NumPy: numpy.array_split() function
numpy.array_split() function
The numpy.array_split() function split an given array into multiple sub-arrays.
The only difference between these functions is that array_split allows indices_or_sections to be an integer that does not equally divide the axis. For an array of length l that should be split into n sections, it returns l % n sub-arrays of size l//n + 1 and the rest of size l//n.
One common application of numpy.array_split() is when you have a large dataset that you want to split into smaller, more manageable pieces for processing or analysis.
Syntax:
numpy.array_split(ary, indices_or_sections, axis=0)
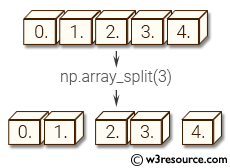
Parameters:
Name | Description | Required / Optional |
---|---|---|
ary | Input array. | Required |
indices_or_sections | Indices or sections. | Required |
axis | The axis along which values are appended. If axis is not given, both arr and values are flattened before use. | Optional |
Return value:
Example: Splitting a NumPy array into equal-sized subarrays using numpy.array_split()
>>> import numpy as np
>>> a = np.arange(9.0)
>>> np.array_split(a, 4)
[array([ 0., 1., 2.]), array([ 3., 4.]), array([ 5., 6.]), array([ 7., 8.])]
In the above code, the array 'a' is created using np.arange() function and it contains 9 elements. The np.array_split() function is then called with the array a and the number of subarrays to be created as parameters. Here, the number of subarrays is 4. The resulting subarrays are returned as a list of NumPy arrays.
Pictorial Presentation:
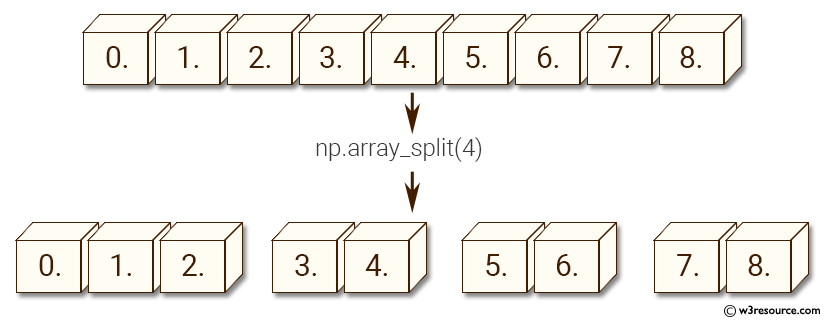
Example: Splitting a numpy array into multiple sub-arrays using numpy.array_split()
>>> import numpy as np
>>> a = np.arange(7.0)
>>> np.array_split(a, 3)
[array([ 0., 1., 2.]), array([ 3., 4.]), array([ 5., 6.])]
In the above code numpy.array_split() function splits a one-dimensional numpy array a into multiple sub-arrays of equal or nearly-equal size. In this case, the array a is created using np.arange(7.0), which generates a sequence of numbers from 0 to 6.
Pictorial Presentation:
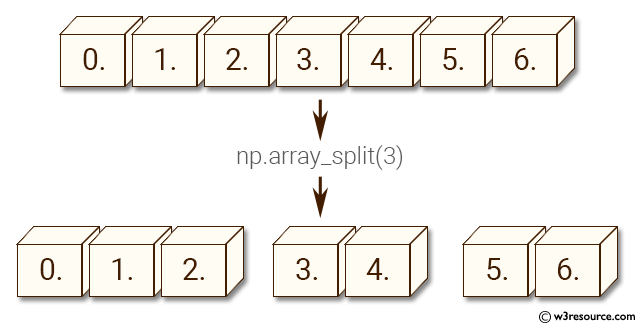
Python - NumPy Code Editor: