NumPy: numpy.expand_dims() function
numpy.expand_dims() function
The numpy.expand_dims() function is used to expand the shape of an array. Insert a new axis that will appear at the axis position in the expanded array shape.
This can be useful when we want to perform operations or calculations that require a specific shape or dimensionality of arrays. For example, if we have a 1-dimensional array, and we want to perform matrix multiplication with a 2-dimensional array, we can use expand_dims() function to add a new axis to the 1-dimensional array to make it a 2-dimensional array before performing the multiplication. Another example is in image processing where we can use expand_dims() to add a new axis to a grayscale image to make it a 3-dimensional image, which is required for certain image processing operations.
Syntax:
numpy.expand_dims(a, axis)
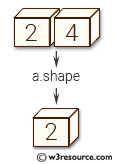
Parameters:
Name | Description | Required / Optional |
---|---|---|
a | Input array. | Required |
subok | IPosition in the expanded axes where the new axis is placed. | Required |
Return value:
res [ndarray] Output array. The number of dimensions is one greater than that of the input array.
Example: Using numpy.expand_dims() function to increase the dimensionality of an array
>>> import numpy as np
>>> a = np.array([2, 4])
>>> b = np.expand_dims(a, axis=0)
>>> b
array([[2, 4]])
>>> b.shape
(1, 2)
In the above code the numpy array "a" is defined as [2, 4]. The numpy.expand_dims() function is then called, which takes two arguments: the array to be expanded (in this case "a"), and the axis along which to expand the array.
Here, axis=0 is specified, which means that the dimension of the array will be increased along the rows. This results in a new array "b" with shape (1,2). The original elements of "a" are now stored within this new 2D array.
Thus, the output of the code is the new array "b" with shape (1,2), which contains the same elements as "a" but with an additional dimension.
Pictorial Presentation:
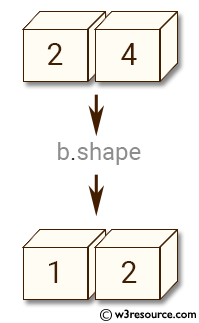
Example: Using numpy.expand_dims() to increase the dimensions of an array
>>> import numpy as np
>>> b = np.expand_dims(a, axis=1) # Equivalent to x[:,np.newaxis]
>>> b
array([[2],
[4]])
>>> b.shape
(2, 1)
In the above code, the numpy expand_dims() function is used to increase the dimensions of an array. The function takes two arguments, the first argument is the array that we want to expand, and the second argument specifies the axis along which we want to expand the array. In this case, the axis parameter is set to 1, which means that the new dimension will be inserted as a column, i.e., the shape of the new array will be (2, 1).
Pictorial Presentation:
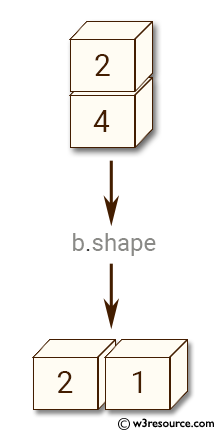
Python - NumPy Code Editor:
Previous: broadcast_arrays()
Next: squeeze()