NumPy: numpy.append() function
numpy.append() function
The numpy.append() function is used to append values to the end of an existing array. It can append values to the flattened version of an array, or to a specified axis of a multi-dimensional array.
Key Features:
- Array Concatenation: It concatenates two or more arrays to form a larger array along the specified axis.
- Adding Rows or Columns: Users can add new rows or columns to an existing array, expanding its dimensions as needed.
- Flexible Usage: The function accepts both single elements and arrays as input for appending, offering versatility in array manipulation.
- Out-of-Place Operation: numpy.append() performs an out-of-place operation, meaning it creates and returns a new array with appended values without modifying the original array.
Here are some applications of numpy.append() function:
- Concatenate two or more arrays together to form a larger array.
- Add a new row or column to an existing array.
- Create a new array by appending an element to an existing array.
- Generate a sequence of numbers with a specific pattern by using numpy.append() in a loop.
Syntax:
numpy.append(arr, values, axis=None)
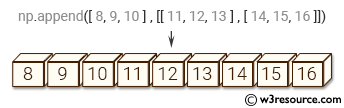
Parameters:
Name | Description | Required / Optional |
---|---|---|
arr | The array to which values will be appended. | Required |
values | The values or arrays to append to arr. Must be of compatible shape with arr along the specified axis. | Required |
axis | The axis along which values are appended. If not specified, both arr and values are flattened before use. | Optional |
Return value:
append: A copy of arr with values appended along the specified axis. Note that the operation does not modify arr; instead, it allocates a new array and fills it with the appended values.
Example: Appending arrays in NumPy using numpy.append()
>>> import numpy as np
>>> np.append ([0, 1, 2], [[3, 4, 5], [6, 7, 8]])
array([0, 1, 2, 3, 4, 5, 6, 7, 8])
In the above code, the np.append() function is used to append arrays in NumPy. The first argument passed to the function is a one-dimensional NumPy array and the second argument is a two-dimensional NumPy array.
Visual Presentation:
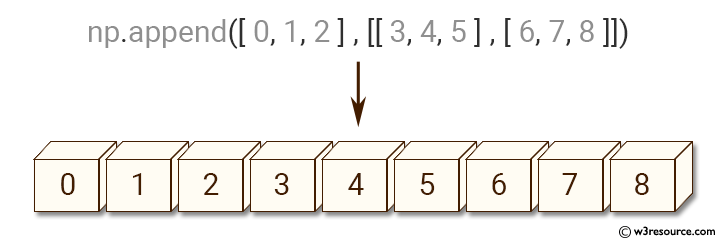
Example: Appending a 2D array to another 2D array along the vertical axis
>>> import numpy as np
>>> np.append([[0, 1, 2], [3, 4, 5]],[[6, 7, 8]], axis=0)
array([[0, 1, 2],
[3, 4, 5],
[6, 7, 8]])
In the above code, we have two 2D arrays with the shape (2,3) and (1,3) respectively. We use the np.append() function to append the second array vertically to the first array.
Visual Presentation:
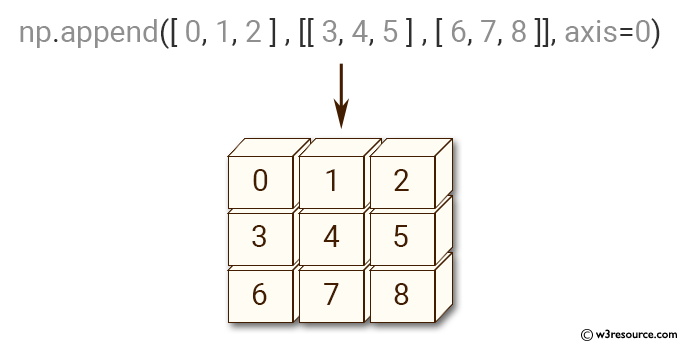
Frequently Asked Questions (FAQ): numpy.append() Function
1. What does the numpy.append() function do?
The numpy.append() function adds values to the end of an existing NumPy array. It can append values to a flattened version of an array or along a specified axis in multi-dimensional arrays.
2. Can numpy.append() handle multi-dimensional arrays?
Yes, numpy.append() can append values along a specified axis of a multi-dimensional array, provided the shapes are compatible.
3. Does numpy.append() modify the original array?
No, numpy.append() does not modify the original array. It creates and returns a new array with the appended values.
4. What types of values can be appended using numpy.append()?
numpy.append() can append single elements, lists, or other arrays to the original array. The appended values must be compatible with the shape of the original array along the specified axis.
5. Is numpy.append() efficient for large arrays?
Repeated use of numpy.append() can lead to performance issues due to the creation of new arrays each time. For large-scale operations, other methods like numpy.concatenate() or preallocating arrays might be more efficient.
6. How does numpy.append() handle the axis parameter?
If the axis parameter is not specified, both the input array and the values to append are flattened before the operation. If specified, the function appends values along the given axis.
7. What happens if the shapes of arrays are incompatible?
If the shapes of the arrays to be appended are not compatible along the specified axis, numpy.append() will raise a ValueError. It is important to ensure shape compatibility before appending.
8. Does numpy.append() maintain the data type of the original array?
Yes, the data type of the resulting array after using numpy.append() will be the same as the data type of the original array, unless the appended values introduce a different data type that can accommodate all values.
Python - NumPy Code Editor: