NumPy: numpy.hstack() function
numpy.hstack() function
The numpy.hstack() function is used to stack arrays in sequence horizontally (column wise).
This is equivalent to concatenation along the second axis, except for 1-D arrays where it concatenates along the first axis. Rebuilds arrays divided by hsplit.
This function is useful in the scenarios when we have to concatenate two arrays of different shapes along the second axis (column-wise). For example, to combine two arrays of shape (n, m) and (n, l) to form an array of shape (n, m+l).
Syntax:
numpy.hstack(tup)
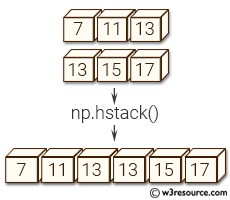
Parameter:
Name | Description | Required / Optional |
---|---|---|
tup | The arrays must have the same shape along all but the second axis, except for 1-D arrays which can be any length. | Required |
Return value:
stacked : ndarray
The array formed by stacking the given arrays.
Example 1: Horizontally Stacking 1-D Arrays
import numpy as np
x = np.array([3, 5, 7])
y = np.array([5, 7, 9])
print(np.hstack((x, y)))
Output:
[3 5 7 5 7 9]
In this example, two one-dimensional NumPy arrays x and y, each with three elements, are stacked horizontally. The resulting array contains all elements of x and y in a single one-dimensional array.
Visual Presentation:
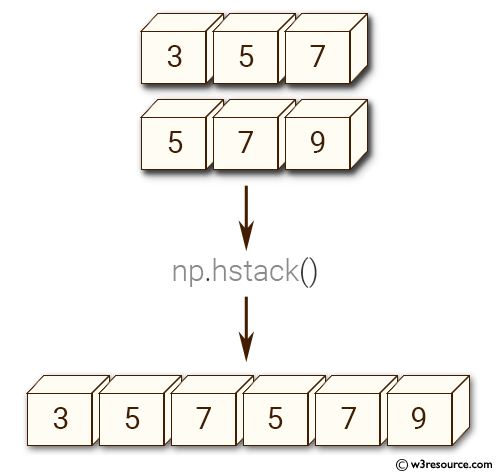
Example 2: Horizontally Stacking 2-D Arrays
import numpy as np
x = np.array([[3], [5], [7]])
y = np.array([[5], [7], [9]])
print(np.hstack((x, y)))
Output:
[[3 5] [5 7] [7 9]]
This example demonstrates horizontally stacking two 2-D arrays. The array x is a column vector of shape (3, 1) and y is also a column vector of the same shape. The numpy.hstack() function combines them into a new 2-D array of shape (3, 2).
Visual Presentation:
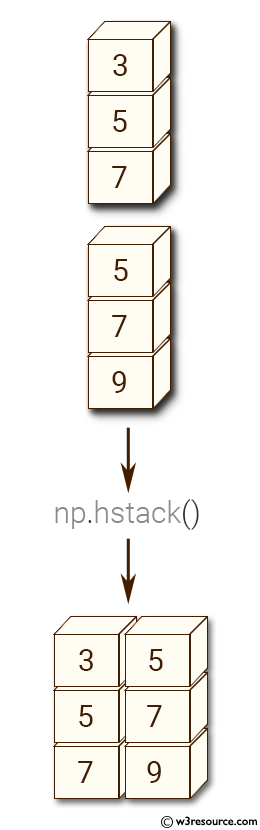
Example 3: Combining Arrays with Different Number of Columns
import numpy as np
a = np.array([[1, 2], [3, 4]])
b = np.array([[5, 6, 7], [8, 9, 10]])
print(np.hstack((a, b)))
Output:
[[ 1 2 5 6 7] [ 3 4 8 9 10]]
In this example, arrays a and b have different numbers of columns. The numpy.hstack() function combines them horizontally into a single array with a shape (2, 5).
Example 4: Stacking 1-D and 2-D Arrays
import numpy as np
x = np.array([1, 2, 3])
y = np.array([[4], [5], [6]])
print(np.hstack((x[:, np.newaxis], y)))
Output:
[[1 4] [2 5] [3 6]]
This example demonstrates how to stack a 1-D array with a 2-D array. The 1-D array x is first converted to a 2-D column vector using np.newaxis, and then stacked with y horizontally.
Frequently Asked Questions (FAQ): numpy. hstack() Function
1. What does the numpy.hstack() function do?
The numpy.hstack() function stacks arrays horizontally (column-wise).
2. In what scenarios is numpy.hstack() useful?
It is useful for concatenating arrays with the same shape along all but the second axis, particularly when combining arrays of different shapes along the column-wise axis.
3. Can numpy.hstack() handle 1-D arrays?
Yes, for 1-D arrays, it concatenates along the first axis.
4. What type of array does numpy.hstack() return?
It returns an ndarray formed by stacking the input arrays horizontally.
5. Do the input arrays need to have the same shape?
The input arrays must have the same shape along all but the second axis. For 1-D arrays, they can be of any length.
Python - NumPy Code Editor: