NumPy: numpy.concatenate() function
numpy.concatenate() function
The numpy.concatenate() is a function is used to join two or more arrays along a specified axis.
The numpy.concatenate() function can be used in various applications, such as merging two or more datasets with different variables or appending new data to an existing array. It is commonly used in data preprocessing and data analysis tasks in scientific computing, machine learning, and artificial intelligence.
Syntax:
numpy.concatenate((a1, a2, ...), axis=0, out=None)
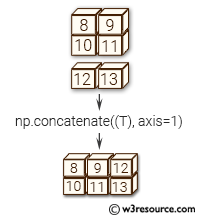
Parameters:
Name | Description | Required / Optional |
---|---|---|
a1,a2 | The arrays must have the same shape, except in the dimension corresponding to axis (the first, by default). | Required |
axis | The axis along which the arrays will be joined. If axis is None, arrays are flattened before use. Default is 0. | Optional |
out | If provided, the destination to place the result. The shape must be correct, matching that of what concatenate would have returned if no out argument were specified. | Optional |
Return value:
res : ndarray - The concatenated array.
Example: Concatenating arrays along a specified axis using NumPy
>>> import numpy as np
>>> x = np.array([[3, 4], [5, 6]])
>>> y = np.array([[7, 8]])
>>> np.concatenate((x,y), axis=0)
array([[3, 4],
[5, 6],
[7, 8]])
In the above code, we first import the NumPy library as 'np'. We then create two arrays 'x' and 'y' using the 'np.array()' function. The array 'x' contains two rows and two columns, whereas the array 'y' contains one row and two columns. We then use the NumPy function 'np.concatenate()' to concatenate the two arrays 'x' and 'y'.
The resulting array is a concatenation of the two input arrays along the specified axis. In this case, the resulting array has three rows and two columns, where the first two rows are from array 'x' and the third row is from array 'y'.
Pictorial Presentation:
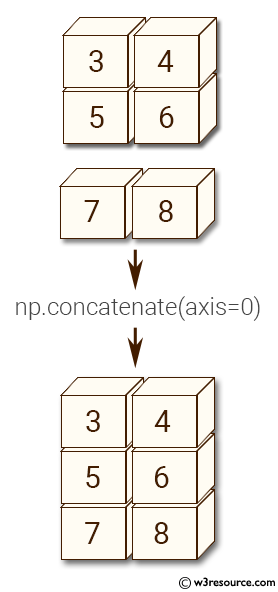
Example: Concatenating NumPy arrays along columns using numpy.concatenate()
>>> import numpy as np
>>> x = np.array([[3, 4], [5, 6]])
>>> y = np.array([[7, 8]])
>>> np.concatenate((x, y.T), axis=1)
array([[3, 4, 7],
[5, 6, 8]])
In the above code:
- First, a 2D NumPy array x is created with shape (2, 2) using the np.array() function.
- Then, another 2D NumPy array y is created with shape (1, 2) using the np.array() function.
- The np.concatenate() function is called with the input arrays x and y.T (transpose of y) and the axis parameter is set to 1 to concatenate along the columns.
- The resulting output is a new 2D NumPy array of shape (2, 3) where the two arrays are concatenated along the columns.
Pictorial Presentation:
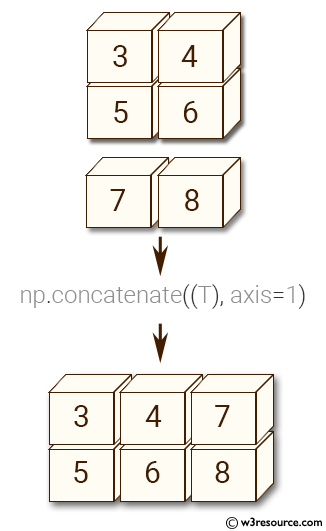
Example: Concatenating NumPy arrays along none axis
>>> import numpy as w3r
>>> x = w3r.array([[3, 4], [5, 6]])
>>> y = w3r.array([[7, 8]])
>>> w3r.concatenate((x, y), axis=None)
array([3, 4, 5, 6, 7, 8])
The above code defines two NumPy arrays, 'x' and 'y', and concatenates them along the none axis using the numpy.concatenate() function. The axis=None parameter concatenates the arrays as a single flat array by flattening each array first. The resulting array is one-dimensional and contains all the elements from both arrays in a single row.
Example: Combining masked and unmasked arrays using numpy.ma.concatenate()
>>> import numpy as w3r
>>> x = w3r.ma.arange(5)
>>> x[1] = w3r.ma.masked
>>> y = w3r.arange(3, 7)
>>> x
masked_array(data = [0 -- 2 3 4],
mask = [False True False False False],
fill_value = 999999)
>>> y
array([3, 4, 5, 6])
>>> w3r.concatenate([x, y])
masked_array(data = [0 1 2 3 4 3 4 5 6],
mask = False,
fill_value = 999999)
>>> w3r.ma.concatenate([x, y])
masked_array(data = [0 -- 2 3 4 3 4 5 6],
mask = [False True False False False False False False False],
fill_value = 999999)
In the above code, we have used the numpy.ma module to work with masked arrays. First, we create a masked array x using the ma.arange() function where the second element is masked using ma.masked. Then, we create a regular array y using numpy.arange().
Next, we concatenate the arrays using numpy.concatenate() and ma.concatenate(), and observe the differences in the results.
In numpy.concatenate([x, y]), the masked value in x is treated as a regular value, and is concatenated with the regular array y to produce a regular array. The resulting array has no mask, as it is not treated as a masked array.
In ma.concatenate([x, y]), the masked value in x is preserved in the resulting masked array. The resulting array has the same mask as x, but also includes the values from the regular array y.
Python - NumPy Code Editor:
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/numpy/manipulation/concatenate.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics