NumPy: numpy.delete() function
numpy.delete() function
The numpy.delete() function is used to remove one or more elements from an array along a specified axis. For a one dimensional array, this returns those entries not returned by arr[obj].
The numpy.delete() function can be used for various purposes such as removing duplicates,
Syntax:
numpy.delete(arr, obj, axis=None)
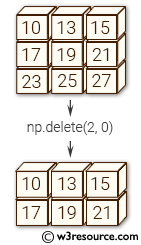
Parameters:
Name | Description | Required / Optional |
---|---|---|
arr | Input array. | Required |
obj | Indicate which sub-arrays to remove. | Required |
axis | The axis along which to delete the subarray defined by obj. If axis is None, obj is applied to the flattened array. | Optional |
Return value:
[ndarray] A copy of arr with the elements specified by obj removed. Note that delete does not occur in-place. If axis is None, out is a flattened array.
Example: Title: Deleting a row from a numpy array using numpy.delete()
>>> import numpy as np
>>> arr = np.array([[0,1,2], [4,5,6], [7,8,9]])
>>> arr
array([[0, 1, 2],
[4, 5, 6],
[7, 8, 9]])
>>> np.delete(arr, 1, 0)
array([[0, 1, 2],
[7, 8, 9]])
In the above code, a 2-dimensional numpy array 'arr' is created with 3 rows and 3 columns. Then, 'arr' is printed to the console to verify its contents.
Next, the np.delete() function is used to remove the second row (index 1) from arr by specifying 1 as the index to be deleted and 0 as the axis along which to delete (i.e., the row axis). The resulting array with the second row removed is returned and printed to the console.
Pictorial Presentation:
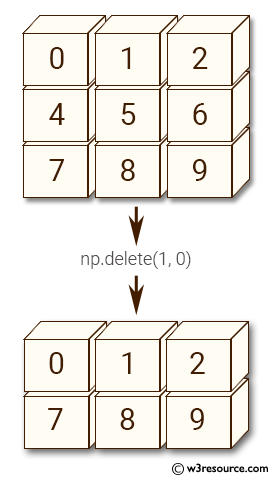
Example: Removing rows and columns using numpy.delete()
>>> import numpy as np
>>> np.delete(arr, np.s_[::2], 1)
array([[1],
[5],
[8]])
>>> np.delete(arr, [1, 2, 5], None)
array([0, 4, 5, 7, 8, 9])
In the first example, np.delete(arr, np.s_[::2], 1) removes every other column (starting from 0) from the arr array using the slice object np.s_[::2] as the second argument, and 1 as the third argument indicating that the operation is performed along the columns. The resulting array has only one column.
In the second example, np.delete(arr, [1, 2, 5], None) removes the elements with indices 1, 2, and 5 from the arr array along both rows and columns because the None argument is used for the second argument, which means the operation is performed on the flattened array. The resulting array is a one-dimensional array containing the remaining elements.
Pictorial Presentation:
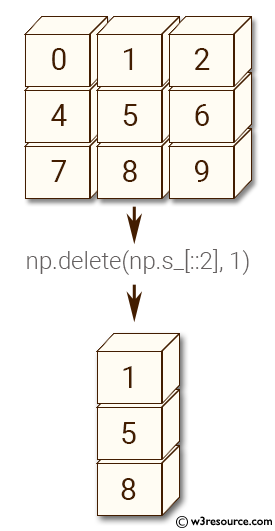
Python - NumPy Code Editor: