NumPy: numpy.reshape() function
numpy.reshape() function
The numpy.reshape() function is used to change the shape (dimensions) of an array without changing its data. This function returns a new array with the same data but with a different shape.
The numpy.reshape() function is useful when we need to change the dimensions of an array, for example, when we want to convert a one-dimensional array into a two-dimensional array or vice versa. It can also be used to create arrays with a specific shape, such as matrices and tensors.
Syntax:
numpy.reshape(a, newshape, order='C')
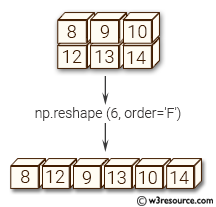
Parameter:
Name | Description | Required / Optional |
---|---|---|
a | Array to be reshaped. | Required |
newshape | The new shape should be compatible with the original shape. If an integer, then the result will be a 1-D array of that length. One shape dimension can be -1. In this case, the value is inferred from the length of the array and remaining dimensions. | Required |
order | Read the elements of a using this index order, and place the elements into the reshaped array using this index order. ‘C’ means to read / write the elements using C-like index order, with the last axis index changing fastest, back to the first axis index changing slowest. ‘F’ means to read / write the elements using Fortran-like index order, with the first index changing fastest, and the last index changing slowest. Note that the ‘C’ and ‘F’ options take no account of the memory layout of the underlying array, and only refer to the order of indexing. ‘A’ means to read / write the elements in Fortran-like index order if a is Fortran contiguous in memory, C-like order otherwise. | Optional |
Return value:
reshaped_array : ndarray - This will be a new view object if possible; otherwise, it will be a copy. Note there is no guarantee of the memory layout (C- or Fortran- contiguous) of the returned array.
Example: Reshaping a 2D NumPy array
>>> import numpy as np
>>> x = np.array([[2,3,4], [5,6,7]])
>> np.reshape(x, (3, 2))
array([[2, 3],
[4, 5],
[6, 7]])
The above code creates a 2D array x with two rows and three columns using the np.array() function. Then, the np.reshape() function is used to reshape the array x into a 3x2 array. The first argument of the np.reshape() function is the array to be reshaped, and the second argument is the desired shape of the new array. In this case, the desired shape is specified as (3, 2), which means that the resulting array will have 3 rows and 2 columns.
Pictorial Presentation:
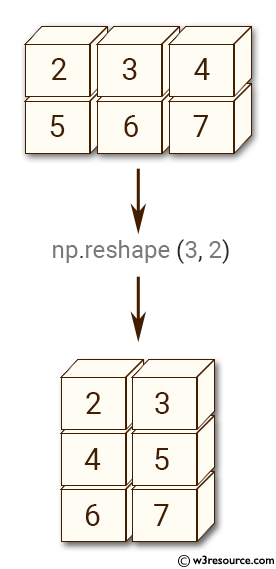
Example: Reshaping a NumPy array using a variable and an inferred dimension
>>> import numpy as np
>>> x = np.array([[2,3,4], [5,6,7]])
>>> np.reshape(x, (3, -1))
array([[2, 3],
[4, 5],
[6, 7]])
The above code shows how to reshape a NumPy array x with an unknown number of columns using the np.reshape() function. In this case, we want to reshape the array x into a new array with 3 rows and an unknown number of columns.
To do this, we pass the arguments (3, -1) to the np.reshape() function. The -1 argument indicates that we want NumPy to automatically determine the number of columns needed based on the total number of elements in the array.
The resulting reshaped array has 3 rows and 2 columns, which is the minimum number of columns required to accommodate all the elements in the original array x.
Pictorial Presentation:
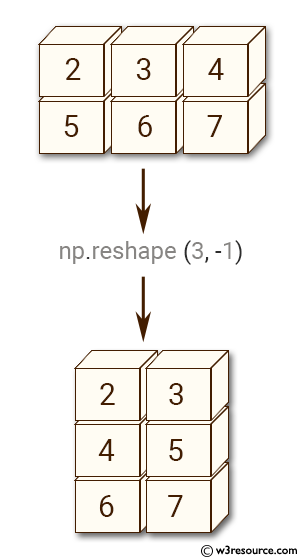
Example: Reshaping an Array to 1D using numpy.reshape() function
>>> import numpy as np
>>> x = np.array([[2,3,4], [5,6,7]])
>>> np.reshape(x, 6)
array([2, 3, 4, 5, 6, 7])
The above code shows how to use NumPy's reshape() function to convert a 2D array into a 1D array. This can be useful in various scenarios where a flattened array is required, such as in machine learning algorithms, data analysis, and numerical computations.
Pictorial Presentation:
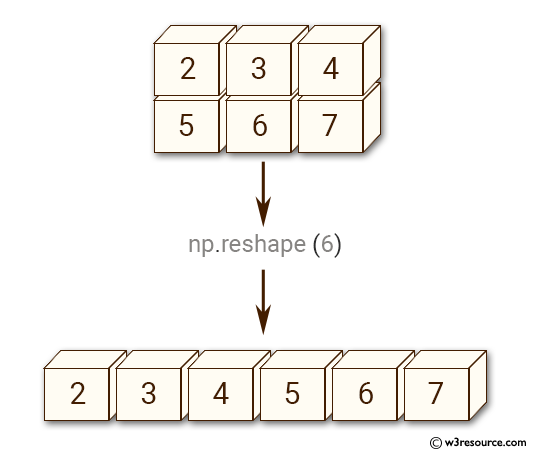
Example: Reshaping an array in column-major (Fortran) order using the order parameter in numpy.reshape() function
>>> import numpy as np
>>> x = np.array([[2,3,4], [5,6,7]])
>>> np.reshape(x, 6, order='F')
array([2, 5, 3, 6, 4, 7])
In the above code we use the np.reshape() function to reshape the array x into a one-dimensional array of size 6 with column-major (Fortran) order. The order parameter is used to specify the order in which elements should be arranged in the new reshaped array.
The resulting array is a flattened version of the original array x, with the elements arranged column-wise. The values in the new array are [2, 5, 3, 6, 4, 7].
Python - NumPy Code Editor: