., 11.]]])]
In the above code, a 3D NumPy array "a" is created using the np.arange() function and the np.reshape() function, which reshapes the array into a 2x3x2 matrix.
Then, the np.vsplit() function is used to split the array vertically into two equal parts. Since the array has a shape of (2,3,2), it is split vertically along the first axis (axis=0), resulting in two arrays, each with a shape of (1,3,2).
Python - NumPy Code Editor:
Previous: hsplit()
Next: Tiling arrays tile()