NumPy: numpy.ndarray.flatten() function
numpy.ndarray.flatten() function
The numpy.ndarray.flatten() function is used to get a copy of an given array collapsed into one dimension.
This function is useful when we want to convert a multi-dimensional array into a one-dimensional array. By default, the array is flattened in row-major (C-style) order, but it can also be flattened in column-major (Fortran-style) order by specifying the 'F' parameter.
Syntax:
numpy.ndarray.flatten(order='C')
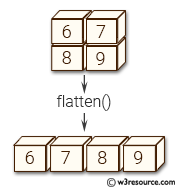
Parameters:
Name | Description | Required / Optional |
---|---|---|
order | ‘C’ means to flatten in row-major (C-style) order. ‘F’ means to flatten in column-major (Fortran- style) order. ‘A’ means to flatten in column-major order if a is Fortran contiguous in memory, row-major order otherwise. ‘K’ means to flatten a in the order the elements occur in memory. The default is ‘C’. | Optional |
Return value:
ndarray - A copy of the input array, flattened to one dimension.
Example: Flattening a NumPy array using numpy.ndarray.flatten()
>>> import numpy as np
>>> y = np.array([[2,3], [4,5]])
>>> y.flatten()
array([2, 3, 4, 5])
In the above code a 2D array y is defined with values [2, 3] and [4, 5]. The flatten() method is then called on y which returns a flattened 1D array. Therefore, the elements in the first row of y (2 and 3) come first in the flattened array, followed by the elements in the second row (4 and 5), resulting in a 1D array.
Pictorial Presentation:
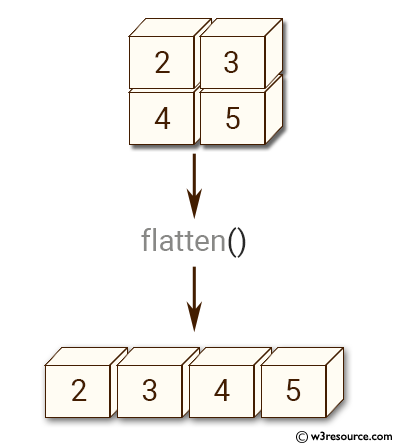
Example: Flattening a NumPy array column-wise using numpy.ndarray.flatten()
>>> import numpy as np
>>> y = np.array([[2,3], [4,5]])
>>> y.flatten('F')
array([2, 4, 3, 5])
In the above code a two-dimensional NumPy array 'y' is created with the array() function, containing the values [[2, 3], [4, 5]]. Then, the flatten() method is called on this array with the parameter 'F', which specifies column-wise flattening.
The resulting flattened array is [2, 4, 3, 5], which represents the original array 'y' flattened column-wise.
Pictorial Presentation:
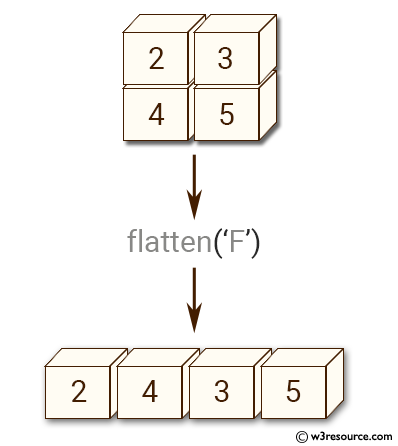
Python - NumPy Code Editor:
Previous: ndarray.flat()
Next: Transpose-like operations
moveaxis()