NumPy: numpy.repeat() function
numpy.repeat() function
The numpy.repeat() function is used to repeat elements of an array. It takes an array and a repetition count as inputs and outputs a new array with the original array elements repeated based on the repetition count.
The function is useful in data processing, scientific computing and image processing, where the repeated elements can be used in feature extraction, data augmentation.
Syntax:
numpy.repeat(a, repeats, axis=None)
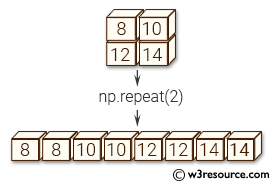
Parameters:
Name | Description | Required / Optional |
---|---|---|
a | Input array. | Required |
repeats | The number of repetitions for each element. 'repeats' is broadcasted to fit the shape of the given axis. | Required |
axis | The axis along which to repeat values. By default, the function uses the flattened input array and returns a flat output array. | Optional |
Return value:
repeated_array [ndarray]: Output array that has the same shape as a, except along the specified axis.
Example: Repeating a scalar using numpy.repeat()
>>> import numpy as np
>>> np.repeat(5,3)
array([5, 5, 5])
In the above code, the numpy.repeat() function is used to repeat the scalar value 5, three times. The first argument of the function is the value to be repeated and the second argument is the number of times to repeat the value.
Visual Presentation:
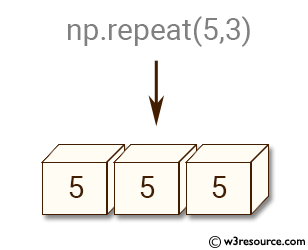
Example: Repeating elements of an array using numpy.repeat()
>>> import numpy as np
>>> a = np.array([[3,5], [7,9]])
>>> np.repeat(a, 4)
array([3, 3, 3, 3, 5, 5, 5, 5, 7, 7, 7, 7, 9, 9, 9, 9])
>>> np.repeat(a, 4, axis=1)
array([[3, 3, 3, 3, 5, 5, 5, 5],
[7, 7, 7, 7, 9, 9, 9, 9]])
In the first example, a 2-dimensional array 'a' is created using numpy.array() function. The np.repeat() function is then applied on the array a with repeat count 4, which results in each element of a being repeated 4 times, producing a 1-dimensional array of length 16.
In the second example, the same array 'a' is used, but this time the np.repeat() function is applied with repeat count 4 along axis=1. This results in each element of a being repeated 4 times along the columns (i.e. horizontally) and the resulting array is 2-dimensional with the same number of rows and 4 times the number of columns.
Visual Presentation:
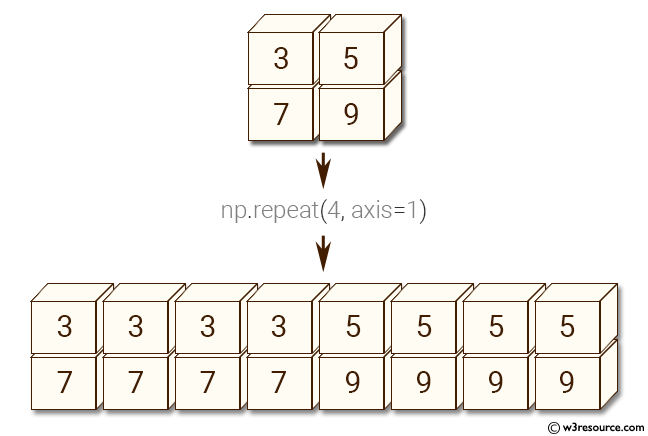
Example: Repeating Rows in Numpy Arrays
>>> import numpy as np
>>> a = np.array([[3,5], [7,9]])
>>> np.repeat(a, [1,3], axis=0)
array([[3, 5],
[7, 9],
[7, 9],
[7, 9]])
In the above code, a 2x2 NumPy array a is defined with values [[3,5], [7,9]]. The np.repeat() function is then used to repeat the rows of a, with the argument [1,3] indicating that the first row should be repeated once, and the second row should be repeated three times.
Visual Presentation:
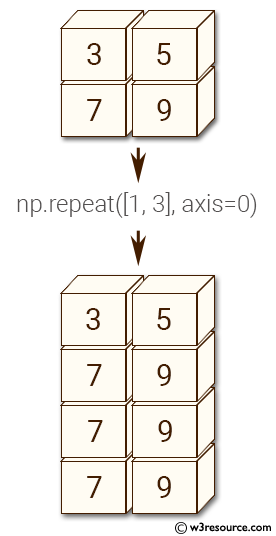
Example: Repeating Elements Along Multiple Axes
import numpy as np
a = np.array([[1, 2], [3, 4]])
print(np.repeat(a, [2, 3], axis=0))
print(np.repeat(a, [2, 3], axis=1))
Output for axis=0:
[[1 2] [1 2] [3 4] [3 4] [3 4]]
Output for axis=1:
[[1 1 2 2 2] [3 3 4 4 4]]
This example demonstrates how to repeat elements along different axes, showing the flexibility of the repeats parameter when working with multi-dimensional arrays.
Frequently Asked Questions (FAQ): numpy. repeat () Function
1. What is the primary use of the numpy.repeat() function?
The numpy.repeat() function is primarily used to repeat elements of an array, creating a new array with repeated values based on the specified repetition count.
2. In what contexts is numpy.repeat() particularly useful?
numpy.repeat() is especially useful in data processing, scientific computing, and image processing for tasks such as feature extraction and data augmentation.
3. How does numpy.repeat() handle the input array?
numpy.repeat() takes the input array and repeats its elements according to the repetition count provided. The repetition can be applied along a specified axis or to the flattened array if no axis is specified.
4. Can numpy.repeat() repeat elements along a specific axis?
Yes, numpy.repeat() can repeat elements along a specified axis. If no axis is provided, it repeats the elements in the flattened input array.
5. Does numpy.repeat() modify the original array?
No, numpy.repeat() does not modify the original array. It returns a new array with repeated elements.
6. What type of array does numpy.repeat() return?
numpy.repeat() returns an ndarray with the same type as the input array but with a modified shape based on the specified repetitions and axis.
7. Can numpy.repeat() handle multi-dimensional arrays?
Yes, numpy.repeat() can handle multi-dimensional arrays, repeating elements along specified axes or across the entire array if no axis is specified.
Python - NumPy Code Editor:
Previous: Tiling arrays tile()
Next: Adding and removing elements delete()