NumPy: numpy.roll() function
numpy.roll() function
The numpy.roll() function is used to roll array elements along a given axis. Elements that roll beyond the last position are re-introduced at the first.
One application of numpy.roll() is in signal processing, where it can be used to shift a signal in time. In image processing, it can be used to shift an image along an axis, for example to correct for camera motion or to align images for image stitching. It can also be used in numerical simulations, where it may be necessary to shift the data along a particular axis for calculations or data analysis.
Syntax:
numpy.roll(a, shift, axis=None)
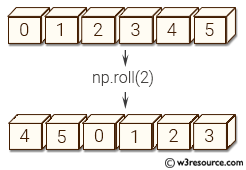
Parameters:
Name | Description | Required / Optional |
---|---|---|
a | Input array. | Required |
shift | The number of places by which elements are shifted. If a tuple, then axis must be a tuple of the same size, and each of the given axes is shifted by the corresponding number. If an int while axis is a tuple of ints, then the same value is used for all given axes. | Required |
axis | Axis or axes along which elements are shifted. By default, the array is flattened before shifting, after which the original shape is restored. | Optional |
Return value:
res : ndarray - Output array, with the same shape as a.
Example: Rolling an array along a given axis
>>> import numpy as np
>>> a = np.arange(8)
>>> np.roll(a, 3)
array([5, 6, 7, 0, 1, 2, 3, 4])
In the above code, a one-dimensional numpy array 'a' is first created using the arange() function to generate the numbers 0 to 7. The numpy.roll() function is then called with 'a' as the input array and 3 as the second argument. This rolls the elements of 'a' by 3 positions to the right along the first axis (axis=0), resulting in the output array [5, 6, 7, 0, 1, 2, 3, 4].
Pictorial Presentation:
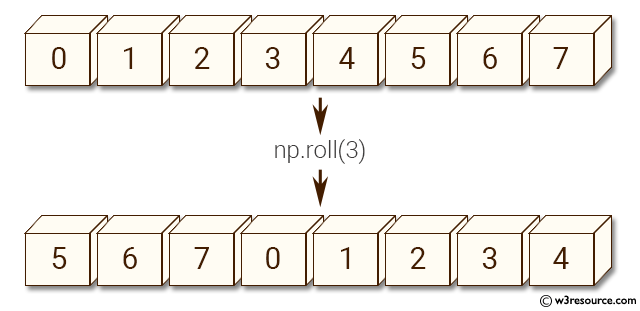
Example: Rolling a 2D NumPy array along an axis
>>> import numpy as np
>>> a = np.arange(8)
>>> b = np.reshape(a, (2, 4))
>>> b
array([[0, 1, 2, 3],
[4, 5, 6, 7]])
>>> np.roll(b, 1)
array([[7, 0, 1, 2],
[3, 4, 5, 6]])
In the above code first, an array a is created using the np.arange() function, which contains the numbers 0 through 7. Then, the a array is reshaped into a 2D array b with 2 rows and 4 columns using the np.reshape() function.
Next, the np.roll() function is used to roll the b array along the axis 1 (columns) by a shift of 1. In the rolled array, the elements from the last column have "rolled over" to the first column, and all other elements have shifted one position to the right.
Pictorial Presentation:
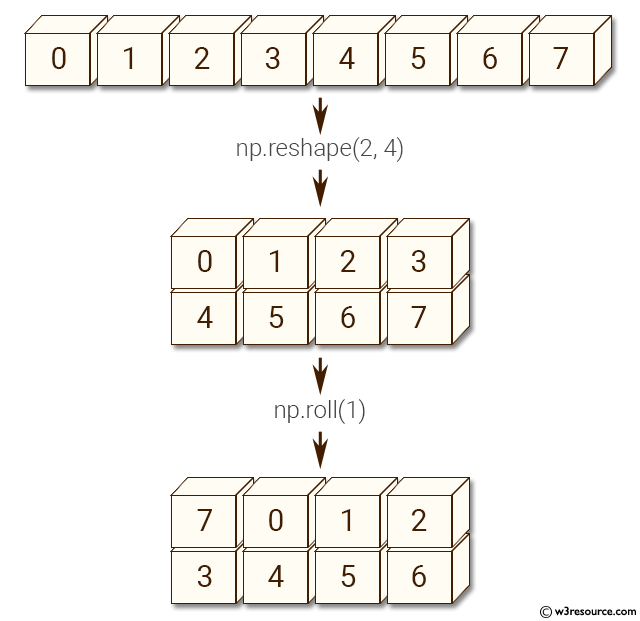
Example: Rolling a numpy array along a specific axis using numpy.roll()
>>> import numpy as np
>>> a = np.arange(8)
>>> b = np.reshape(a, (2, 4))
>>> np.roll(b, 1, axis=0)
array([[4, 5, 6, 7],
[0, 1, 2, 3]])
In the above code, first, a one-dimensional numpy array 'a' is created using np.arange() function with values from 0 to 7. Then, this array is reshaped into a 2x4 array 'b' using np.reshape() function.
Next, the numpy.roll() function is applied to array 'b' with a shift of 1 along the axis=0, i.e., the rows are rolled downwards by one.
Pictorial Presentation:
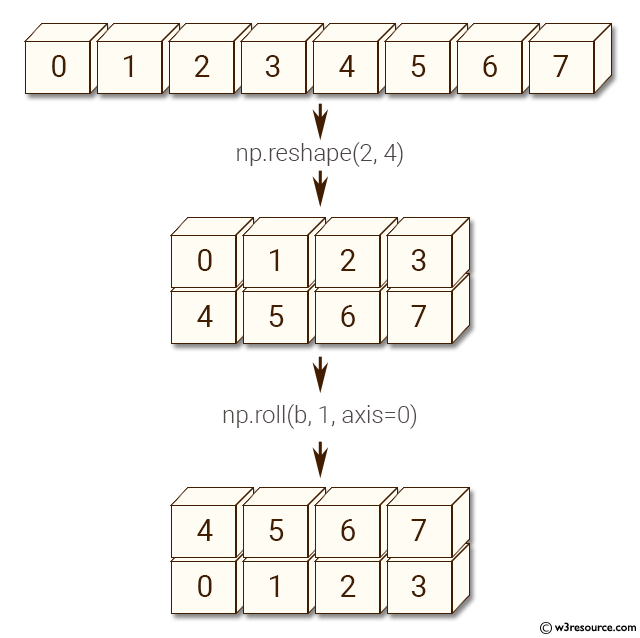
Example: Rolling an array in a specific axis using numpy.roll()
>>> import numpy as np
>>> a = np.arange(8)
>>> b = np.reshape(a, (2, 4))
>>> np.roll(b, 1, axis=1)
array([[3, 0, 1, 2],
[7, 4, 5, 6]])
In the above code, first example, np.roll(b, 1) is used to roll the elements of the b array one position to the right along the flattened array, resulting in the array where the last element is moved to the first position.
In the second example, np.roll(b, 1, axis=0) is used to roll the elements of the b array one position along the 0-th axis, i.e., the rows. This results in the array where each row is shifted down by one position, and the last row becomes the first row.
Similarly, in the third example, np.roll(b, 1, axis=1) is used to roll the elements of the b array one position along the 1st axis, i.e., the columns.
Pictorial Presentation:
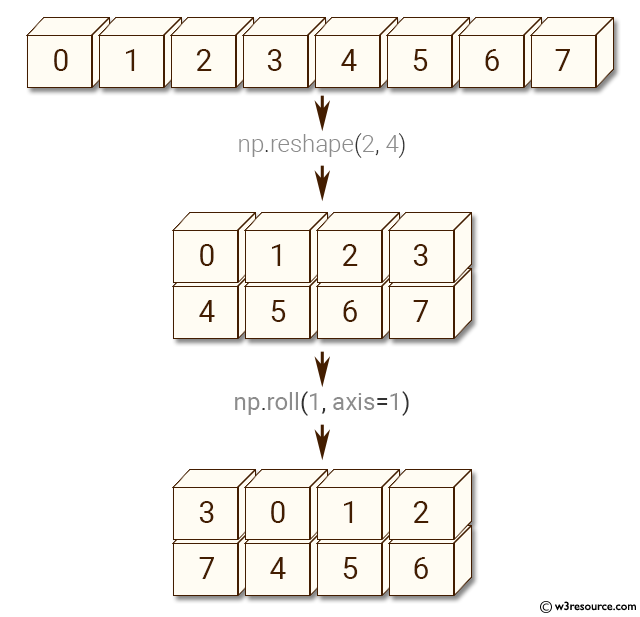
Python - NumPy Code Editor:
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics