NumPy: numpy.vstack() function
numpy.vstack() function
numpy.vstack() function is used to stack arrays vertically (row-wise) to make a single array. It takes a sequence of arrays and joins them vertically.
This is equivalent to concatenation along the first axis after 1-D arrays of shape (N,) have been reshaped to (1,N).
This function is useful when you have two or more arrays with the same number of columns, and you want to concatenate them vertically (row-wise). It is also useful to append a single array as a new row to an existing 2D array.
Syntax:
numpy.vstack(tup)
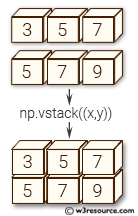
Parameters:
Name | Description | Required / Optional |
---|---|---|
tup | The sequence of arrays to be stacked. These arrays must have the same shape along all but the first axis. 1-D arrays must have the same length. | Required |
Return value:
stacked: ndarray - The array formed by stacking the given arrays.
Applications of numpy.vstack()
- Combining multiple 1-D arrays into a 2-D array.
- Merging multiple 2-D arrays row-wise.
- Appending rows to an existing 2-D array.
Example 1: Vertically Stacking 1-D Arrays
import numpy as np
x = np.array([3, 5, 7])
y = np.array([5, 7, 9])
result = np.vstack((x, y))
print(result)
Output:
array([[3, 5, 7], [5, 7, 9]])
In this example, two 1-D arrays x and y are stacked vertically to form a 2-D array with shape (2, 3).
Visual Presentation:
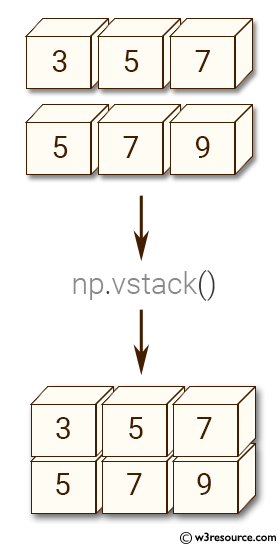
Example 2: Vertically Stacking 2-D Arrays
import numpy as np
x = np.array([[3], [5], [7]])
y = np.array([[5], [7], [9]])
result = np.vstack((x, y))
print(result)
Output:
array([[3], [5], [7], [5], [7], [9]])
Here, two 2-D arrays x and y, each with three rows and one column, are stacked vertically to form a 2-D array with six rows and one column.
Example 3: Combining Multiple Arrays
import numpy as np
a = np.array([[1, 2], [3, 4]])
b = np.array([[5, 6], [7, 8]])
c = np.array([[9, 10], [11, 12]])
result = np.vstack((a, b, c))
print(result)
Output:
array([[ 1, 2], [ 3, 4], [ 5, 6], [ 7, 8], [ 9, 10], [11, 12]])
In this example, three 2-D arrays a, b, and c are vertically stacked to form a single 2-D array with six rows and two columns.
Additional Considerations:
- Ensure that all arrays have the same shape along all but the first axis to avoid errors.
- When stacking 1-D arrays, they are first reshaped to (1, N) before being stacked.
Frequently Asked Questions (FAQ):
1. What is numpy.vstack() used for?
numpy.vstack() is used to vertically stack multiple arrays into a single array. It is commonly used to concatenate arrays row-wise.
2. When should I use numpy.vstack()?
Use numpy.vstack() when you need to combine two or more arrays with the same number of columns into a single array by stacking them vertically.
3. Can numpy.vstack() be used with 1-D arrays?
Yes, numpy.vstack() can be used with 1-D arrays. These arrays will be reshaped to 2-D arrays with a single row before being stacked.
4. What types of arrays can be combined using numpy.vstack()?
Any arrays that have the same shape along all but the first axis can be combined using numpy.vstack(). This includes combinations of 1-D and 2-D arrays, as long as the column counts match.
5. Does numpy.vstack() modify the original arrays?
No, numpy.vstack() does not modify the original arrays. It returns a new array with the specified arrays stacked vertically.
6. What happens if the arrays have different shapes along the concatenation axis?
If the arrays have different shapes along the first axis, numpy.vstack() will raise a ValueError. Ensure that all arrays have the same number of columns before stacking.
7. Is numpy.vstack() limited to only two arrays?
No, numpy.vstack() can be used to stack any number of arrays. Simply pass the arrays as a sequence (e.g., a list or tuple) to the function.
8. How does numpy.vstack() handle arrays with different dimensions?
When using numpy.vstack(), all arrays must have the same shape along all but the first axis. For 1-D arrays, they will be reshaped to 2-D with a single row before stacking. Arrays with incompatible shapes will cause an error.
Python - NumPy Code Editor: