NumPy: numpy.ndarray.flat() function
numpy.ndarray.flat() function
The numpy.ndarray.flat() function is used to make 1-D iterator over the array. This is a numpy.flatiter instance, which acts similarly to, but is not a subclass of, Python’s built-in iterator object.
This function can be useful for iterating over all the elements of a multi-dimensional array without having to write nested loops. The flat attribute can be accessed directly from the array object.
Syntax:
ndarray.flat
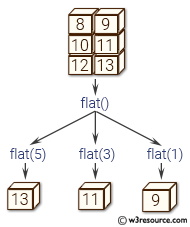
Example: Accessing array elements and iterating over them
>>> import numpy as np
>>> a = np.arange(2, 8).reshape(3, 2)
>>> a
array([[2, 3],
[4, 5],
[6, 7]])
>>> a.flat[4]
6
>>> a.T
array([[2, 4, 6],
[3, 5, 7]])
>>> a.T.flat[4]
5
>>> type(a.flat)
<class 'numpy.flatiter'>
In the above code, a NumPy array 'a' is created using the arange() and reshape() functions. 'a' is a 3x2 array of integers ranging from 2 to 7.
To access the 5th element of the array 'a', the .flat attribute is used. .flat returns a 1-dimensional iterator object that can be used to iterate over the elements of 'a' in a linear fashion. In this case, the 5th element (0-indexed) of the flattened a is 6.
The .T attribute is used to get the transpose of 'a', which is a 2x3 array. The 5th element of the flattened transpose is '5'.
The type of the .flat attribute is numpy.flatiter, which is an iterator that allows us to iterate over a flattened array.
Pictorial Presentation:
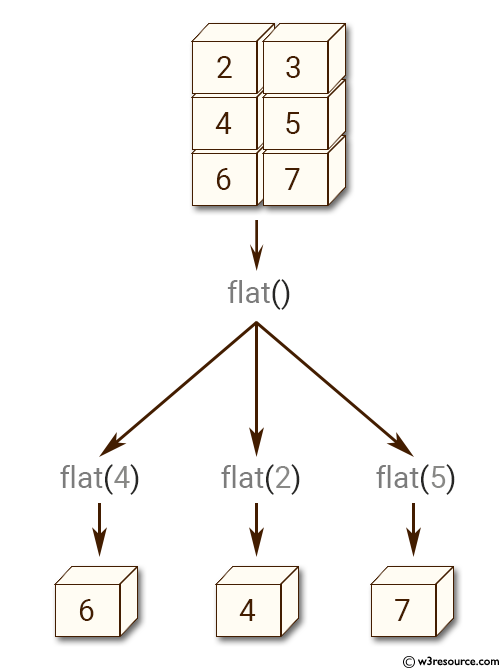
Example: Changing values of NumPy array using flat attribute
>>> import numpy as np
>>> a = np.arange(2, 8).reshape(3, 2)
>>> a
array([[2, 3],
[4, 5],
[6, 7]])
>>> a.flat = 4;a
array([[4, 4],
[4, 4],
[4, 4]])
>>> a.flat[[2,5]] = 2; a
array([[4, 4],
[2, 4],
[4, 2]])
In the above code, a 3x2 array is created using the arange() and reshape() functions. Then, the flat attribute is used to access the values of the array as a 1-dimensional array.
In the next line, all the values of the array are set to 4 using a single assignment statement. This is possible because the flat attribute provides a reference to the same memory locations as the original array. Therefore, changing the values of the flat attribute changes the values of the original array.
In the last line, the values at index 2 and 5 of the flat attribute are set to 2.
Python - NumPy Code Editor:
Previous: ravel()
Next: ndarray.flatten()